Java で 1 から 10 までの乱数を生成する方法
-
1 から 10 までの乱数を生成するには
random.nextInt()
を使用する -
1 から 10 までの乱数を生成するための
Math.random()
の例 -
1 から 10 までの乱数を生成するために
ThreadLocalRandom.current.nextInt()
を使用する
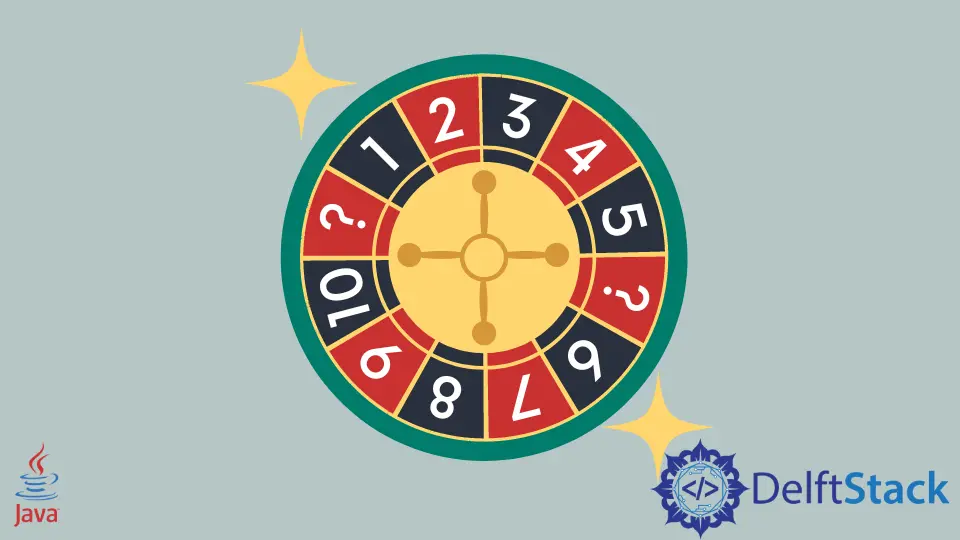
Java で 1 から 10 の間の乱数をランダムに生成する手順を見ていきます。1 から 10 の間の乱数を生成することができる 3つの Java パッケージやクラスを見て、どれを使用するのが最も適しているかを見ていきます。
1 から 10 までの乱数を生成するには random.nextInt()
を使用する
java.util.Random
は Java に付属しているパッケージであり、これを使って範囲を指定して乱数を生成することができます。ここでは、1 から 10 までの範囲を指定します。
このパッケージにはクラス Random
があり、これを使用すると、int
でも float
でも、複数のタイプの数値を生成できます。理解を深めるには、例を確認してください。
import java.util.Random;
public class Main {
public static void main(String[] args) {
int min = 1;
int max = 10;
Random random = new Random();
int value = random.nextInt(max + min) + min;
System.out.println(value);
}
}
出力:
6
上記の手法が動作し、毎回乱数を生成していることを示すために、ループを使って終了するまで新しい乱数を生成することができます。大きな範囲の数値を持っていないので、乱数が繰り返される可能性があります。
import java.util.Random;
public class Main {
public static void main(String[] args) {
Random random = new Random();
for (int i = 1; i <= 10; i++) {
int value = random.nextInt((10 - 1) + 1) + 1;
System.out.println(value);
}
}
出力:
10
7
2
9
2
7
6
4
9
1 から 10 までの乱数を生成するための Math.random()
の例
目標を達成するのに役立つもう一つのクラスは、数値をランダム化するための複数の静的関数を持つ Math
です。ここでは random()
メソッドを使用することにします。これは float
型の乱数値を返します。このため、これを int
にキャストしなければなりません。
public class Main {
public static void main(String[] args) {
int min = 1;
int max = 10;
for (int i = min; i <= max; i++) {
int getRandomValue = (int) (Math.random() * (max - min)) + min;
System.out.println(getRandomValue);
}
}
出力:
5
5
2
1
6
9
3
6
5
7
1 から 10 までの乱数を生成するために ThreadLocalRandom.current.nextInt()
を使用する
1 から 10 までの乱数を取得する最後のメソッドは、JDK 7 でマルチスレッドプログラム用に導入された ThreadLocalRandom
クラスを使用します。
現在のスレッドで乱数を生成したいので、クラスの current()
メソッドを呼び出す必要があることがわかります。
import java.util.concurrent.ThreadLocalRandom;
public class Main {
public static void main(String[] args) {
int min = 1;
int max = 10;
for (int i = 1; i <= 10; i++) {
int getRandomValue = ThreadLocalRandom.current().nextInt(min, max) + min;
System.out.println(getRandomValue);
}
}
}
出力:
3
4
5
8
6
2
6
10
6
2
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn