Java で数式を評価する
Zeeshan Afridi
2023年10月12日
Java
Java Math
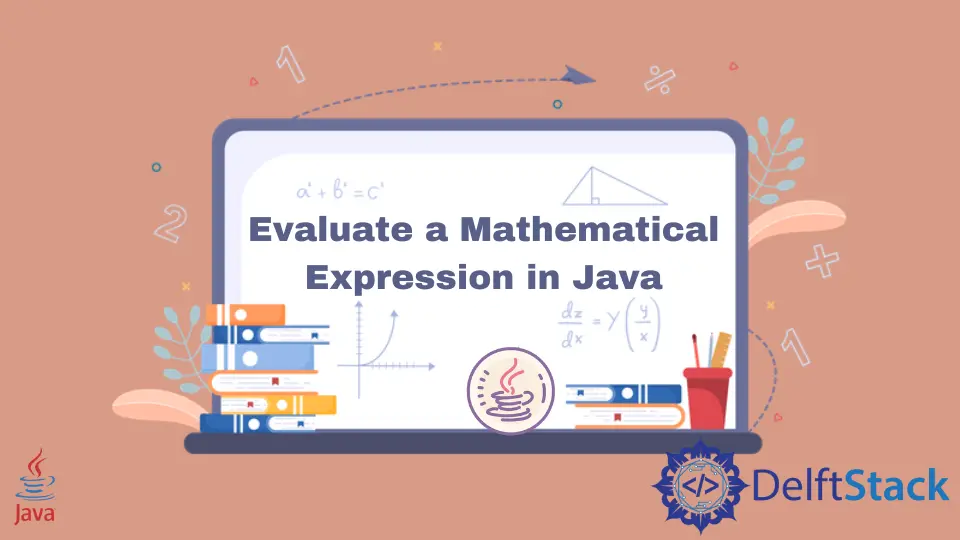
スタックを使用して数式を評価することは、最も一般的で便利なオプションの 1つです。
スタックには、pop()
と push()
の 2つの標準メソッドがあり、スタックからオペランドまたは演算子を配置および取得するために使用されます。pop()
メソッドは式の最上位要素を削除しますが、push()
メソッドは要素をスタックの最上位に配置します。
Java で数式を評価する
これは、数式を評価するための Java の例です。このコードは、除算、乗算、加算、および減算の優先順位を持つ適切な DMAS ルールに従います。
入力として任意の数式を指定できますが、式が次の 4つの演算(加算、乗算、除算、減算)のみで構成されていることを確認してください。
コード例:
package evaluateexpression;
import java.util.Scanner;
import java.util.Stack;
public class EvaluateExpression {
public static void main(String[] args) {
Scanner scan = new Scanner(System.in);
// Creating stacks for operators and operands
Stack<Integer> operator = new Stack();
Stack<Double> value = new Stack();
// Let's create some temparory stacks for operands and operators
Stack<Integer> tmpOp = new Stack();
Stack<Double> tmpVal = new Stack();
// Enter an arthematic expression
System.out.println("Enter expression");
String input = scan.next();
System.out.println(
"The type of the expression is " + ((Object) input).getClass().getSimpleName());
input = "0" + input;
input = input.replaceAll("-", "+-");
// In the respective stacks store the operators and operands
String temp = "";
for (int i = 0; i < input.length(); i++) {
char ch = input.charAt(i);
if (ch == '-')
temp = "-" + temp;
else if (ch != '+' && ch != '*' && ch != '/')
temp = temp + ch;
else {
value.push(Double.parseDouble(temp));
operator.push((int) ch);
temp = "";
}
}
value.push(Double.parseDouble(temp));
// Create a character array for the operator precedence
char operators[] = {'/', '*', '+'};
/* Evaluation of expression */
for (int i = 0; i < 3; i++) {
boolean it = false;
while (!operator.isEmpty()) {
int optr = operator.pop();
double v1 = value.pop();
double v2 = value.pop();
if (optr == operators[i]) {
// if operator matches evaluate and store it in the temporary stack
if (i == 0) {
tmpVal.push(v2 / v1);
it = true;
break;
} else if (i == 1) {
tmpVal.push(v2 * v1);
it = true;
break;
} else if (i == 2) {
tmpVal.push(v2 + v1);
it = true;
break;
}
} else {
tmpVal.push(v1);
value.push(v2);
tmpOp.push(optr);
}
}
// pop all the elements from temporary stacks to main stacks
while (!tmpVal.isEmpty()) value.push(tmpVal.pop());
while (!tmpOp.isEmpty()) operator.push(tmpOp.pop());
// Iterate again for the same operator
if (it)
i--;
}
System.out.println("\nResult = " + value.pop());
}
}
出力:
Enter expression
2+7*5-3/2
The type of the expression is String
Result = 35.5
上記のコードの出力からわかるように、式 2+7*5-3/2
が入力として与えられました。そして、プログラムは結果を 35.5
として計算しました。
DMAS ルールでは、分割の優先順位が最も高いため、最初に 3/2 = 1.5
を分割しました。次に、乗算部分は 7*5 = 35
として計算されます。
次に、2+35 = 37
を加算します。式の最後の部分は、37 -1.5 = 35.5
である減算です。
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe
著者: Zeeshan Afridi
Zeeshan is a detail oriented software engineer that helps companies and individuals make their lives and easier with software solutions.
LinkedIn