Java で文字列が空かヌルかを調べる方法
Hassan Saeed
2023年10月12日
Java
Java String
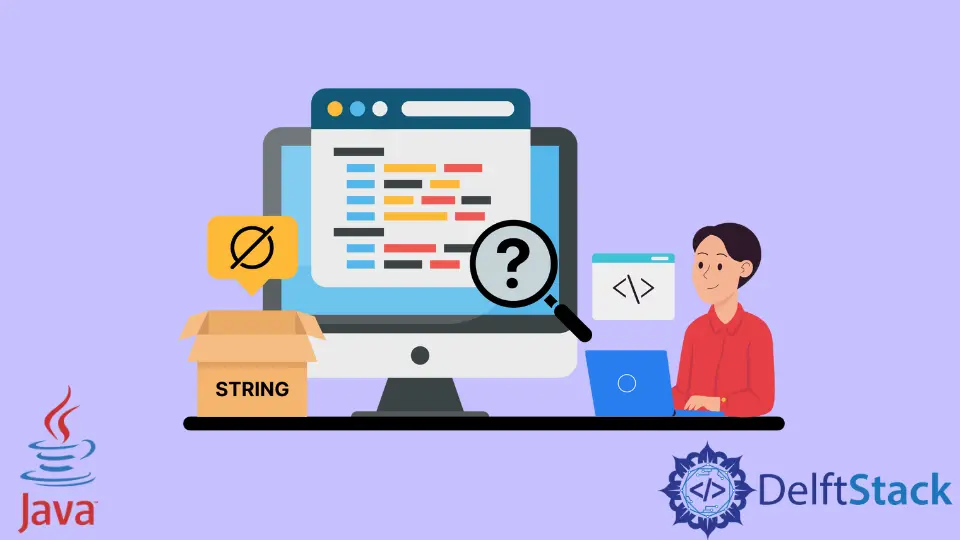
このチュートリアルでは、Java で文字列が空か null
かを調べるメソッドについて説明します。
Java で文字列が null
かどうかを調べるには str == null
を使用する
Java で与えられた文字列が null
であるかどうかを調べる最も簡単な方法は、str == null
を用いて null
と比較することです。以下の例はこれを示しています。
public class MyClass {
public static void main(String args[]) {
String str1 = null;
String str2 = "Some text";
if (str1 == null)
System.out.println("str1 is a null string");
else
System.out.println("str1 is not a null string");
if (str2 == null)
System.out.println("str2 is a null string");
else
System.out.println("str2 is not a null string");
}
}
出力:
str1 is a null string
str2 is not a null string
Java で str.isEmpty()
を使用して文字列が空かどうかを確認する
Java で与えられた文字列が空かどうかを調べる最も簡単な方法は、String
クラスの組み込みメソッド isEmpty()
を使用することです。以下の例はこれを示しています。
public class MyClass {
public static void main(String args[]) {
String str1 = "";
String str2 = "Some text";
if (str1.isEmpty())
System.out.println("str1 is an empty string");
else
System.out.println("str1 is not an empty string");
if (str2.isEmpty())
System.out.println("str2 is an empty string");
else
System.out.println("str2 is not an empty string");
}
}
出力:
str1 is an empty string
str2 is not an empty string
=両方の条件を同時にチェックしたい場合は、論理的な OR
演算子 - ||
を使用してチェックすることができます。以下の例はこれを示しています。
public class MyClass {
public static void main(String args[]) {
String str1 = "";
if (str1.isEmpty() || str1 == null)
System.out.println("This is an empty or null string");
else
System.out.println("This is neither empty nor null string");
}
}
出力:
This is an empty or null string
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe