java.io.IOException: Stream Closed エラーを修正します。
-
java.io.IOException: Stream closed
エラーの原因 -
新しいストリームを作成して、
java.io.IOException: Stream closed
エラーを修正する -
close()
をwriteToFile()
の外に移動して、java.io.IOException: Stream closed
エラーを修正する
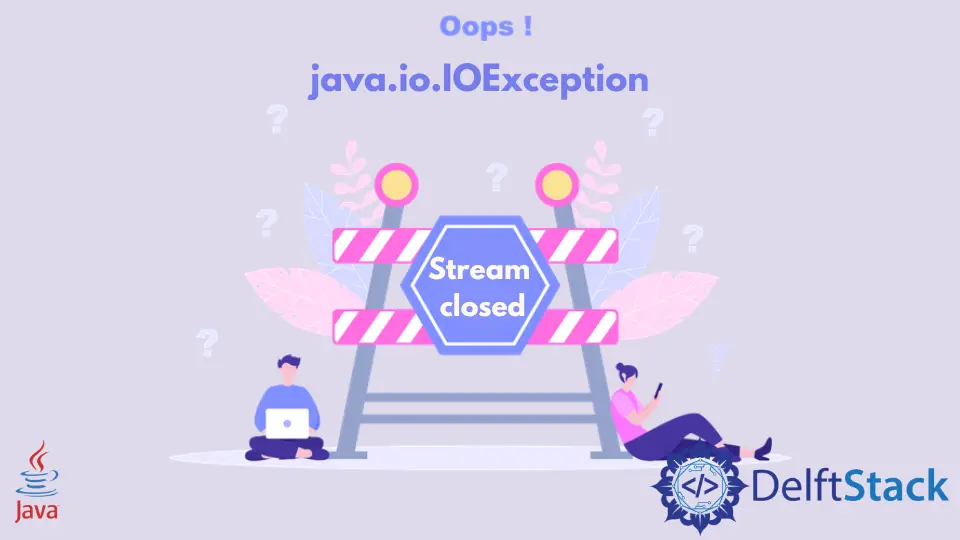
今日は、Java プログラミングでコーディング中に java.io.IOException: Stream closed
エラーを生成する考えられる原因を突き止めます。 また、コード例を使用して、このエラーを修正するための 2つの解決策を探ります。
java.io.IOException: Stream closed
エラーの原因
コード例 (エラーの原因):
// import libraries
import java.io.FileWriter;
import java.io.IOException;
// Test Class
public class Test {
// this method writes the given data into the specified file
// and closes the stream
static void writeToFile(
String greetings, String firstName, String lastName, FileWriter fileWriter) {
String customizedGreetings = greetings + "! " + firstName + " " + lastName;
try {
fileWriter.write(customizedGreetings + "\n");
fileWriter.flush();
fileWriter.close();
} catch (IOException exception) {
exception.printStackTrace();
}
} // end writeToFile() method
// main() method
public static void main(String[] args) throws IOException {
// creates a file in append mode and keeps it open
FileWriter fileWriter = new FileWriter("Files/file.txt", true);
// writeToFile() is called to write data into the file.txt
writeToFile("Hi", "Mehvish", "Ashiq", fileWriter);
writeToFile("Hello", "Tahir", "Raza", fileWriter);
} // end main()
} // end Test class
java.io.IOException: Stream closed
エラーの原因を見つけるためにコードを理解しましょう。 次に、その解決策にジャンプします。
このコード スニペットは、java.io
パッケージにある FileWriter
クラスを使用し、指定されたファイルに characters
形式でデータを書き込むために使用されます。 指定された場所にファイルが存在しない場合は、指定されたファイルを作成し、開いたままにします。
ファイルが既に存在する場合は、FileWriter
がそれを置き換えます。
main()
メソッド内で、FileWriter
コンストラクターを呼び出して指定されたファイルを append
モードで作成し、次に writeToFile()
メソッドを 2 回呼び出して、指定されたデータを file.txt
ファイルに書き込みます。 .
最初の呼び出しで、writeToFile()
メソッドはデータを file.txt
に書き込み、FileWriter
のデータをフラッシュして閉じます。 close()
メソッドを呼び出してストリームを閉じたことに注意してください。
2 回目の呼び出しでは、ストリームが閉じられているため、FileWriter
オブジェクトは書き込み先のファイルを見つけることができません。 そのため、writeToFile()
メソッドへの 2 回目の呼び出しがこのエラーを引き起こしています。
このエラーを修正するには、2つの解決策があります。 どちらもコード サンプルと共に以下に示します。
新しいストリームを作成して、java.io.IOException: Stream closed
エラーを修正する
最初の解決策は、FileWriter
オブジェクトを writeToFile()
関数に移動して、指定したファイルに書き込みたいときはいつでも新しいストリームを作成することです。
コード例:
// import libraries
import java.io.FileWriter;
import java.io.IOException;
// Test class
public class Test {
// this method writes the given data into the specified file
// and closes the stream
static void writeToFile(String greetings, String firstName, String lastName) throws IOException {
FileWriter fileWriter = new FileWriter("Files/file.txt", true);
String customizedGreetings = greetings + "! " + firstName + " " + lastName;
fileWriter.write(customizedGreetings + "\n");
fileWriter.flush();
fileWriter.close();
} // end writeToFile()
// main()
public static void main(String[] args) {
// writeToFile() is called to write data into the file
try {
writeToFile("Hi", "Mehvish", "Ashiq");
writeToFile("Hello", "Tahir", "Raza");
} catch (IOException e) {
e.printStackTrace();
}
} // end main()
} // end Test class
出力 (file.txt
内のデータ):
Hi! Mehvish Ashiq
Hello! Tahir Raza
close()
を writeToFile()
の外に移動して、java.io.IOException: Stream closed
エラーを修正する
2 番目の解決策は、close()
メソッドを writeToFile()
関数の外に移動することです。これは、解決策 1 と比較して適切なアプローチのようです。
コード例:
// import libraries
import java.io.FileWriter;
import java.io.IOException;
// Test Class
public class Test {
// this method writes the given data into the specified file
static void writeToFile(
String greetings, String firstName, String lastName, FileWriter fileWriter) {
String customizedGreetings = greetings + "! " + firstName + " " + lastName;
try {
fileWriter.write(customizedGreetings + "\n");
fileWriter.flush();
} catch (IOException exception) {
exception.printStackTrace();
}
} // end writeToFile()
// closes the stream
static void cleanUp(FileWriter fileWriter) throws IOException {
fileWriter.close();
} // end cleanUp()
// main()
public static void main(String[] args) throws IOException {
// create the file in the append mode and keep it open
FileWriter fileWriter = new FileWriter("Files/file.txt", true);
// writeToFile() is called to write data into the file.txt
writeToFile("Hi", "Mehvish", "Ashiq", fileWriter);
writeToFile("Hello", "Tahir", "Raza", fileWriter);
// close the stream
cleanUp(fileWriter);
} // end main()
} // end Test class
出力 (file.txt
内のデータ):
Hi! Mehvish Ashiq
Hello! Tahir Raza