継承中の Java コンストラクターの実行
- 継承中の Java コンストラクターの実行
- 継承におけるデフォルトの Java コンストラクターの実行
- 親クラスにデフォルトのパラメーター化されたコンストラクターがある場合の継承での Java コンストラクターの実行
-
super
を使用して、親クラスとすべての子クラスのパラメーター化されたコンストラクターを呼び出する
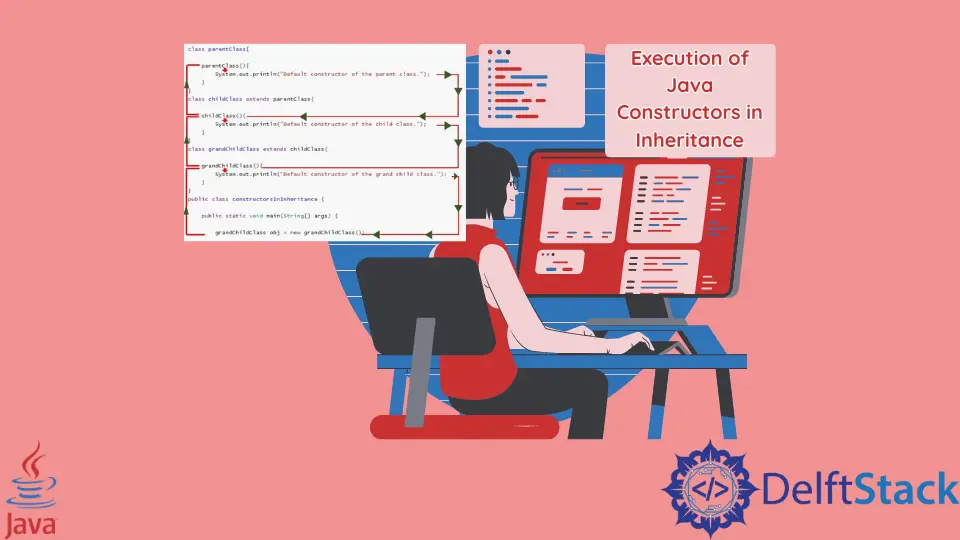
今日は、継承における Java コンストラクターの実行について学習します。派生クラス(子クラスおよびサブクラスとも呼ばれます)のデフォルトおよびパラメーター化されたコンストラクターのコード例を示します。
継承中の Java コンストラクターの実行
この記事を続けるには、継承に関する十分な知識が必要です。このチュートリアルを読んでいる場合は、Java の継承について十分に理解していることを前提としています。
extends
キーワードを使用して親クラス(基本クラスまたはスーパークラスとも呼ばれます)を拡張している間の Java コンストラクターの実行プロセスについて学びましょう。
継承におけるデフォルトの Java コンストラクターの実行
コード例:
class parentClass {
parentClass() {
System.out.println("Default constructor of the parent class.");
}
}
class childClass extends parentClass {
childClass() {
System.out.println("Default constructor of the child class.");
}
}
class grandChildClass extends childClass {
grandChildClass() {
System.out.println("Default constructor of the grand child class.");
}
}
public class constructorsInInheritance {
public static void main(String[] args) {
grandChildClass obj = new grandChildClass();
}
}
出力:
Default constructor of the parent class.
Default constructor of the child class.
Default constructor of the grand child class.
ここでは、grandChildClass
のオブジェクトを作成する constructorsInInheritance
という名前のテストクラスが 1つあります。
parentClass
、childClass
、grandChildClass
という名前の他の 3つのクラスがあり、grandChildClass
は childClass
を継承し、childClass
は parentClass
を拡張します。
ここで、parentClass
デフォルトコンストラクターは、childClass
コンストラクターによって自動的に呼び出されます。子クラスをインスタンス化するたびに、親クラスのコンストラクターが自動的に実行され、その後に子クラスのコンストラクターが続きます。
上記の出力を確認してください。それでも混乱する場合は、次の視覚的な説明を参照してください。
main
メソッドで childClass
のオブジェクトを作成するとどうなりますか?デフォルトのコンストラクターはどのように実行されますか?
parentClass
のコンストラクターが最初に実行され、次に childClass
のコンストラクターが次の結果を生成します。
出力:
Default constructor of the parent class.
Default constructor of the child class.
親クラスにデフォルトのパラメーター化されたコンストラクターがある場合の継承での Java コンストラクターの実行
コード例:
class parentClass {
parentClass() {
System.out.println("Default constructor of the parent class.");
}
parentClass(String name) {
System.out.println("Hi " + name + "! It's a parameterized constructor of the parent class");
}
}
class childClass extends parentClass {
childClass() {
System.out.println("Default constructor of the child class.");
}
}
class grandChildClass extends childClass {
grandChildClass() {
System.out.println("Default constructor of the grand child class.");
}
}
public class constructorsInInheritance {
public static void main(String[] args) {
grandChildClass obj = new grandChildClass();
}
}
出力:
Default constructor of the parent class.
Default constructor of the child class.
Default constructor of the grand child class.
ここでは、parentClass
にパラメーター化されたコンストラクターがあります。ただし、main
メソッドで grandChildClass()
デフォルトコンストラクターを呼び出し、さらに親クラスのデフォルトコンストラクターを呼び出すため、デフォルトコンストラクターは引き続き呼び出されます。
また、パラメータ化されたコンストラクタを childClass
と grandChildClass
に記述しましょう。次に、main
関数で grandChildClass
のパラメーター化されたコンストラクターを呼び出します。
デフォルトであるかパラメータ化されているかに関係なく、呼び出されるコンストラクタを観察します。
コード例:
class parentClass {
parentClass() {
System.out.println("Default constructor of the parent class.");
}
parentClass(String name) {
System.out.println("Hi " + name + "! It's a parameterized constructor of the parent class");
}
}
class childClass extends parentClass {
childClass() {
System.out.println("Default constructor of the child class.");
}
childClass(String name) {
System.out.println("Hi " + name + "! It's a parameterized constructor of the child class");
}
}
class grandChildClass extends childClass {
grandChildClass() {
System.out.println("Default constructor of the grand child class.");
}
grandChildClass(String name) {
System.out.println(
"Hi " + name + "! It's a parameterized constructor of the grand child class");
}
}
public class constructorsInInheritance {
public static void main(String[] args) {
grandChildClass obj = new grandChildClass("Mehvish");
}
}
出力:
Default constructor of the parent class.
Default constructor of the child class.
Hi Mehvish! It's a parameterized constructor of the grand child class
上記のコードは、grandChildClass
のパラメーター化されたコンストラクターのみを呼び出します。super()
を使用して、parentClass
、childClass
、および grandChildClass
のパラメーター化されたコンストラクターを呼び出します。
親クラスのコンストラクター呼び出しは、子クラスのコンストラクターの最初の行にある必要があることに注意してください。
super
を使用して、親クラスとすべての子クラスのパラメーター化されたコンストラクターを呼び出する
コード例:
class parentClass {
parentClass() {
System.out.println("Default constructor of the parent class.");
}
parentClass(String name) {
System.out.println("Hi " + name + "! It's a parameterized constructor of the parent class");
}
}
class childClass extends parentClass {
childClass() {
System.out.println("Default constructor of the child class.");
}
childClass(String name) {
super(name);
System.out.println("Hi " + name + "! It's a parameterized constructor of the child class");
}
}
class grandChildClass extends childClass {
grandChildClass() {
System.out.println("Default constructor of the grand child class.");
}
grandChildClass(String name) {
super(name);
System.out.println(
"Hi " + name + "! It's a parameterized constructor of the grand child class");
}
}
public class constructorsInInheritance {
public static void main(String[] args) {
grandChildClass obj = new grandChildClass("Mehvish");
}
}
出力:
Hi Mehvish! It's a parameterized constructor of the parent class
Hi Mehvish! It's a parameterized constructor of the child class
Hi Mehvish! It's a parameterized constructor of the grand child class
super
キーワードを使用して、パラメーター化された親クラスコンストラクターを呼び出しました。親クラス(スーパークラスまたはベースクラス)を指します。
これを使用して親クラスのコンストラクターにアクセスし、親クラスのメソッドを呼び出しました。
super
は、親クラスと子クラスの正確な名前を持つメソッドに非常に役立ちます。