Go でオブジェクトのタイプを見つける方法
Suraj Joshi
2024年2月16日
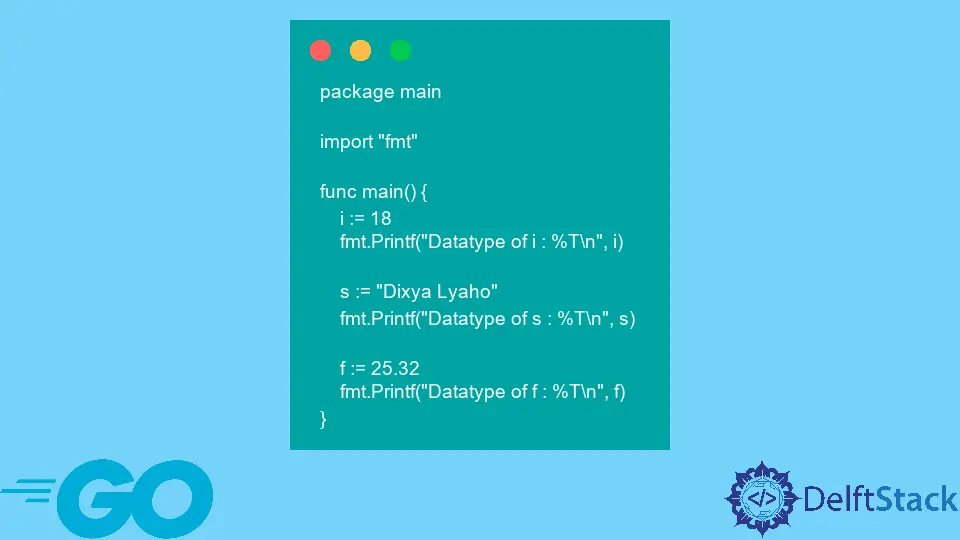
データ型は、有効な Go 変数に関連付けられた型を指定します。Go のデータ型には、次の 4つのカテゴリがあります。
-
基本タイプ:数値、文字列、ブール値
-
集計タイプ:配列と構造体
-
参照タイプ:ポインター、スライス、マップ、関数、およびチャネル
-
インターフェースタイプ
Go でオブジェクトのデータ型を見つけるには、文字列フォーマット
、リフレクト
パッケージ、タイプアサーション
を使用します。
Go でデータ型を検索するための文字列フォーマット
パッケージ fmt
の Printf
関数を特別なフォーマットオプションで使用できます。fmt
を使用して変数を表示します。ために使用できるフォーマットオプションは次のとおりです。
フォーマット | 説明 |
---|---|
%v |
変数値をデフォルト形式で出力します |
%+v |
値を持つフィールド名を追加する |
%#v |
値の Go 構文表現 |
%T |
値のタイプの Go 構文表現 |
%% |
文字通りのパーセント記号。価値を消費しない |
Go でオブジェクトのタイプを見つけるために、fmt
パッケージの%T
フラグを使用します。
package main
import "fmt"
func main() {
i := 18
fmt.Printf("Datatype of i : %T\n", i)
s := "Dixya Lyaho"
fmt.Printf("Datatype of s : %T\n", s)
f := 25.32
fmt.Printf("Datatype of f : %T\n", f)
}
出力:
Datatype of i : int
Datatype of s : string
Datatype of f : float64
reflect
パッケージ
また、reflect
パッケージを使用してオブジェクトのデータ型を見つけることもできます。reflect
パッケージの Typeof
関数は、.String()
で string
に変換できるデータ型を返します。
package main
import (
"fmt"
"reflect"
)
func main() {
o1 := "string"
o2 := 10
o3 := 1.2
o4 := true
o5 := []string{"foo", "bar", "baz"}
o6 := map[string]int{"apple": 23, "tomato": 13}
fmt.Println(reflect.TypeOf(o1).String())
fmt.Println(reflect.TypeOf(o2).String())
fmt.Println(reflect.TypeOf(o3).String())
fmt.Println(reflect.TypeOf(o4).String())
fmt.Println(reflect.TypeOf(o5).String())
fmt.Println(reflect.TypeOf(o6).String())
}
出力:
string
int
float64
bool
[]string
map[string]int
type assertions
メソッド
型アサーションメソッドは、アサーション操作が成功したかどうかを示すブール変数を返します。タイプ switch
を使用して、いくつかのタイプアサーションを連続して実行し、オブジェクトのデータタイプを検索します。
package main
import (
"fmt"
)
func main() {
var x interface{} = 3.85
switch x.(type) {
case int:
fmt.Println("x is of type int")
case float64:
fmt.Println("x is of type float64")
default:
fmt.Println("x is niether int nor float")
}
}
出力:
x is of type float64
著者: Suraj Joshi
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn