CSS でボタンを中央に配置する
- ボタンを垂直および水平方向に中央揃え
- ボタンを中央に配置するさまざまな方法
-
text-align: center
を使用してボタンを中央に配置 -
display: grid
とmargin: auto
を使用してボタンを中央に配置する -
display: flex
を使用してボタンを中央に配置する -
position: fixed
を使用してボタンを中央に配置する - 2つ以上のボタンを中央に配置
- まとめ
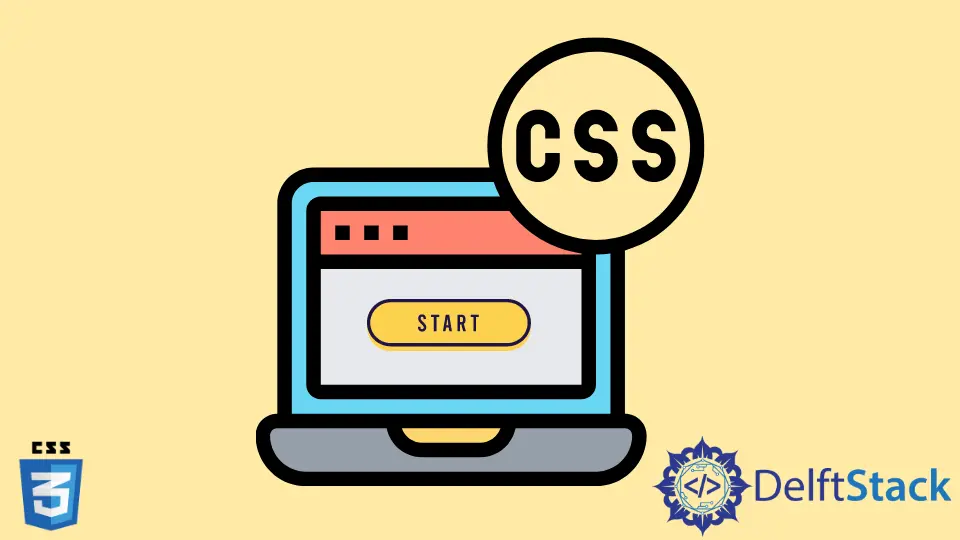
HTML Web ページの最も繊細なスタイル設定は、主に CSS によって実現されます。 Web ページ上のコンポーネントの配置は CSS で制御できます。
Web ページの中央にボタンを配置するには、さまざまな方法があります。 ボタンの配置は、好みに応じて水平または垂直に取り付けることができます。
ボタンを垂直および水平方向に中央揃え
この例では、ポジショニングと transform
プロパティを使用して、button
要素を垂直方向および水平方向に中央揃えにしています。
<!DOCTYPE html>
<html>
<head>
<style>
.container {
height: 200px;
position: relative;
border: 2px solid blue;
}
.center {
margin: 0;
position: absolute;
top: 50%;
left: 50%;
-ms-transform: translate(-50%, -50%);
transform: translate(-50%, -50%);
}
</style>
</head>
<body>
<div class="container">
<div class="center">
<button>Button at the Center</button>
</div>
</div>
</body>
</html>
ここでは、コンテナ内のボタンのビューを設定して、中央揃えを明確に表示しています。
ボタンを中央に配置するさまざまな方法
次の方法を使用して、ボタンを中央に配置できます。
text-align: center
- 親div
タグのtext-align
プロパティの値をcenter
として入力します。margin: auto
-margin
プロパティの値をauto
に入力します。display: flex
-display
プロパティの値をflex
に入力し、justify-content
プロパティの値をcenter
に設定します。display: grid
-display
プロパティの値をgrid
として入力します。
ボタンを中央に配置するために使用できる方法は他にもあります。
text-align: center
を使用してボタンを中央に配置
次の例では、text-align
プロパティを使用し、その値を center
に設定します。 これは、body
タグまたは要素の親 div
タグ内に配置できます。
button
要素の親 div
タグに text-align: center
を配置します。
<!DOCTYPE html>
<html>
<head>
<style>
.container{
text-align: center;
border: 2px solid blue;
width: 300px;
height: 200px;
padding-top: 100px;
}
#bttn{
font-size: 18px;
}
</style>
</head>
<body>
<div class="container">
<button id ="bttn"> Button at the Center </button>
</div>
</body>
</html>
body
タグ内に text-align: center
を配置する:
<!DOCTYPE html>
<html>
<head>
<style>
body {
text-align: center;
}
</style>
</head>
<body>
<button>Button at the Center</button>
</body>
</html>
必要に応じて、ボタンをページの正確な中央に移動します。padding-top
プロパティを使用する必要がありますが、これはボタンを中央に配置するための最良の方法ではありません。
display: grid
と margin: auto
を使用してボタンを中央に配置する
ここでは、CSS で display: grid
プロパティと margin: auto
プロパティを使用します。 次の例では、display: grid
は button
要素の親 div
タグに配置されています。
<!DOCTYPE html>
<html>
<head>
<style>
.container {
width: 300px;
height: 300px;
border: 2px solid blue;
display: grid;
}
button {
background-color: lightpink;
color: black;
font-size: 18px;
margin: auto;
}
</style>
</head>
<body>
<div class="container">
<button>Button at the Center</button>
</div>
</body>
</html>
ここでは、ボタンを縦位置と横位置の中央に配置します。
grid
値を持つ display
プロパティがコンテナー内で使用されます。 したがって、ボタンに影響する button
要素で auto
として margin
プロパティが使用されている間、コンテナに影響します。
これら 2つのプロパティは、ボタンを中央に配置する際に重要な役割を果たします。
display: flex
を使用してボタンを中央に配置する
これは、ボタンを中央に揃える場合に最もよく使用される方法です。 display: flex;
、justify-content: center;
、align-items: center;
の 3つのプロパティは、ボタンを中央に配置する際に重要な役割を果たします。
この例は、垂直位置と水平位置の中央でのボタンの配置を示しています。
<!DOCTYPE html>
<html>
<head>
<style>
.container {
width: 300px;
height: 300px;
border: 2px solid blue;
display: flex;
justify-content: center;
align-items: center;
}
button {
background-color: lightpink;
color: black;
font-size: 18px;
}
</style>
</head>
<body>
<div class="container">
<button>Button at the Center</button>
</div>
</body>
</html>
position: fixed
を使用してボタンを中央に配置する
次の例では、ページの左側から 50% のマージンを与えてから、ボディの中央の位置だけを取る position: fixed
を設定しています。
<!DOCTYPE html>
<html>
<head>
<style>
button {
position: fixed;
left: 50%;
}
</style>
</head>
<body>
<div class="button-container-div">
<button>Button at the Center</button>
</div>
</body>
</html>
ここでは、ボタンは Web ページ全体の中央には適用されません。
2つ以上のボタンを中央に配置
ボタンが 2つ以上ある場合は、それらすべてのボタンを 1つの div
要素でラップし、flexbox プロパティを使用してボタンを中央に配置できます。 2つのボタンが 1つの div 要素と見なされる場合、すべてのプロパティが両方に適用されます。
例えば:
<!DOCTYPE html>
<html>
<head>
<style>
.flex-box {
display: flex;
}
.jc-center {
justify-content: center;
}
button.margin-right {
margin-right: 20px;
}
</style>
</head>
<body>
<div class="flex-box jc-center">
<button type="submit" class="margin-right">Select</button>
<button type="submit">Submit</button>
</div>
</body>
</html>
または、次の例のように、フレックスボックスを使用してインライン ブロック要素とブロック要素の両方を中央に配置することもできます。
<!DOCTYPE html>
<html>
<head>
<style>
.flex-box {
display: flex;
}
.jc-center {
justify-content: center;
}
</style>
</head>
<body>
<div class="flex-box jc-center">
<button type="submit">Select</button>
</div>
<br>
<div class="flex-box jc-center">
<button type="submit">Submit</button>
</div>
</body>
</html>
まとめ
CSS と HTML のさまざまなプロパティを使用して、ボタンを中央に配置する方法は多数あります。 前述のように、CSS のさまざまなプロパティを HTML と組み合わせて使用することで、Web ページの中央にボタンを簡単に配置するためのさまざまなアプローチを使用できます。
Nimesha is a Full-stack Software Engineer for more than five years, he loves technology, as technology has the power to solve our many problems within just a minute. He have been contributing to various projects over the last 5+ years and working with almost all the so-called 03 tiers(DB, M-Tier, and Client). Recently, he has started working with DevOps technologies such as Azure administration, Kubernetes, Terraform automation, and Bash scripting as well.