C# で文字列を型に変換する
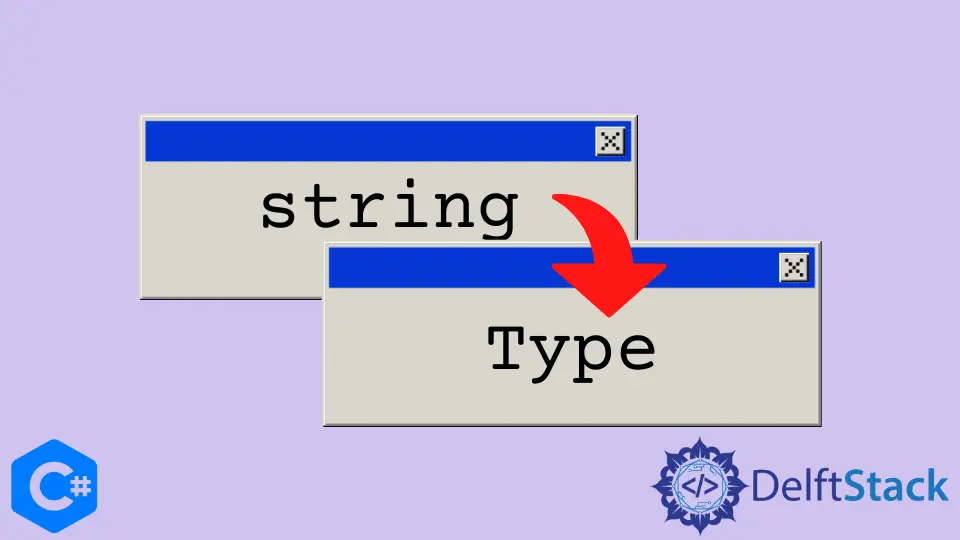
この記事では、文字列を type
に変換する方法、または C# でデータ型を取得する方法について説明します。値の型を決定するために、.GetType
関数を利用します。
C#
で文字列値の type
を取得する
以下の例では、文字列のランタイム
タイプとその他の値を取得し、各値のタイプの取得に進みます。
StringtoType
という名前のクラスと Main()
メソッドを作成します。
class StringtoType {
public static void Main() {}
}
次に、allvalues
と呼ばれる Object[]
型変数を作成し、"Abc"
(文字列として)や 89
(バイトとして)などの値を指定します。
object[] allvalues = { "Abc",
(long)193527,
"Happy Programming",
(byte)89,
'Z',
(sbyte)-11,
"Zeelandia is 8th continent",
27.9,
"I am a string line",
(int)20,
'7' };
次に、foreach
ループを使用して、配列内の各エントリを検証し、そのタイプを判別します。
foreach (var data in allvalues) {
}
foreach
ループ内のタイプの Type
の t
という名前の変数を初期化します。変数 t
は、data.GetType()
メソッドを使用して、配列 allvalues
に存在するすべての値のデータ型を保持します。
Type t = data.GetType();
その後、if
条件を適用して、すべての値が文字列になるかどうかを確認します。
if (t.Equals(typeof(string)))
値が文字列であることが判明した場合、以下のようなメッセージが表示されます。
'Happy Programming' is a String
文字列を Type
に変換した後、else if
チェックに従って、byte
、sbyte
、int
、double
などの他のタイプのデータを入力します。
else if (t.Equals(typeof(sbyte))) Console.WriteLine(" '{0}' is a Signed Byte", data);
else if (t.Equals(typeof(byte))) Console.WriteLine(" '{0}' is a Byte", data);
else if (t.Equals(typeof(int))) Console.WriteLine(" '{0}' is an Integer of 32-bit", data);
else if (t.Equals(typeof(long))) Console.WriteLine(" '{0}' is an Integer of 64-bit", data);
else if (t.Equals(typeof(double))) Console.WriteLine("'{0}' is a double ", data);
最後に、配列 allvalues
に byte
、sbyte
、int
、double
、long
タイプ以外のデータが見つかった場合は、次のようなメッセージが表示されます。
'Z' is another type of data
完全なコード例:
using System;
using System.Diagnostics;
class StringtoType {
public static void Main() {
object[] allvalues = { "Abc",
(long)193527,
"Happy Programming",
(byte)89,
'Z',
(sbyte)-11,
"Zeelandia is 8th continent",
27.9,
"I am a string line",
(int)20,
'7' };
foreach (var data in allvalues) {
Type t = data.GetType();
if (t.Equals(typeof(string)))
Console.WriteLine(" '{0}' is a String", data);
else if (t.Equals(typeof(sbyte)))
Console.WriteLine(" '{0}' is a Signed Byte", data);
else if (t.Equals(typeof(byte)))
Console.WriteLine(" '{0}' is a Byte", data);
else if (t.Equals(typeof(int)))
Console.WriteLine(" '{0}' is an Integer of 32-bit", data);
else if (t.Equals(typeof(long)))
Console.WriteLine(" '{0}' is an Integer of 64-bit", data);
else if (t.Equals(typeof(double)))
Console.WriteLine("'{0}' is a double", data);
else
Console.WriteLine("'{0}' is another type of data", data);
}
}
}
出力:
'Abc' is a String
'193527' is an Integer of 64-bit
'Happy Programming' is a String
'89' is a Byte
'Z' is another type of data
'-11' is a Signed Byte
'Zeelandia is 8th continent' is a String
'27.9' is a double
'I am a string line' is a String
'20' is an Integer of 32-bit
'7' is another type of data
C#
の type
オブジェクトを比較する
タイプを持つ Type
オブジェクトは区別されます。2つの Type
オブジェクト識別子は、同じタイプを表す場合、同じオブジェクトに対応します。
これにより、参照の同等性を使用して Type
オブジェクトを比較できます。次の例では、複数の整数値を含む Type
オブジェクトを比較して、それらが同じタイプであるかどうかを確認します。
最初に、Main()
メソッド内で int
、double
、および long
タイプの変数を初期化し、それらにいくつかの値を割り当てます。
int Value1 = 2723;
double Value2 = 123.56;
int Value3 = 1747;
long Value4 = 123456789;
タイプ Type
の t
という名前の変数を変数 t
に初期化します。.GetType
メソッドを使用して、Value1
という名前の値 1 のデータ型を格納します。
Type t = Value1.GetType();
ここで、Object.ReferenceEquals(t, Value2.GetType())
関数を使用して、すべてのオブジェクトのタイプを Value1
と比較します。
Console.WriteLine("Data type of Value1 and Value2 are equal: {0}",
Object.ReferenceEquals(t, Value2.GetType()));
Console.WriteLine("Data type of Value1 and Value3 are equal: {0}",
Object.ReferenceEquals(t, Value3.GetType()));
Console.WriteLine("Data type of Value1 and Value4 are equal: {0}",
Object.ReferenceEquals(t, Value4.GetType()));
完全なコード例:
using System;
using System.Diagnostics;
class CompareTypeObjects {
public static void Main() {
int Value1 = 2723;
double Value2 = 123.56;
int Value3 = 1747;
long Value4 = 123456789;
Type t = Value1.GetType();
Console.WriteLine("The data type of Value1 and Value2 are equal: {0}",
Object.ReferenceEquals(t, Value2.GetType()));
Console.WriteLine("The data type of Value1 and Value3 are equal: {0}",
Object.ReferenceEquals(t, Value3.GetType()));
Console.WriteLine("The data type of Value1 and Value4 are equal: {0}",
Object.ReferenceEquals(t, Value4.GetType()));
}
}
出力:
The data type of Value1 and Value2 are equal: False
The data type of Value1 and Value3 are equal: True
The data type of Value1 and Value4 are equal: False
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn