C# でリストからアイテムを削除
Minahil Noor
2023年10月12日
Csharp
Csharp List
-
C# で
Remove()
メソッドを使用してList
からアイテムを削除する -
C# で
RemoveAt()
メソッドを使用してList
からアイテムを削除する -
C# で
RemoveRange()
メソッドを使用してList
からアイテムを削除する
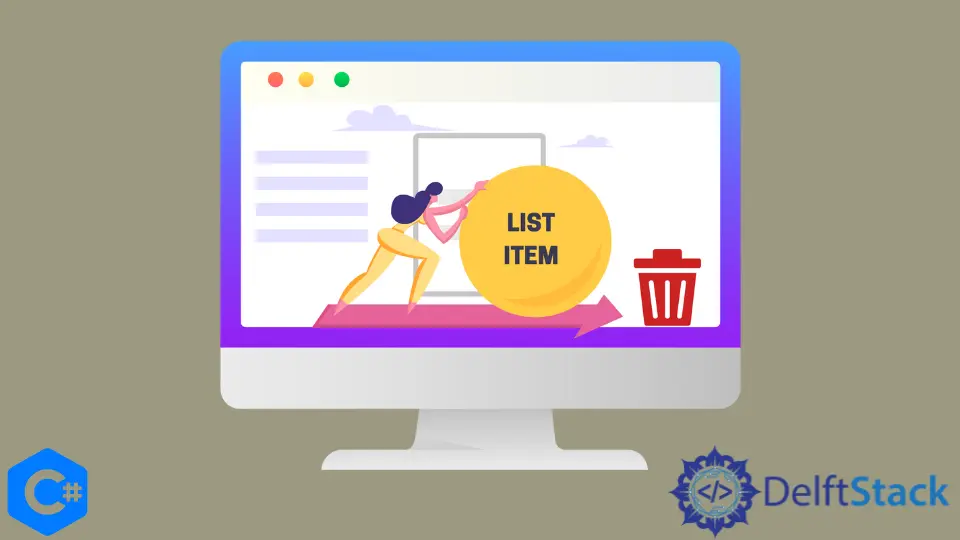
C# では、List
データ構造に対していくつかの操作を実行できます。アイテムは追加、削除、置換などができます。C# でリスト
からアイテムを削除するには、Remove()
、RemoveAt()
、RemoveRange()
メソッドを使用します。
これらのメソッドは、インデックスまたは値のいずれかに基づいて、List
からアイテムを削除します。次のいくつかの例では、これらを実装する方法を学びます。
C# で Remove()
メソッドを使用して List
からアイテムを削除する
この Remove()
メソッドは List
の名前に基づいてアイテムを削除します。このメソッドを使用するための正しい構文は次のとおりです。
C
# cCopyListName.Remove("NameOfItemInList");
コード例:
C
# cCopyusing System;
using System.Collections.Generic;
public class Removal {
public static void Main() {
List<string> Flowers = new List<string>();
Flowers.Add("Rose");
Flowers.Add("Jasmine");
Flowers.Add("Lili");
Flowers.Add("Hibiscus");
Flowers.Add("Daisy");
Console.WriteLine("List Before Removal:");
foreach (string flower in Flowers) {
Console.WriteLine(flower);
}
Console.WriteLine();
// Use of Remove() method
Flowers.Remove("Lili");
Console.WriteLine("List After Removal:");
foreach (string flower in Flowers) {
Console.WriteLine(flower);
}
}
}
出力:
textCopyList Before Removal:
Rose
Jasmine
Lili
Hibiscus
Daisy
List After Removal:
Rose
Jasmine
Hibiscus
Daisy
C# で RemoveAt()
メソッドを使用して List
からアイテムを削除する
RemoveAt()
メソッドは、アイテムのインデックス番号に基づいて、アイテムを List
から削除します。C# のインデックスは 0 で始まることは既に知っています。そのため、インデックス番号の選択には注意してください。このメソッドを使用するための正しい構文は次のとおりです。
C
# cCopyListName.RemoveAt(Index);
コード例:
C
# cCopyusing System;
using System.Collections.Generic;
public class Removal {
public static void Main() {
List<string> Flowers = new List<string>();
Flowers.Add("Rose");
Flowers.Add("Jasmine");
Flowers.Add("Lili");
Flowers.Add("Hibiscus");
Flowers.Add("Daisy");
Console.WriteLine("List Before Removal:");
foreach (string flower in Flowers) {
Console.WriteLine(flower);
}
Console.WriteLine();
// Use of RemoveAt() method
Flowers.RemoveAt(3);
Console.WriteLine("List After Removal:");
foreach (string flower in Flowers) {
Console.WriteLine(flower);
}
}
}
出力:
textCopyList Before Removal:
Rose
Jasmine
Lili
Hibiscus
Daisy
List After Removal:
Rose
Jasmine
Lili
Daisy
C# で RemoveRange()
メソッドを使用して List
からアイテムを削除する
C# では、複数のアイテムを同時に削除することもできます。この目的のために、RemoveRange()
メソッドが使用されます。削除するアイテムの範囲をパラメーターとしてメソッドに渡します。このメソッドを使用するための正しい構文は次のとおりです。
C
# cCopyListName.RemoveRange(int index, int count);
index
は削除する要素の開始インデックスで、count
は削除する要素の数です。
コード例:
C
# cCopyusing System;
using System.Collections.Generic;
public class Removal {
public static void Main() {
List<string> Flowers = new List<string>();
Flowers.Add("Rose");
Flowers.Add("Jasmine");
Flowers.Add("Lili");
Flowers.Add("Hibiscus");
Flowers.Add("Daisy");
Console.WriteLine("List Before Removal:");
foreach (string flower in Flowers) {
Console.WriteLine(flower);
}
Console.WriteLine();
// Use of RemoveRange() method
Flowers.RemoveRange(3, 2);
Console.WriteLine("List After Removal:");
foreach (string flower in Flowers) {
Console.WriteLine(flower);
}
}
}
出力:
textCopyList Before Removal:
Rose
Jasmine
Lili
Hibiscus
Daisy
List After Removal:
Rose
Jasmine
Lili
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe