C# は get メソッドに複数のパラメーターを渡する
Minahil Noor
2023年10月12日
Csharp
Csharp Method
-
C# で
コントローラーアクション
を使用して複数のパラメーターをGet
メソッドに渡す -
Attribute Routing
を使用して複数のパラメーターをGet
メソッドに渡すコードスニペット -
複数のパラメーターを
Get
メソッドを使用して[FromQuery]
に渡すコードスニペット
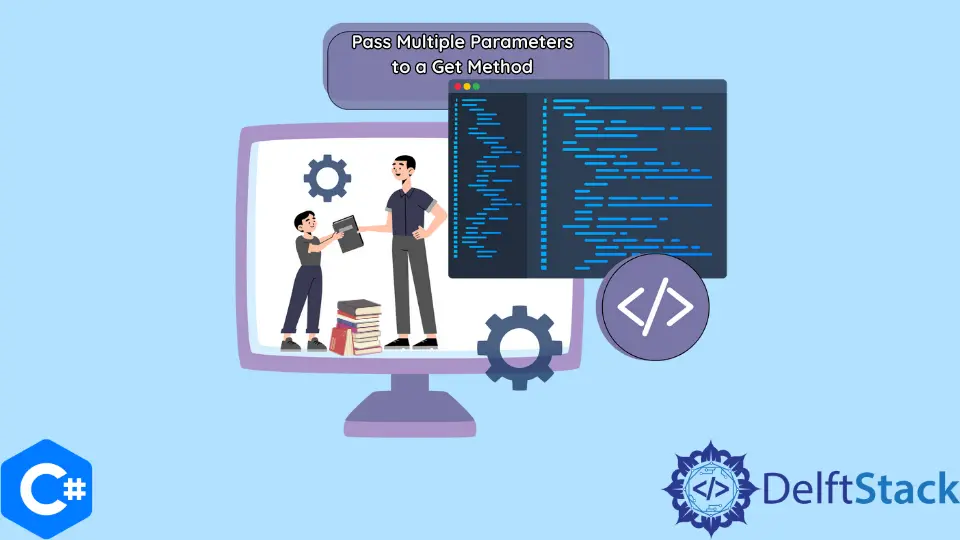
ASP.NET MVC
は Web アプリケーションの開発に使用されます。MVC Web API
と Web pages
フレームワークは、MVC 6
という名前の 1つのフレームワークに統合されました。MVC
は、ルーティングされるリクエストを介してモデル、ビュー、コントローラーとやり取りできるようにするパターンです。
この記事では、MVC
コントローラーの Get
メソッドに複数のパラメーターを渡すために使用されるさまざまなメソッドについて説明します。
C# でコントローラーアクション
を使用して複数のパラメーターを Get
メソッドに渡す
コントローラーアクション
は、着信要求を処理するために使用されるメソッドです。そしてアクション結果
を返します。アクション結果
は、着信リクエストに対する応答です。この場合、コントローラーアクションを使用して複数のパラメーターを Get
メソッドに渡しました。
コード例:
public string Get(int? id, string FirstName, string LastName, string Address) {
if (id.HasValue)
GetById(id);
else if (string.IsNullOrEmpty(Address))
GetByName(FirstName, LastName);
else
GetByNameAddress(FirstName, LastName, Address);
}
Attribute Routing
を使用して複数のパラメーターを Get
メソッドに渡すコードスニペット
attribute routing
では、attributes
を使用して routes
を定義します。エラーの可能性を減らすため、これは推奨される方法です。Web API
で URL
をより詳細に制御できます。
このメソッドを使用するための正しい構文は次のとおりです。
// Specify route
[Route("api/YOURCONTROLLER/{parameterOne}/{parameterTwo}")]
public string Get(int parameterOne, int parameterTwo) {
return $"{parameterOne}:{parameterTwo}";
}
}
コード例:
// Specify route
[Route("api/yourControllerName/{FirstName}/{LastName}/{Address}")]
public string Get(string id, string FirstName, string LastName, string Address) {
return $"{FirstName}:{LastName}:{Address}";
}
複数のパラメーターを Get
メソッドを使用して [FromQuery]
に渡すコードスニペット
[FromQuery]
は、パラメータがリクエストクエリ string
にバインドされるように指定するために使用されます。これは FromQueryAttribute
クラスのプロパティです。
このプロパティを使用するための正しい構文は次のとおりです。
[HttpGet]
public string GetByAttribute([FromQuery] string parameterOne, [FromQuery] string parameterTwo) {}
コード例:
[Route("api/person")]
public class PersonController : Controller {
[HttpGet]
// Passing one parameter to the Get method
public string GetById([FromQuery] int id) {}
[HttpGet]
// Passing two parameters to the Get method
public string GetByName([FromQuery] string firstName, [FromQuery] string lastName) {}
[HttpGet]
// Passing multiple parameters to the Get method
public string GetByNameAndAddress([FromQuery] string firstName, [FromQuery] string lastName,
[FromQuery] string address) {}
}
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe