C# でのフィールドとプロパティ
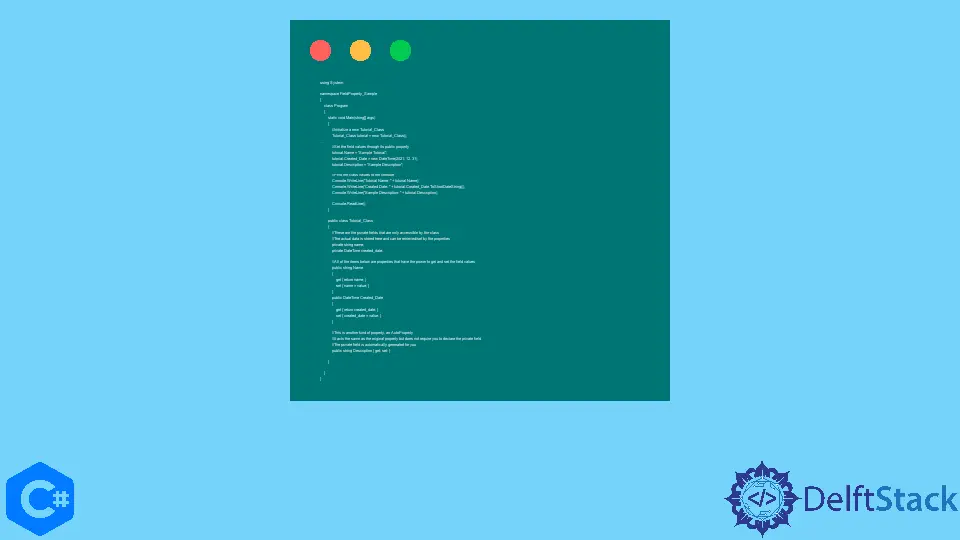
このチュートリアルでは、C# のクラスのフィールドとプロパティの違いについて説明します。これをわかりやすく説明するには、最初に次の概念を知っておく必要があります。
クラスとは何ですか
クラスは、オブジェクトの青写真またはテンプレートとして機能することにより、カスタムタイプを作成できるようにするデータメンバーのコレクションです。クラス内では、フィールドとプロパティはデータを格納できるという点で似ていますが、違いはそれらの使用方法にあります。
フィールドとは何ですか
field
はデータを変数として保存します。公開してからクラスからアクセスすることもできますが、できるだけ多くのデータを制限して非表示にすることをお勧めします。これにより、ユーザーがデータにアクセスできる唯一の方法がクラスのパブリックメソッドの 1つを利用することであることが保証されるため、より詳細な制御が可能になり、予期しないエラーが回避されます。
プロパティとは何ですか
プロパティはフィールドを公開します。フィールドの代わりにプロパティを直接使用すると、クラスを使用するオブジェクトがアクセスする外部の方法に影響を与えずにフィールドを変更できる抽象化レベルが提供されます。プロパティを使用すると、フィールドの値を設定したり、有効なデータを確認したりする前に計算を行うこともできます。
例:
using System;
namespace FieldProperty_Sample {
class Program {
static void Main(string[] args) {
// Initialize a new Tutorial_Class
Tutorial_Class tutorial = new Tutorial_Class();
// Set the field values through its public property
tutorial.Name = "Sample Tutorial";
tutorial.Created_Date = new DateTime(2021, 12, 31);
tutorial.Description = "Sample Description";
// Print the class values to the console
Console.WriteLine("Tutorial Name: " + tutorial.Name);
Console.WriteLine("Created Date: " + tutorial.Created_Date.ToShortDateString());
Console.WriteLine("Sample Description: " + tutorial.Description);
Console.ReadLine();
}
public class Tutorial_Class {
// These are the private fields that are only accessible by the class
// The actual data is stored here and can be retrieved/set by the properties
private string name;
private DateTime created_date;
// All of the items below are properties that have the power to get and set the field values
public string Name {
get { return name; }
set { name = value; }
}
public DateTime Created_Date {
get { return created_date; }
set { created_date = value; }
}
// This is another kind of property, an AutoProperty
// It acts the same as the original property but does not require you to declare the private
// field The private field is automatically generated for you
public string Description { get; set; }
}
}
}
上記の例では、フィールド、プロパティ、および AutoProperties を使用するサンプルクラスがあります。Tutorial_Class
のインスタンスを初期化した後、クラスのパブリックプロパティを使用して値を設定します。フィールドに直接アクセスしようとすると、Program.Tutorial_Class.x is inaccessible due to its protection level
というエラーが表示されることに注意してください。最後に、値をコンソールに出力して、変更が行われたことを示します。
出力:
Tutorial Name: Sample Tutorial
Created Date: 31/12/2021
Sample Description: Sample Description