C# でクラスを拡張する
Muhammad Zeeshan
2023年10月12日
Csharp
Csharp Class
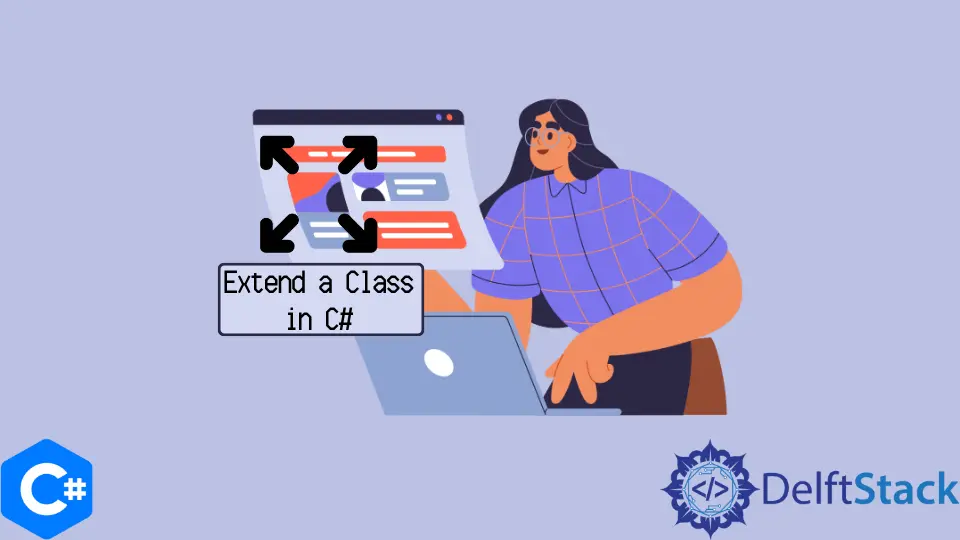
このチュートリアルでは、C# プログラミング言語を使用してクラスを拡張する方法について説明します。
継承を使用して C#
でクラスを拡張する
オブジェクト指向プログラミング (OOP
) を使用する場合、継承は階層レベルに関連付けられます。 派生
クラスをその ベース
クラスにキャストすることは可能ですが、その逆はできません。
基本
クラスから 派生
クラスにキャストすることはできません。 次の例を見て理解を深めてください。
次の例では、プロパティを一度に 1つずつ新しいオブジェクトに追加し、新しいオブジェクトを使用して前のオブジェクトから 1つのプロパティを拡張します。
-
まず、次のライブラリをインポートします。
using System; using System.Collections.Generic; using System.Linq; using System.Text.RegularExpressions;
-
Firstname
プロパティとLastname
プロパティを持つPerson
クラスを作成します。public class Person { public string Firstname { get; set; } public string Lastname { get; set; } public Person(string fname, string lname) { Firstname = fname; Lastname = lname; } }
-
Person
からStudent
を構築するために、コンストラクターのオーバーロードが使用されるようになりました。public class Student : Person { public Student(Person person, string code) : base(person.Firstname, person.Lastname) { this.code = code; } public Student(Person person) : base(person.Firstname, person.Lastname) {} public string code { get; set; } }
-
Main()
メソッドでは、Person
オブジェクトを作成し、以下のようにデータを入力します。Person person = new Person("Muhammad Zeeshan", "Khan");
-
次に、
s1
とs2
という名前の 2つのStudent
オブジェクトを作成し、person
オブジェクトとcode
をパラメーターとして渡します。Student s1 = new Student(person, "3229"); Student s2 = new Student(person, "3227");
-
最後に、
Person
プロパティを持つStudent
オブジェクトを出力します。Console.WriteLine(s1.code); Console.WriteLine(s2.code);
-
完全なソース コード。
using System; using System.Collections.Generic; using System.Linq; using System.Text.RegularExpressions; public class Person { public string Firstname { get; set; } public string Lastname { get; set; } public Person(string fname, string lname) { Firstname = fname; Lastname = lname; } } public class Student : Person { public Student(Person person, string code) : base(person.Firstname, person.Lastname) { this.code = code; } public Student(Person person) : base(person.Firstname, person.Lastname) {} public string code { get; set; } } static class Program { static void Main(string[] args) { Person person = new Person("Muhammad Zeeshan", "Khan"); Student s1 = new Student(person, "3229"); Student s2 = new Student(person, "3227"); Console.WriteLine(s1.code); Console.WriteLine(s2.code); } }
出力:
3229 3227
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe
著者: Muhammad Zeeshan
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn