C# で読み取り専用プロパティを実装する
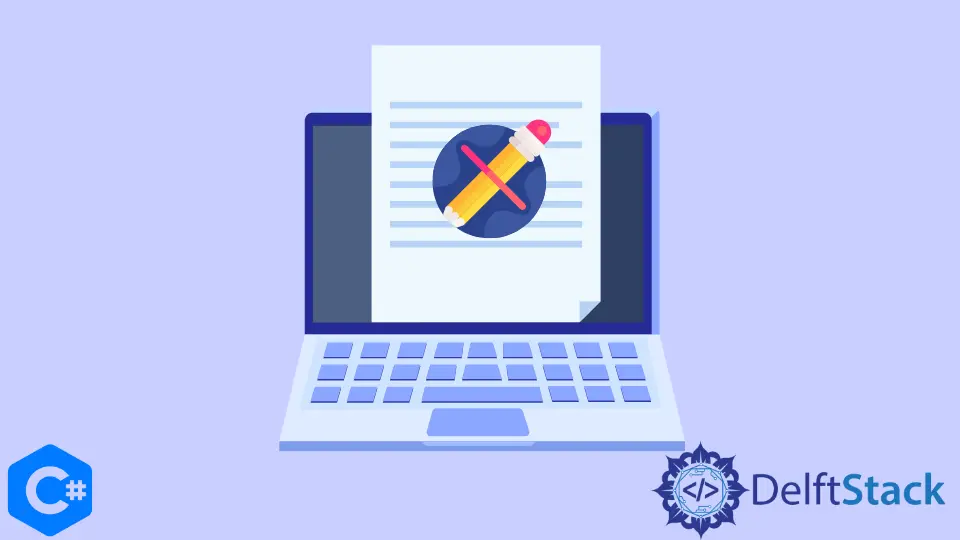
今日は、C# で読み取り専用プロパティを作成する方法を学習します。これにより、読み取りのみが可能になり、変更できなくなります。
C#
で初期化するときに変数の読み取り専用プロパティを宣言する
最後の 2つの記事では、クラス CAR
に取り組んできました。同じクラスを使用して、読み取り専用プロパティを実装してみましょう。クラス CAR
に、セキュリティのために読み取り専用のままにしておきたい maker_id
というプロパティがあるとします。
using System;
class CAR {
private readonly int maker_id;
public CAR(int maker_id) {
this.maker_id = maker_id;
}
public int get_maker_id() {
return maker_id;
}
}
public class Program {
static void Main(String[] args) {
CAR x = new CAR(5);
Console.WriteLine(x.get_maker_id());
}
}
そのため、maker_id
を読み取り専用
として宣言しました。上記を実行すると、出力は次のようになります。
5
したがって、maker_id
フィールドに別のセッターを追加したいとします。次に、次のように実行できます。
public void set_maker_id(int maker_id) {
this.maker_id = maker_id;
}
しかし、エラーがスローされることに気付くでしょう。
したがって、readonly
プロパティでは、コンストラクター内の変数に値を 1 回だけ割り当てることができます。それ以外の場合は、アクセシビリティのためにエラーをスローし、変更を拒否する傾向があります。
完全なコードを以下に示します。
using System;
class CAR {
private readonly int maker_id;
public CAR(int maker_id) {
this.maker_id = maker_id;
}
public void set_maker_id(int maker_id) {
this.maker_id = maker_id; // line ERROR
}
public int get_maker_id() {
return maker_id;
}
}
public class Program {
static void Main(String[] args) {
CAR x = new CAR(5);
Console.WriteLine(x.get_maker_id());
}
}
エラーはコードのコメントに記載されています。
C#
で単純な get
プロパティを使用して読み取り専用プロパティを実装する
maker_id
プロパティは次のように記述できます。
private int maker_id { get; }
そして、次のように関数を書き込もうとした場合:
public void set_val(int val) {
this.maker_id = val;
}
次のようなエラーが発生します。
したがって、get
プロパティを設定すると、どのように読み取り専用になるかがわかります。完全なコードは次のとおりです。
using System;
class CAR {
private int maker_id {
get;
set;
}
public CAR(int maker_id) {
this.maker_id = maker_id;
}
}
class Program {
static void Main(String[] args) {
CAR x = new CAR(5);
}
}
これは規則に従うだけでなく、シリアライザーを破る傾向があります。また、変化せず、不変になる傾向があります。
get
が何かを返すことを確認したい場合は、次のようにコードを作成します。
class CAR {
public int maker_id {
get { return maker_id; }
}
}
これが、C# で読み取り専用を実装する方法です。
Hello, I am Bilal, a research enthusiast who tends to break and make code from scratch. I dwell deep into the latest issues faced by the developer community and provide answers and different solutions. Apart from that, I am just another normal developer with a laptop, a mug of coffee, some biscuits and a thick spectacle!
GitHub