C# で JSON をサーバーに投稿する
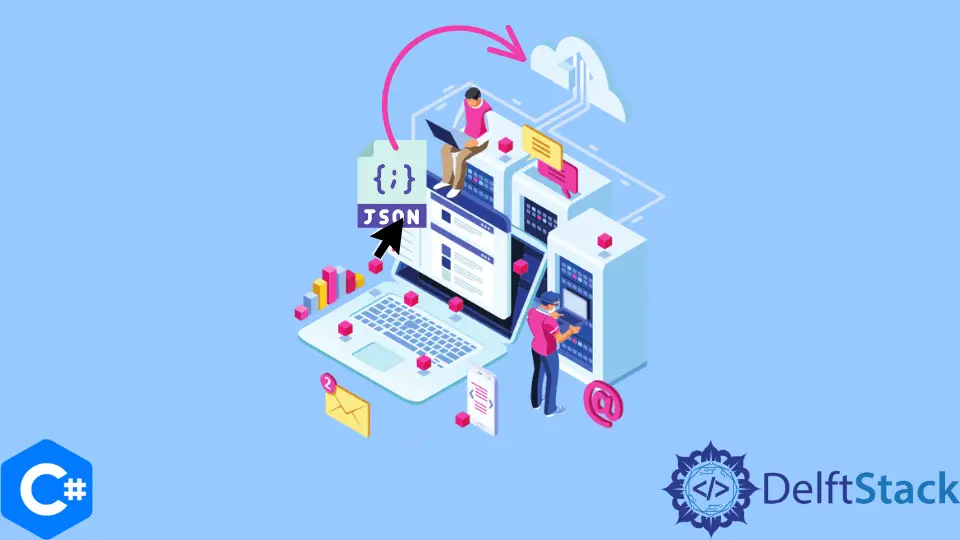
では、JSON とは何ですか? JSON は Javascript Object Notation の略です。 これは、角括弧内にカプセル化されたキーに値をマップするのと同様の構造に従います。
今日は、C# で JSON をサーバーにポストする方法を学びます。
C#
で HttpWebRequest
を使用して JSON をサーバーにポストする
Web サービスを使用するには、バックエンドで C# をサポートする Web フレームワークである .NET ASP で IDE を起動する必要があります。
起動した ASP.NET アプリケーション内で、新しい ASPX ページを追加して、JSON をテキストとしてポストするボタンと、ポストされた JSON テキストを表示する別のラベルを追加する必要があります。
ボタン:
ラベル:
Web ページは次のように表示されます。
ボタンがクリックされると、JSON がこの Web ページに投稿されます。 ボタンの下のテキストボックスに JSON が表示されます。
ボタン クリック関数内で、データを送信するためのリクエストを作成し、リクエストが成功したかどうかを示すステータス コードを読み取ります。
var url = "https://reqres.in/api/users";
var httpRequest = (HttpWebRequest)WebRequest.Create(url);
httpRequest.Method = "POST";
httpRequest.Accept = "application/json";
httpRequest.ContentType = "application/json";
var data =
@"{
""name"": ""morpheus"",
""job"": ""leader"",
""id"": ""264"",
""createdAt"": ""2022-07-09T07:33:55.681Z""
}";
using (var streamWriter = new StreamWriter(httpRequest.GetRequestStream())) {
streamWriter.Write(data);
}
var httpResponse = (HttpWebResponse)httpRequest.GetResponse();
using (var streamReader = new StreamReader(httpResponse.GetResponseStream())) {
var result = streamReader.ReadToEnd();
}
this.jsontext.Text = httpResponse.StatusCode.ToString();
ここでは、データを投稿して取得するための無料の URL である URL を使用しました。 プロセス後のステータス コードのイメージを以下に示します。
出力:
URL の httpRequest
変数を作成します。 次に、JSON に設定された Accept
要件を定義する傾向があります。 次に、streamWriter
がデータをサーバーに書き込むと、応答から取得された StatusCode
が、前に定義した jsontext
変数のテキストに入れられます。
JSON を手作りしたくない場合は、次のように Serializer
を使用できます。
string json = new JavaScriptSerializer().Serialize(
new { name = "morpheus", job = "leader", id = "264", createdAt = "2022-07-09T07:33:55.681Z" });
そして、次のように書きます。
streamWriter.Write(json);
ただし、これを実装するには、System.Web.Script.Serialization
名前空間を含める必要があります。
完全なコードを以下に示します。
using System;
using System.Collections.Generic;
using System.IO;
using System.Linq;
using System.Net;
using System.Text;
using System.Web;
using System.Web.Script.Serialization;
using System.Web.UI;
using System.Web.UI.WebControls;
namespace WebApplication2 {
public partial class WebForm1 : System.Web.UI.Page {
protected void Page_Load(object sender, EventArgs e) {}
protected void json_Click(object sender, EventArgs e) {
var url = "https://reqres.in/api/users";
var httpRequest = (HttpWebRequest)WebRequest.Create(url);
httpRequest.Method = "POST";
httpRequest.Accept = "application/json";
httpRequest.ContentType = "application/json";
// HANDCRAFTED JSON
var data =
@"{
""name"": ""morpheus"",
""job"": ""leader"",
""id"": ""264"",
""createdAt"": ""2022-07-09T07:33:55.681Z""
}";
// SERIALIZED JSON
string json =
new JavaScriptSerializer().Serialize(new { name = "morpheus", job = "leader", id = "264",
createdAt = "2022-07-09T07:33:55.681Z" });
using (var streamWriter = new StreamWriter(httpRequest.GetRequestStream())) {
streamWriter.Write(json);
}
var httpResponse = (HttpWebResponse)httpRequest.GetResponse();
using (var streamReader = new StreamReader(httpResponse.GetResponseStream())) {
var result = streamReader.ReadToEnd();
}
this.jsontext.Text = httpResponse.StatusCode.ToString();
}
}
}
C#
で new HttpClient()
を使用して JSON をサーバーにポストする
次のコードを使用して、非常に簡単に JSON をサーバーに送信できます。
using (var client = new HttpClient()) {
var result = client.PostAsync(url, new StringContent(json, Encoding.UTF8, "application/json"));
}
これは CLIENT.POSTASYNC()
を使用して POST
リクエストを発行します。 完全なコードを以下に示します。
protected void json_Click(object sender, EventArgs e) {
var url = "https://reqres.in/api/users";
var httpRequest = (HttpWebRequest)WebRequest.Create(url);
httpRequest.Method = "POST";
httpRequest.Accept = "application/json";
httpRequest.ContentType = "application/json";
// HANDCRAFTED JSON
var data =
@"{
""name"": ""morpheus"",
""job"": ""leader"",
""id"": ""264"",
""createdAt"": ""2022-07-09T07:33:55.681Z""
}";
// SERIALIZED JSON
string json =
new JavaScriptSerializer().Serialize(new { name = "morpheus", job = "leader", id = "264",
createdAt = "2022-07-09T07:33:55.681Z" });
using (var client = new HttpClient()) {
var result = client.PostAsync(url, new StringContent(json, Encoding.UTF8, "application/json"));
}
// using (var streamWriter = new StreamWriter(httpRequest.GetRequestStream()))
//{
// streamWriter.Write(json);
// }
var httpResponse = (HttpWebResponse)httpRequest.GetResponse();
using (var streamReader = new StreamReader(httpResponse.GetResponseStream())) {
var result = streamReader.ReadToEnd();
}
this.jsontext.Text = httpResponse.StatusCode.ToString();
}
上記の関数を置き換えて、結果を表示します。 コードは完全に機能します。
Hello, I am Bilal, a research enthusiast who tends to break and make code from scratch. I dwell deep into the latest issues faced by the developer community and provide answers and different solutions. Apart from that, I am just another normal developer with a laptop, a mug of coffee, some biscuits and a thick spectacle!
GitHub関連記事 - Csharp JSON
- C# 解析 JSON
- C# オブジェクトを JSON 文字列に変換する
- C# で JSON をファイルに書き込む
- C# で JSON を逆シリアル化する
- JSON 文字列を C# オブジェクトに変換する