C# で代入演算子をオーバーロードする
Muhammad Zeeshan
2023年10月12日
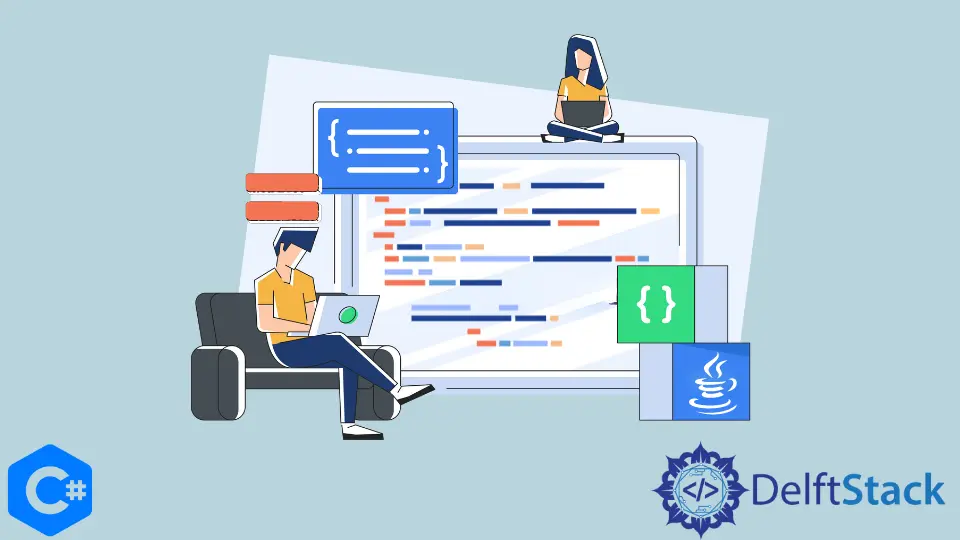
この記事では、C# を使用して代入演算子をオーバーロードする方法について説明します。 まず、演算子のオーバーロードを見てみましょう。
C#
での演算子のオーバーロード
組み込み演算子を再定義する方法は、演算子のオーバーロードと呼ばれます。 一方または両方のオペランドがユーザー定義型の場合、さまざまな操作のユーザー定義実装を作成できます。
暗黙的な変換演算子を使用して C#
で代入演算子のオーバーロードを実装する
暗黙的な変換操作を開発できます。 それらを不変の構造体にすることは、もう 1つの賢明な方法です。
プリミティブはまさにそれであり、それがそれらから継承することを不可能にしています。
以下の例では、暗黙的な変換演算子を、加算およびその他の演算子と共に開発します。
-
まず、次のライブラリをインポートします。
using System; using System.Collections.Generic; using System.Linq; using System.Text.RegularExpressions;
-
Velocity
という名前の構造体を作成し、value
という double 変数を作成します。public struct Velocity { private readonly double value; }
-
Velocity
に、変数をパラメーターとして受け取る publicVelocity
を作成するように指示します。public Velocity(double value) { this.value = value; }
-
次に、暗黙の演算子
Velocity
を作成します。public static implicit operator Velocity(double value) { return new Velocity(value); }
-
次に、オーバーロードする
Addition
およびSubtraction
演算子を作成します。public static Velocity operator +(Velocity first, Velocity second) { return new Velocity(first.value + second.value); } public static Velocity operator -(Velocity first, Velocity second) { return new Velocity(first.value - second.value); }
-
最後に、
Main()
メソッドで、Velocity
のオブジェクトを呼び出してオーバーロードします。static void Main() { Velocity v = 0; v = 17.4; v += 9.8; }
完全なソース コード:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text.RegularExpressions;
public struct Velocity {
private readonly double value;
public Velocity(double value) {
this.value = value;
}
public static implicit operator Velocity(double value) {
return new Velocity(value);
}
public static Velocity operator +(Velocity first, Velocity second) {
return new Velocity(first.value + second.value);
}
public static Velocity operator -(Velocity first, Velocity second) {
return new Velocity(first.value - second.value);
}
}
class Example {
static void Main() {
Velocity v = 0;
v = 17.4;
v += 9.8;
}
}
著者: Muhammad Zeeshan
I have been working as a Flutter app developer for a year now. Firebase and SQLite have been crucial in the development of my android apps. I have experience with C#, Windows Form Based C#, C, Java, PHP on WampServer, and HTML/CSS on MYSQL, and I have authored articles on their theory and issue solving. I'm a senior in an undergraduate program for a bachelor's degree in Information Technology.
LinkedIn