C# における LINQ Group by
Muhammad Maisam Abbas
2023年10月12日
Csharp
Csharp LINQ
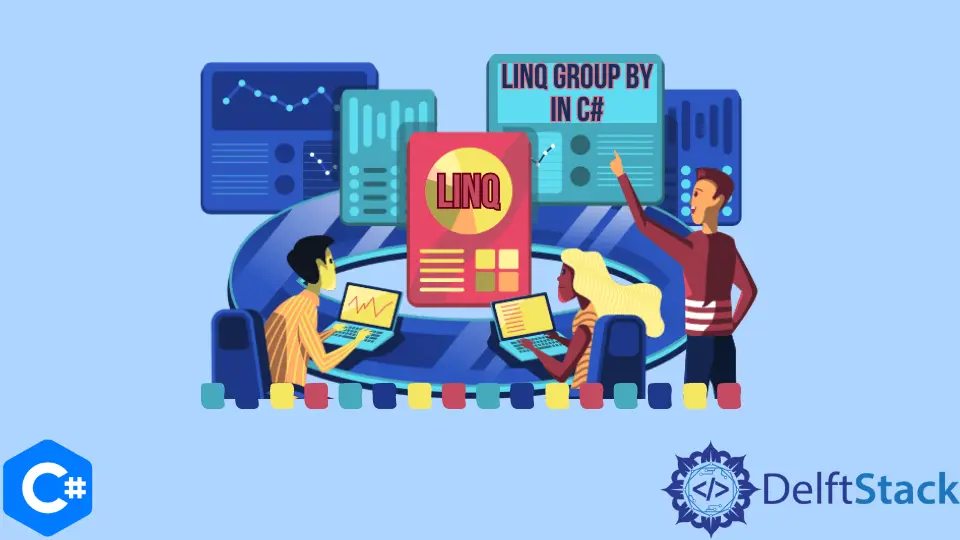
このチュートリアルでは、オブジェクトのリストを C# の値でグループ化する方法について説明します。
C# における LINQ の Group by
LINQ は、SQL のようなクエリ機能を C# のデータ構造と統合します。
次のクラスのオブジェクトのリストがあるとします。
class Car {
public string Brand { get; set; }
public int Model { get; set; }
}
Brand
は車のブランド名、Model
は車の型番です。複数のオブジェクトの Brand
プロパティは同じでもかまいませんが、Model
番号はオブジェクトごとに異なる必要があります。オブジェクトのリストをブランド名でグループ化する場合は、LINQ の GroupBy
メソッドを使用できます。次のコード例は、LINQ の GroupBy
メソッドを使用して、特定のクラスのオブジェクトをある値でグループ化する方法を示しています。
using System;
using System.Collections.Generic;
using System.Linq;
namespace linq_goup_by {
public class Car {
public string Brand { get; set; }
public int Model { get; set; }
public Car(string b, int m) {
Brand = b;
Model = m;
}
}
class Program {
static void Main(string[] args) {
List<Car> cars = new List<Car>();
cars.Add(new Car("Brand 1", 11));
cars.Add(new Car("Brand 1", 22));
cars.Add(new Car("Brand 2", 12));
cars.Add(new Car("Brand 2", 21));
var results = from c in cars group c by c.Brand;
foreach (var r in results) {
Console.WriteLine(r.Key);
foreach (Car c in r) {
Console.WriteLine(c.Model);
}
}
}
}
}
出力:
Brand 1
11
22
Brand 2
12
21
上記のコードでは、最初にオブジェクト cars
のリストを宣言して初期化し、次に値を Brand
プロパティでグループ化して、result
変数に保存しました。
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe
Maisam is a highly skilled and motivated Data Scientist. He has over 4 years of experience with Python programming language. He loves solving complex problems and sharing his results on the internet.
LinkedIn