C# の DateTime に null を設定する
Haider Ali
2023年10月12日
Csharp
Csharp DateTime
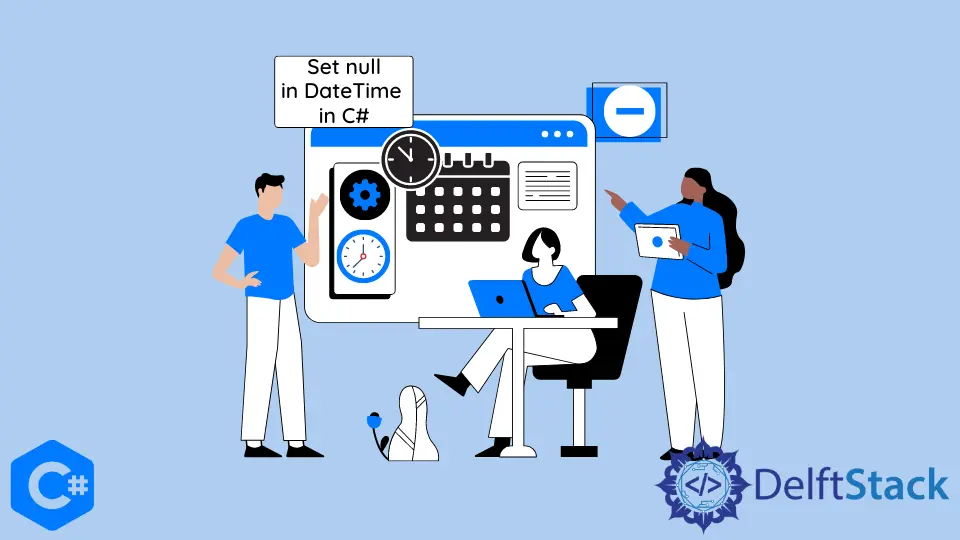
このレッスンでは、DateTime
に null
値を設定する方法を説明します。この概念を完全に理解するには、DateTime
の基本と null 許容値に精通している必要があります。
C#
の DateTime
の基本を理解する
ユーザーが 2015 年 12 月 25 日の日の始まりから時計を開始したいとします。DateTime
オブジェクトに値を割り当てます。
コードスニペット:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace nulldatetime {
class Program {
static void Main(string[] args) {
DateTime date1 = new DateTime(2015, 12, 25); // Assgining User Defined Time ;
Console.WriteLine("Time " + date1);
Console.ReadKey();
}
}
}
出力:
Time 12/25/2015 12:00:00 AM
C#
の DateTime
に最大値と最小値を割り当てる
最小値を DateTime
に割り当てると、開始最小時間:1/1/000112:00:00AM
から時刻が開始されます。同じことが最大時間にも当てはまり、時間の最大値最大時間:12/31/999911:59:59PM
で時間を開始します。
コードスニペット:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace nulldatetime {
class Program {
static void Main(string[] args) {
// How to Define an uninitialized date. ?
// First Method to use MinValue field of datetime object
DateTime date2 = DateTime.MinValue; // minimum date value
Console.WriteLine("Time: " + date2);
// OR
date2 = DateTime.MaxValue;
Console.WriteLine("Max Time: " + date2);
Console.ReadKey();
}
}
}
出力:
Min Time: 1/1/0001 12:00:00 AM
Max Time: 12/31/9999 11:59:59 PM
C#
の DateTime
に null
値を割り当てる
DateTime
は、デフォルトでは値型であるため、null 許容ではありません。値型は、メモリ割り当てに格納されているデータの形式です。
一方、NullableDateTime
を使用する場合。それに null
値を割り当てることができます。
コードスニペット:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace nulldatetime {
class Program {
static void Main(string[] args) {
// By default DateTime is not nullable because it is a Value Type;
// Problem # Assgin Null value to datetime instead of MAX OR MIN Value.
// Using Nullable Type
Nullable<DateTime> nulldatetime; // variable declaration
nulldatetime = DateTime.Now; // Assgining DateTime to Nullable Object.
Console.WriteLine("Current Date Is " + nulldatetime); // printing Date..
nulldatetime = null; // assgining null to datetime object.
Console.WriteLine("My Value is null ::" + nulldatetime);
Console.ReadKey();
}
}
}
出力:
Current Date Is 02/11/2022 18:57:33
My Value is null ::
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe
著者: Haider Ali
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn