C# で CURL 呼び出しを行う
-
C#
でHttpWebRequest
またはHttpWebResponse
を使用して CURL 呼び出しを行う -
C#
でWebClient
を使用して CURL 呼び出しを行う -
C#
でHttpClient
を使用して CURL 呼び出しを行う - まとめ
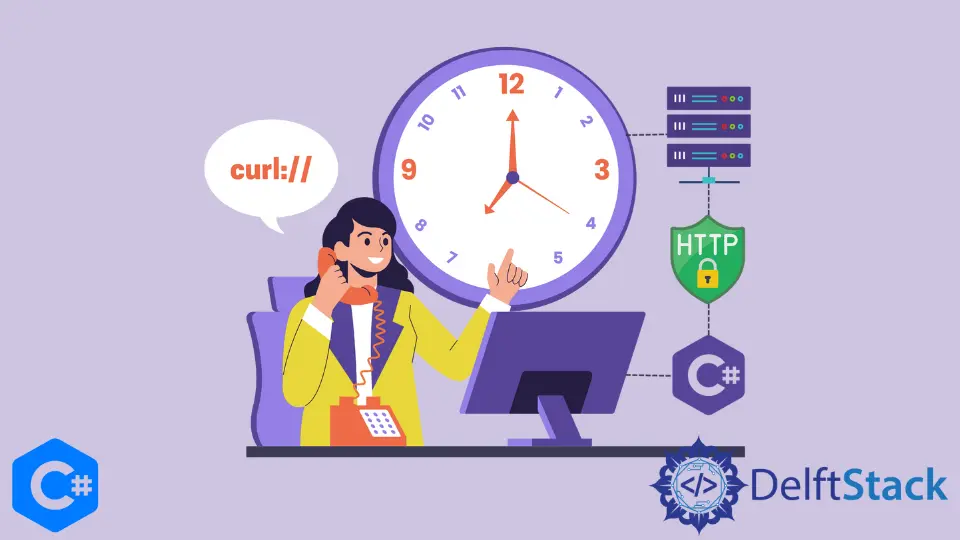
クライアント URL (cURL) は、C# の API ドキュメントのデファクト スタンダードです。 ある形式から別の形式への変換が必要です。
したがって、JSON を C# クラスに変換することは、かなり一般的なアプローチです。 Web またはホストからの応答オブジェクトも C# クラスに変換され、開発者にとってより使いやすくなっています。
この記事では、C# で cURL 呼び出しを行うための 3つの方法について説明します。
C# で cURL を使用して送信する場所とデータを指定して、サーバーとの通信を作成します。 URL はすべての cURL コマンドに従うため、常に何らかのデータを取得し、cURL の HTML ソースを返します。
cURL は移植性が高く、ほぼすべての OS および接続されたデバイスと互換性があります。 C# では、cURL はエンドポイントのテストに役立ち、送受信された内容の正確な詳細を提供します。これは、エラー ログとデバッグに適しています。
cURL は libcurl
を使用するため、libcurl
と同じ範囲の一般的なインターネット プロトコルをサポートします。
C#
で HttpWebRequest
または HttpWebResponse
を使用して CURL 呼び出しを行う
HttpWebRequest
クラスは、WebRequest
で定義されたメソッドとプロパティをサポートします。 その追加のプロパティとメソッドにより、ユーザーは HTTP を使用してサーバーと直接対話できます。
WebRequest.Create
メソッドを使用して、新しい HttpWebRequest
オブジェクトを初期化します。 GetResponse
メソッドを使用して、RequestUri
プロパティで指定されたリソースに同期リクエストを送信します。
応答オブジェクトを含む HttpWebResponse
を返します。 クローズド レスポンス オブジェクトの場合、残りのデータは強化されます。
キープアライブまたはパイプライン化されたリクエストです。 短い間隔で少量のデータのみを受信する必要があります。 C# では、.NET フレームワークに新しいセキュリティ機能が含まれています。これは、既定でより安全な動作を取得する接続およびターゲットの安全でない暗号およびハッシュ アルゴリズムをブロックします。
// create a separate class
// Include the `System.Net` and `System.IO` into your C# project and install NuGet `Newtonsoft.Json`
// package before executing the C# code.
using System.Net;
using System.IO;
using Newtonsoft.Json;
namespace cURLCall {
public class gta_allCustomersResponse {
public static void gta_AllCustomers() {
var httpWebRequest = (HttpWebRequest)WebRequest.Create(
"https://api.somewhere.com/desk/external_api/v1/customers.json");
httpWebRequest.ContentType = "application/json";
httpWebRequest.Accept = "*/*";
httpWebRequest.Method = "GET";
httpWebRequest.Headers.Add("Authorization", "Basic reallylongstring");
var httpResponse = (HttpWebResponse)httpWebRequest.GetResponse();
using (var streamReader = new StreamReader(httpResponse.GetResponseStream())) {
gta_allCustomersResponse answer =
JsonConvert.DeserializeObject<gta_allCustomersResponse>(streamReader.ReadToEnd());
// return answer;
}
}
}
}
gta_allCustomersResponse
クラスの gta_AllCustomers()
メソッドをメイン フォームに呼び出して、cURL 呼び出しを行います。
using static cURLCall.gta_allCustomersResponse;
gta_AllCustomers();
HttpWebResponse
は、WebResponse
クラスのプロパティとメソッドの HTTP 固有のユーザーをサポートして、C# で cURL 呼び出しを行います。 HttpWebResponse
クラスのインスタンスを作成し、Stream.Close
または HttpWebResponse.Close
メソッドを呼び出して応答を閉じ、応答が正常に受信されたら再利用するために接続を解放します。
C#
で WebClient
を使用して CURL 呼び出しを行う
WebClient
クラスは、URI で識別されるローカルまたはインターネット リソースを介して、データ変換または受信メソッドを提供します。 WebRequest
クラスを使用して、リソースへのアクセスを提供します。
WebClient
は、HttpWebRequest
の上に構築された高レベルの抽象化であり、C# で cURL 呼び出しを行うための最も一般的なタスクを簡素化します。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Net;
using Newtonsoft.Json;
namespace cURLCall {
public static class StaticItems {
public static string EndPoint = "http://localhost:8088/api/";
}
public class CityInfo {
public int CityID { get; set; }
public int CountryID { get; set; }
public int StateID { get; set; }
public string Name { get; set; }
public decimal Latitude { get; set; }
public decimal Longitude { get; set; }
}
public class receiveData {
public List<CityInfo> CityGet() {
try {
using (WebClient webClient = new WebClient()) {
webClient.BaseAddress = StaticItems.EndPoint;
var json = webClient.DownloadString("City/CityGetForDDL");
var list = JsonConvert.DeserializeObject<List<CityInfo>>(json);
return list.ToList();
}
} catch (WebException ex) {
throw ex;
}
}
}
public class ReturnMessageInfo {
public ReturnMessageInfo CitySave(CityInfo city) {
ReturnMessageInfo result = new ReturnMessageInfo();
try {
using (WebClient webClient = new WebClient()) {
webClient.BaseAddress = StaticItems.EndPoint;
var url = "City/CityAddUpdate";
webClient.Headers.Add(
"user-agent",
"Mozilla/4.0 (compatible; MSIE 6.0; Windows NT 5.2; .NET CLR 1.0.3705;)");
webClient.Headers[HttpRequestHeader.ContentType] = "application/json";
string data = JsonConvert.SerializeObject(city);
var response = webClient.UploadString(url, data);
result = JsonConvert.DeserializeObject<ReturnMessageInfo>(response);
return result;
}
} catch (Exception ex) {
throw ex;
}
}
}
}
FileWebRequest
や FtpWebRequest
のようなクラスは WebRequest
を継承しています。 一般に、WebRequest
を使用してリクエストを作成し、リクエストに応じて、リターンを HttpWebRequest
、FileWebRequest
、または FtpWebRequest
に変換できます。
これは、URI によって識別されるリソースからデータを送受信するための一般的な操作を提供することにより、cURL 呼び出しを行う理想的な方法の 1つです。
C#
で HttpClient
を使用して CURL 呼び出しを行う
HttpClient
クラスは、HTTP リクエストを送信し、URI によって識別されるリソースから HTTP レスポンスを受信するメソッドを提供する他のどのクラスよりもはるかに優れているように設計されています。 そのインスタンスは、HTTP リクエストを送信するセッションとして機能するすべてのリクエストに適用される設定のコレクションです。
さらに、すべての HttpClient
インスタンスは、cURL 呼び出しごとに接続プールを使用します。
C# では、HttpClient
は一度インスタンス化され、アプリケーションの存続期間を通じて再利用されることを意図しています。 上位レベルの API として、HttpClient
は利用可能な各プラットフォーム (それが実行されるプラットフォームを含む) で下位レベルの機能をラップします。
さらに、このクラスの動作はカスタマイズ可能であり、特に HTTP 要求のパフォーマンスを大幅に向上させることができます。 .NET のランタイム構成オプションは、HttpClient
を構成してその動作をカスタマイズできます。
using System;
using System.Text;
using System.Threading.Tasks;
using System.Net.Http;
using Newtonsoft.Json;
class UserInfo {
public string UserName { get; set; }
public string Email { get; set; }
public override string ToString() {
return $"{UserName}: {Email}";
}
}
class cURLCall {
static async Task Main(string[] args) {
var user = new UserInfo();
user.UserName = "My Name";
user.Email = "myname4321@exp.com";
var json = JsonConvert.SerializeObject(person);
var data = new StringContent(json, Encoding.UTF8, "application/json");
var url = "https://httpbin.org/post";
var client = new HttpClient();
var response = await client.PostAsync(url, data);
string result = response.Content.ReadAsStringAsync().Result;
// processed user info thorugh HttpClient
Console.WriteLine(result);
}
}
HttpClient
クラスは、さまざまな応答タイプの処理をより適切にサポートし、前述のオプションよりも非同期操作をより適切にサポートします。
まとめ
この記事では、C# で cURL 呼び出しを行うための 3つの便利な方法を説明しました。 C# コードを使用して cURL 呼び出しを行い、エンドポイントをテストして C# アプリケーションをトラブルシューティングできるようになりました。
Hassan is a Software Engineer with a well-developed set of programming skills. He uses his knowledge and writing capabilities to produce interesting-to-read technical articles.
GitHub