C# で文字列配列を Int 配列に変換する
Haider Ali
2023年12月11日
Csharp
Csharp Array
-
C#
でArray.ConvertAll()
メソッドを使用して文字列配列を Int 配列に変換する -
C#
で LINQ のSelect()
メソッドを使用して文字列配列を Int 配列に変換する
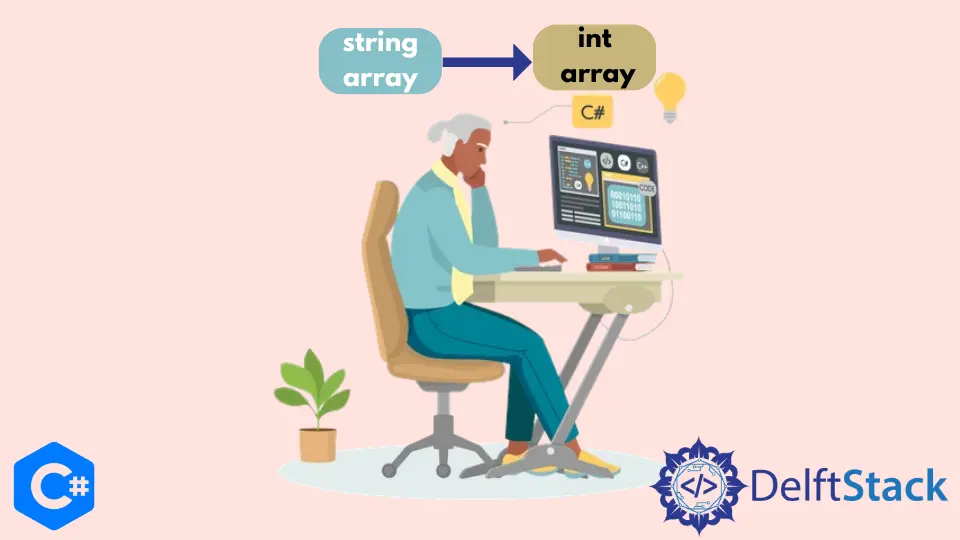
このガイドでは、C# で文字列配列を int 配列に変換する方法について説明します。
String を int に変換する方法は 2つあります。これらの方法はどちらも非常に単純で、実装も非常に簡単です。
C#
で Array.ConvertAll()
メソッドを使用して文字列配列を Int 配列に変換する
文字列の内容を理解して文字列を別のデータ型に変換することについて話すときはいつでも、構文解析が必要です。たとえば、文字列 321
は 321 に変換できます。
使用できる最初のメソッドは、Array.ConvertAll()
メソッドです。このメソッドの実装を見てみましょう。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Linq;
namespace Array_of_String_to_integer {
class Program {
static void Main(string[] args) {
// method 1 using Array.ConvertAll
string[] temp_str = new string[] { "1000", "2000", "3000" };
int[] temp_int = Array.ConvertAll(temp_str, s => int.Parse(s));
for (int i = 0; i < temp_int.Length; i++) {
Console.WriteLine(temp_int[i]); // Printing After Conversion.............
}
Console.ReadKey();
}
}
}
上記のコードで Array.Convertall()
メソッドを使用して、配列を渡します。int.Parse()
を使用して、文字列配列を int 配列に解析しています。
出力:
1000
2000
3000
C#
で LINQ の Select()
メソッドを使用して文字列配列を Int 配列に変換する
次のメソッドを使用して、int.Parse()
メソッドを LINQ の Select()
メソッドに渡すことにより、文字列配列を int 配列に変換できます。また、配列を取得するには、ToArray()
メソッドを呼び出す必要があります。
実装を見てみましょう。LINQ の詳細についてはこちらをご覧ください。
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
using System.Linq;
namespace Array_of_String_to_integer {
class Program {
static void Main(string[] args) {
Console.WriteLine("===================Using LINQ=======================================");
// Method Using LINQ.........................
// We can also pass the int.Parse() method to LINQ's Select() method and then call ToArray to
// get an array.
string[] temp_str = new string[] { "1000", "2000", "3000" };
int[] temp_int1 = temp_str.Select(int.Parse).ToArray();
for (int i = 0; i < temp_int1.Length; i++) {
Console.WriteLine(temp_int1[i]); // Printing After Conversion.............
}
Console.ReadKey();
}
}
}
出力:
===================Using LINQ=======================================
1000
2000
3000
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe
著者: Haider Ali
Haider specializes in technical writing. He has a solid background in computer science that allows him to create engaging, original, and compelling technical tutorials. In his free time, he enjoys adding new skills to his repertoire and watching Netflix.
LinkedIn