C# で入力ダイアログボックスを作成する
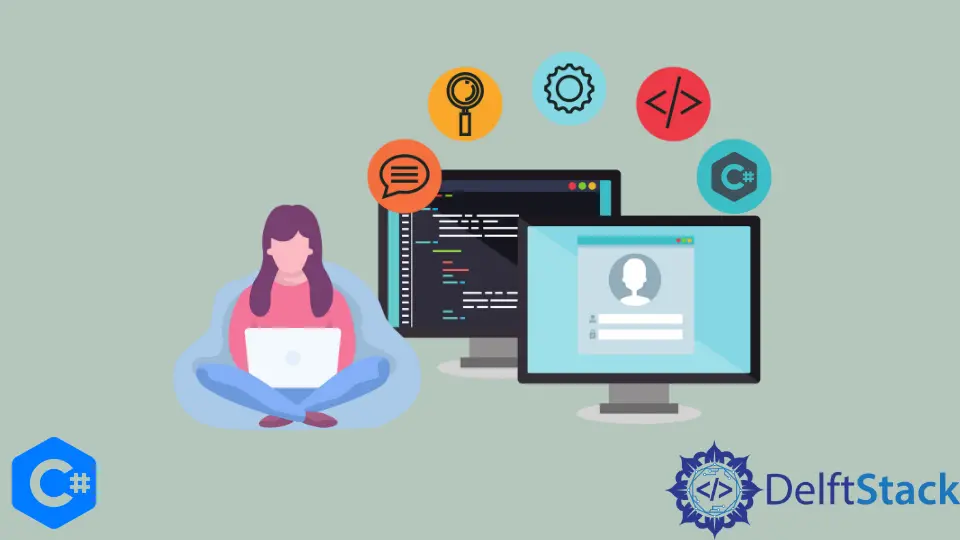
このチュートリアルでは、VB.Net と同様の 2つの異なる方法を使用して、C# で入力ダイアログボックスを作成する方法を示します。
入力ダイアログボックスは、メッセージプロンプトを表示し、ユーザーからの入力を要求するポップアップウィンドウです。その後、入力をコードで使用できます。
C# には VB.NET の入力ダイアログボックスのバージョンがないため、2つの方法のいずれかを使用できます。最初で最も簡単なのは、Microsoft.VisualBasic.Interaction
で提供される InputBox
を使用することです。もう 1つの方法は、System.Windows.Forms
と System.Drawing
を使用して独自のカスタムダイアログボックスを作成することです。
Microsoft Visual Basic を使用した入力ダイアログボックス
C# には VB.NET のような独自のバージョンの入力ダイアログボックスがないため、Microsoft.VisualBasic
への参照を追加して、その InputBox
を使用できます。
Microsoft.VisualBasic
を利用するには、最初に以下の手順に従ってプロジェクトの参照に追加する必要があります。
-
ソリューションエクスプローラーに移動します
-
参照を右クリックします
-
参照の追加
をクリックします -
左側の
Assemblies
タブをクリックします -
Microsoft.VisualBasic.dll
ファイルを見つけて、OK
をクリックします
参照を正常に追加したら、コードでその using ステートメントを使用できます。
using Microsoft.VisualBasic;
入力ボックス自体は、次のパラメータを指定して作成されます。
- プロンプト:ウィンドウ内に表示されるメッセージ。これは、渡す必要がある唯一の必須パラメーターです。
- タイトル:表示されるウィンドウタイトル
- デフォルト:入力テキストボックスのデフォルト値
- X 座標:入力ボックスの起動位置の X 座標
- Y 座標:入力ボックスの起動位置の Y 座標
string input = Interaction.InputBox("Prompt", "Title", "Default", 10, 10);
この入力ボックスが呼び出されると、プログラムは応答を待ってから残りのコードを続行します。
例:
using System;
using Microsoft.VisualBasic;
namespace VisualBasic_Example {
class Program {
static void Main(string[] args) {
// Create the input dialog box with the parameters below
string input =
Interaction.InputBox("What is at the end of the rainbow?", "Riddle", "...", 10, 10);
// After the user has provided input, print to the console
Console.WriteLine(input);
Console.ReadLine();
}
}
}
上記の例では、画面の左上隅に表示されるなぞなぞプロンプトを含む入力ボックスを作成します。入力が送信されると、値がコンソールに出力されます。
出力:
The letter w
C# で Windows フォームを使用するカスタム入力ダイアログボックス
入力ダイアログボックスを作成するための別のオプションは、独自のカスタム入力ダイアログボックスを作成することです。入力ダイアログボックスを作成する利点の 1つは、最初の方法よりもウィンドウをカスタマイズできることです。以下の例のコードを利用するには、最初に以下の手順を使用して、System.Windows.Forms.dll
および System.Drawing.dll
への参照を追加する必要があります。
-
ソリューションエクスプローラーに移動します
-
参照を右クリックします
-
参照の追加
をクリックします -
左側の
Assemblies
タブをクリックします -
Microsoft.VisualBasic.dll
およびSystem.Drawing.dll
ファイルを見つけて、OK
をクリックします
参照を正常に追加した後、コードでそれらの using ステートメントを使用できます。
例:
using System;
using System.Windows.Forms;
using System.Drawing;
namespace CustomDialog_Example {
class Program {
static void Main(string[] args) {
// Initialize the input variable which will be referenced by the custom input dialog box
string input = "...";
// Display the custom input dialog box with the following prompt, window title, and dimensions
ShowInputDialogBox(ref input, "What is at the end of the rainbow?", "Riddle", 300, 200);
// Print the input provided by the user
Console.WriteLine(input);
Console.ReadLine();
}
private static DialogResult ShowInputDialogBox(ref string input, string prompt,
string title = "Title", int width = 300,
int height = 200) {
// This function creates the custom input dialog box by individually creating the different
// window elements and adding them to the dialog box
// Specify the size of the window using the parameters passed
Size size = new Size(width, height);
// Create a new form using a System.Windows Form
Form inputBox = new Form();
inputBox.FormBorderStyle = FormBorderStyle.FixedDialog;
inputBox.ClientSize = size;
// Set the window title using the parameter passed
inputBox.Text = title;
// Create a new label to hold the prompt
Label label = new Label();
label.Text = prompt;
label.Location = new Point(5, 5);
label.Width = size.Width - 10;
inputBox.Controls.Add(label);
// Create a textbox to accept the user's input
TextBox textBox = new TextBox();
textBox.Size = new Size(size.Width - 10, 23);
textBox.Location = new Point(5, label.Location.Y + 20);
textBox.Text = input;
inputBox.Controls.Add(textBox);
// Create an OK Button
Button okButton = new Button();
okButton.DialogResult = DialogResult.OK;
okButton.Name = "okButton";
okButton.Size = new Size(75, 23);
okButton.Text = "&OK";
okButton.Location = new Point(size.Width - 80 - 80, size.Height - 30);
inputBox.Controls.Add(okButton);
// Create a Cancel Button
Button cancelButton = new Button();
cancelButton.DialogResult = DialogResult.Cancel;
cancelButton.Name = "cancelButton";
cancelButton.Size = new Size(75, 23);
cancelButton.Text = "&Cancel";
cancelButton.Location = new Point(size.Width - 80, size.Height - 30);
inputBox.Controls.Add(cancelButton);
// Set the input box's buttons to the created OK and Cancel Buttons respectively so the window
// appropriately behaves with the button clicks
inputBox.AcceptButton = okButton;
inputBox.CancelButton = cancelButton;
// Show the window dialog box
DialogResult result = inputBox.ShowDialog();
input = textBox.Text;
// After input has been submitted, return the input value
return result;
}
}
}
上記の例では、System.Windows.Forms
の要素を使用してダイアログボックスに個別に追加するカスタム関数を作成しました。さまざまな要素をハードコーディングすることもできますが、パラメーターを追加して関数内でそれらを参照し、必要なだけカスタマイズすることもできます。ダイアログボックスを作成して表示した後、プログラムはユーザーがコンソール内でそれを出力するための入力を提供するのを待ちます。
出力:
the letter w