C# でブール値を整数に変換する
-
C#
でConvertToInt32
ステートメントを使用してブール値を整数に変換する -
C#
で三元条件演算子を使用してブール値を整数に変換する -
C#
でif
ステートメントを使用してブール値から整数に変換する
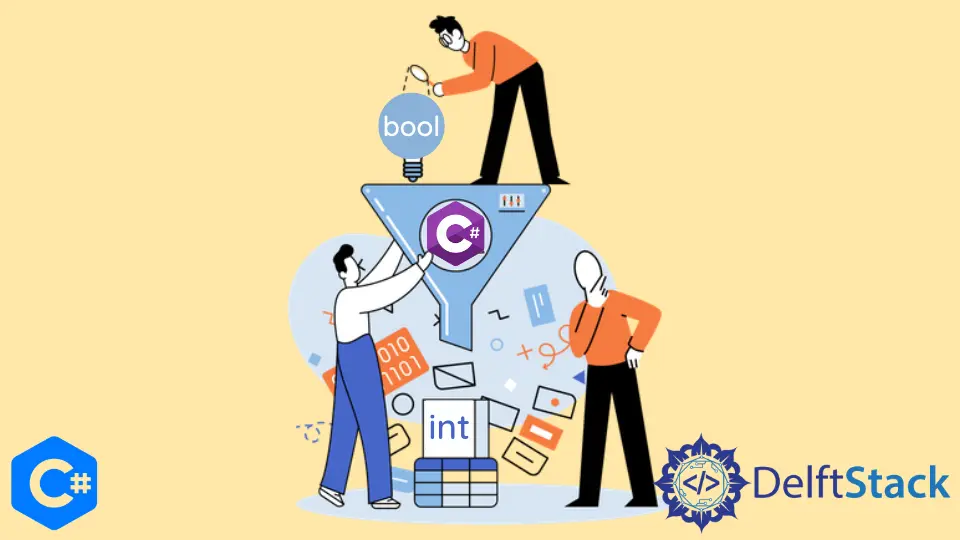
この記事では、ブールデータ型を C# で整数に変換する方法を紹介します。
C#
で ConvertToInt32
ステートメントを使用してブール値を整数に変換する
従来、データ型をブール値から整数に暗黙的に変換することはありません。ただし、Convert.ToInt32()
メソッドは、指定された値を 32 ビットの符号付き整数に変換します。
Convert.ToInt32()
メソッドは int.Parse()
メソッドに似ていますが、int.Parse()
メソッドは引数として文字列データ型のみを受け入れ、エラーをスローすることは言及する価値があります null が引数として渡されたとき。
比較すると、Convert.ToInt32()
メソッドはこれらの制限の影響を受けません。
詳細については、このリファレンスを参照してください。
以下は、Convert.ToInt32()
メソッドを使用したコードの例です。
// C# program to illustrate the
// use of Convert.ToInt32 statement
// and Convert.ToBoolean
using System;
class Test {
// Main Method
static public void Main() {
bool boolinput = true;
int intRresult = Convert.ToInt32(boolinput);
bool boolRresult = Convert.ToBoolean(intRresult);
Console.Write("When Boolean is True, the converted integer value is: ");
Console.WriteLine(intRresult);
Console.Write("When integer is 1, the converted boolean value is: ");
Console.WriteLine(boolRresult);
}
}
出力:
When Boolean is True, the converted integer value is: 1
When integer is 1, the converted boolean value is: True
以下は、int.Parse()
メソッドを使用したコードの例です。
// C# program to illustrate the
// use of int.Parse statement
using System;
class Test {
// Main Method
static public void Main() {
bool boolinput = true;
int intRresult = int.Parse(boolinput);
Console.Write("When Boolean is True, the converted integer value is: ");
Console.WriteLine(intRresult);
Console.Write("When integer is 1, the converted boolean value is: ");
}
}
出力:
program.cs(12,30): error CS1503: Argument 1: cannot convert from 'bool' to 'string'
上記のエラーは、int.Parse
メソッドを使用して Boolean
データ型を integer
に変換することで表示されます。このメソッドは、ブール
データ型ではなく、引数として文字列
を期待していることがわかります。
C#
で switch
ステートメントを使用してブール値を整数に変換する
switch
ステートメントは、プログラムの実行中に条件付きで分岐するために使用されます。コードブロックの実行を決定します。式の値は、各ケースの値と比較されます。
一致するものがある場合、関連するコードブロックが実行されます。switch
式は 1 回評価されます。
以下は、switch
ステートメントを使用したコードの例です。
// C# program to illustrate the
// use of switch statement
using System;
namespace Test {
class Program {
static void Main(string[] args) {
int i = 1;
bool b = true;
switch (i) {
case 0:
b = false;
Console.WriteLine("When integer is 0, boolean is:");
Console.WriteLine(b);
break;
case 1:
b = true;
Console.WriteLine("When integer is 1, boolean is:");
Console.WriteLine(b);
break;
}
switch (b) {
case true:
i = 1;
Console.WriteLine("When boolean is true, integer is:");
Console.WriteLine(i);
break;
case false:
i = 0;
Console.WriteLine("When boolean is false, integer is:");
Console.WriteLine(i);
break;
}
}
}
}
出力:
When an integer is 1, boolean is:
True
When boolean is true, integer is:
1
C#
で三元条件演算子を使用してブール値を整数に変換する
条件演算子 ?:
は、3 項条件演算子とも呼ばれ、if
ステートメントに似ています。ブール式を評価し、2つの式のうちの 1つの結果を返します。
ブール式が true の場合、最初のステートメントが返されます(つまり、?
の後のステートメント)。2 番目のステートメントが返されます(つまり、:
の後のステートメント)。詳細については、このリファレンスを参照してください。
以下はコードの例です。
// C# program to illustrate the
// use of ternary operator
using System;
class Test {
// Main Method
static public void Main() {
bool boolinput = true;
int intRresult = boolinput ? 1 : 0;
bool boolRresult = (intRresult == 1) ? true : false;
Console.Write("When Boolean is True, the converted integer value is: ");
Console.WriteLine(intRresult);
Console.Write("When integer is 1, the converted boolean value is: ");
Console.WriteLine(boolRresult);
}
}
出力:
When Boolean is True, the converted integer value is: 1
When integer is 1, the converted boolean value is: True
C#
で if
ステートメントを使用してブール値から整数に変換する
if
ステートメントは、論理式を実行した後、特定の条件が真であるか偽であるかをチェックします。式が true
を返すときはいつでも、数値 1 が返されます 0 が返されます。
同様に、数値が 1 の場合、ブール値 true
が返されます。それ以外の場合は、false
が返されます。
以下はコードの例です。
// C# program to illustrate the
// use of if statement
using System;
class Test {
// Main Method
static public void Main() {
bool boolinput = true;
int intResult;
if (boolinput) {
intResult = 1;
} else {
intResult = 0;
}
bool boolResult;
if (intResult == 1) {
boolResult = true;
} else {
boolResult = false;
}
Console.Write("When Boolean is True, the converted integer value is: ");
Console.WriteLine(intResult);
Console.Write("When integer is 1, the converted boolean value is: ");
Console.WriteLine(boolResult);
}
}
出力:
When Boolean is True, the converted integer value is: 1
When integer is 1, the converted boolean value is: True