C# でコンソール ウィンドウをクリアする
Aimen Fatima
2023年10月12日
Csharp
Csharp Console
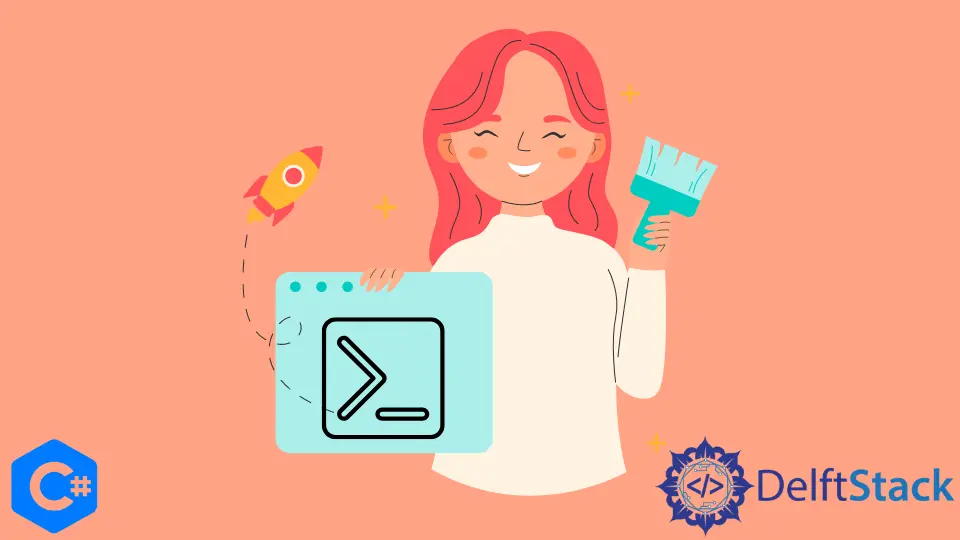
C# プロジェクトでコンソールをクリアする方法を示します。 概念をよりよく理解するためのコード スニペットを提供します。
Clear()
メソッドを使用して、C#
でコンソール ウィンドウをクリアする
実行中にコンソール ウィンドウをクリアする必要がある場合があります。 C# の Console
クラスは Clear()
メソッドを提供します。
このメソッドの定義は次のとおりです。
public static void Clear();
このメソッドは静的であるため、名前で呼び出すことができます。 I/O エラーが発生した場合、このメソッドは IOException をスローします。
Clear()
メソッドは、コンソール ウィンドウのすべてのコンテンツを削除し、画面とコンソール バッファーをクリアします。
Clear()
メソッドを表すサンプル プロジェクトを作成してみましょう。 この例では、for
ループを作成して 10 回反復し、ユーザーに任意のキーを入力するように求めます。
ユーザーがキーボードから任意のキーを押すと、Console.Clear()
が呼び出され、画面がクリアされ、2 番目の for
ループが実行されます。
プログラム 1:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ClearConsole {
class Program {
static void Main(string[] args) {
for (int i = 0; i < 10; i++) {
Console.WriteLine("count: " + i);
}
Console.WriteLine("Note: We are going to make the screen clear. Press any key to continue.");
Console.ReadKey();
Console.Clear();
for (int i = 10; i < 20; i++) {
Console.WriteLine("count: " + i);
}
Console.ReadKey();
}
}
}
出力:
count: 0
count: 1
count: 2
count: 3
count: 4
count: 5
count: 6
count: 7
count: 8
count: 9
Note: We are going to make the screen clear. Press any key to continue.
キーボードから任意のキーを押すと、画面がクリアになり、コンソールに次のような出力が表示されます。
count: 10
count: 11
count: 12
count: 13
count: 14
count: 15
count: 16
count: 17
count: 18
count: 19
以下は、Clear()
メソッドを示す別のプログラム例です。 このコード スニペットは、コンソール ウィンドウにテキストを表示し、Console.Clear()
が呼び出され、1 秒後にコンソールがクリアされます。
一部のテキストは、後でコンソール ウィンドウに表示されます。
プログラム 2:
using System;
using System.Collections.Generic;
using System.Linq;
using System.Text;
using System.Threading.Tasks;
namespace ClearConsole {
class Program {
static void Main(string[] args) {
string welcome = "Hi, Welcome! \nI am ClearConsole. What's your name?";
foreach (char c in welcome) {
Console.Write(c);
System.Threading.Thread.Sleep(50);
}
Console.WriteLine();
string name = Console.ReadLine();
string thanks = "Nice to see you " + name;
Console.WriteLine();
foreach (char c in thanks) {
Console.Write(c);
System.Threading.Thread.Sleep(50);
}
string note = "You are going to experience Clear() method.";
Console.WriteLine();
foreach (char c in note) {
Console.Write(c);
System.Threading.Thread.Sleep(50);
}
System.Threading.Thread.Sleep(1000);
Console.Clear();
string cleared = "Cleared the screen. \nHope you enjoyed this method. \nGood Bye!";
foreach (char c in cleared) {
Console.Write(c);
System.Threading.Thread.Sleep(50);
}
Console.ReadKey();
}
}
}
出力:
Hi, Welcome!
I am ClearConsole. What's your name?
human
Nice to see you human
You are going to experience Clear() method.
まず、このテキストがコンソールの出力として表示されます。 1 秒後、コンソールはクリアされ、その後の結果は次のようになります。
Cleared the screen.
Hope you enjoyed this method.
Good Bye!
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe