C++ で文字列をトークン化する
胡金庫
2023年10月12日
-
C++ で文字列をトークン化するには
find
関数とsubstr
関数を使用する -
関数
std::stringstream
とgetline
を用いて C++ で文字列をトークン化する -
C++ で文字列をトークン化するには、
istringstream
とcopy
アルゴリズムを利用する
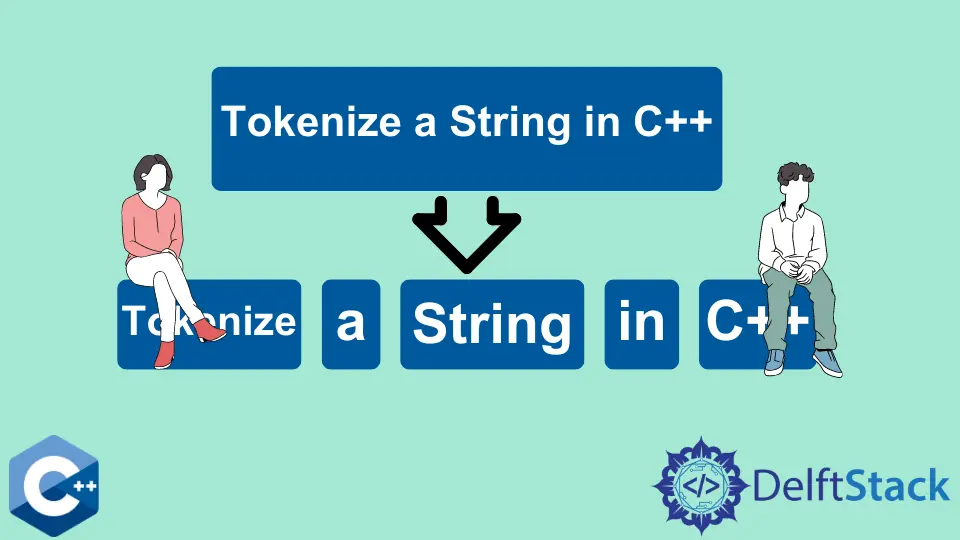
この記事では、C++ で文字列をトークン化する方法のいくつかの方法を説明します。
C++ で文字列をトークン化するには find
関数と substr
関数を使用する
std::string
クラスには、与えられた文字列オブジェクト内の文字列を検索するための find
関数が組み込まれています。関数 find
は文字列の最初の文字の位置を返し、見つからなければ npos
を返します。関数 find
の呼び出しは if
文の中に挿入され、最後の文字列トークンが抽出されるまで文字列の繰り返し処理を行います。
ユーザは任意の string
型の区切り文字を指定して find
メソッドに渡すことができることに注意してください。トークンは文字列の vector
にプッシュされ、反復処理のたびに erase()
関数で処理済みの部分が削除されます。
#include <algorithm>
#include <iostream>
#include <iterator>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
string text = "Think you are escaping and run into yourself.";
int main() {
string delim = " ";
vector<string> words{};
size_t pos = 0;
while ((pos = text.find(delim)) != string::npos) {
words.push_back(text.substr(0, pos));
text.erase(0, pos + delim.length());
}
if (!text.empty()) words.push_back(text.substr(0, pos));
for (const auto &str : words) {
cout << str << endl;
}
return EXIT_SUCCESS;
}
出力:
Think
you
are
escaping
and
run
into
yourself.
関数 std::stringstream
と getline
を用いて C++ で文字列をトークン化する
stringstream
を利用して処理対象の文字列を取得し、getline
を用いて与えられた区切り文字が見つかるまでトークンを抽出することができます。このメソッドは 1 文字のデリミタでのみ動作することに注意してください。
#include <algorithm>
#include <iostream>
#include <iterator>
#include <sstream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::istringstream;
using std::string;
using std::stringstream;
using std::vector;
int main() {
string text = "Think you are escaping and run into yourself.";
char del = ' ';
vector<string> words{};
stringstream sstream(text);
string word;
while (std::getline(sstream, word, del)) words.push_back(word);
for (const auto &str : words) {
cout << str << endl;
}
return EXIT_SUCCESS;
}
出力:
Think
you
are
escaping
and
run
into
yourself.
C++ で文字列をトークン化するには、istringstream
と copy
アルゴリズムを利用する
あるいは、<アルゴリズム>
ヘッダの copy
関数を利用して、空白区切りで文字列トークンを抽出することもできます。以下の例では、トークンを繰り返し処理して標準出力にストリームするだけです。copy
メソッドで文字列を処理するには、istringstream
に文字列を挿入し、そのイテレータを利用します。
#include <algorithm>
#include <iostream>
#include <iterator>
#include <sstream>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::istringstream;
using std::string;
using std::stringstream;
using std::vector;
int main() {
string text = "Think you are escaping and run into yourself.";
string delim = " ";
vector<string> words{};
istringstream iss(text);
copy(std::istream_iterator<string>(iss), std::istream_iterator<string>(),
std::ostream_iterator<string>(cout, "\n"));
return EXIT_SUCCESS;
}
出力:
Think
you
are
escaping
and
run
into
yourself.
著者: 胡金庫