C++ で std::stod 関数ファミリーを使用する
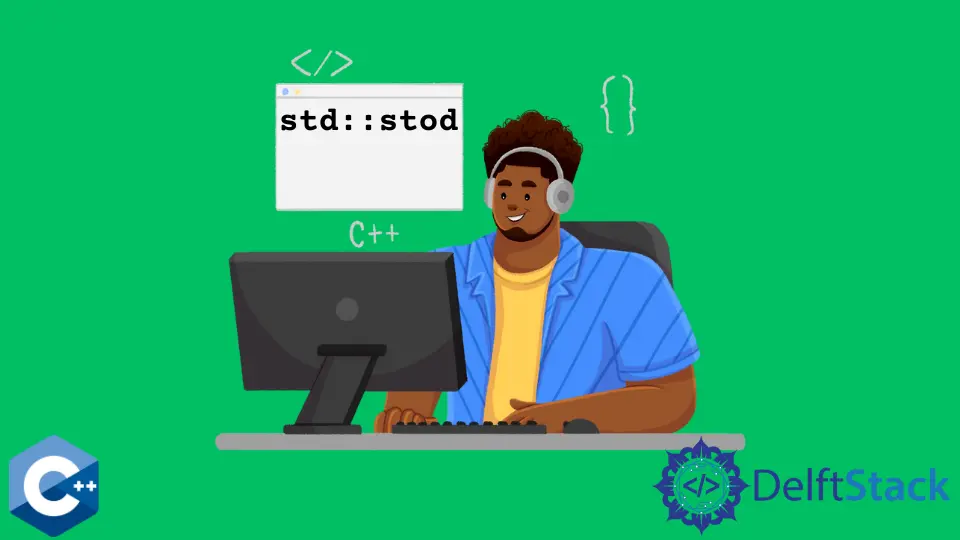
この記事では、C++ で std::stod
ファミリーの関数を使用する方法について説明し、説明します。
std::stod
を使用して、文字列
を C++ の浮動小数点値に変換する
std::stod
関数は std::stof
および std::stold
とともに STL によって提供され、文字列
から浮動小数点への変換を実行します。変換と言いますが、これは文字列の内容を浮動小数点値として解釈するようなものであることに注意してください。つまり、これらの関数は、事前定義された解析ルールを使用して指定された文字列
引数をスキャンし、有効な浮動小数点数を識別して、対応する型オブジェクトに格納します。
関数は、返されるタイプを示す接尾辞によって区別されます。std::stod
オブジェクトは double
値を返し、std::stof
は float を返し、std::stold
は long double
を返します。これらの関数は C++ 11 以降 STL の一部であり、<string>
ヘッダーファイルに含まれています。
以下のコードスニペットでは、さまざまな変換シナリオを調査し、これらの関数の解析ルールを掘り下げます。最初の例は、string
オブジェクトに数字と小数点記号(.
)のみが含まれている最も単純なケースです。std::stod
関数は、指定された文字シーケンスを有効な浮動小数点数として解釈し、それらを double
タイプに格納します。小数点は数値の一部とは見なされないため、コンマ(.
)文字にすることはできません。
#include <iostream>
#include <string>
using std::cout;
using std::endl;
using std::string;
int main() {
string str1 = "123.0";
string str2 = "0.123";
auto m1 = std::stod(str1);
auto m2 = std::stod(str2);
cout << "std::stod(\"" << str1 << "\") is " << m1 << endl;
cout << "std::stod(\"" << str2 << "\") is " << m2 << endl;
return EXIT_SUCCESS;
}
出力:
std::stod("123.0") is 123
std::stod("0.123") is 0.123
あるいは、数字が他の文字と混合されている文字列
オブジェクトがある場合、2つの一般的なケースを選び出すことができます。最初のシナリオ:string
オブジェクトは数字で始まり、その後に他の文字が続きます。std::stod
関数は、最初の非数字文字(小数点を除く)が検出される前に開始桁を抽出します。
2 番目のシナリオ:string
引数は数字以外の文字で始まります。この場合、関数は std::invalid_argument
例外をスローし、変換を実行できません。
#include <iostream>
#include <string>
using std::cout;
using std::endl;
using std::string;
int main() {
string str3 = "123.4 with chars";
string str4 = "chars 1.2";
auto m3 = std::stod(str3);
// auto m4 = std::stod(str4);
cout << "std::stod(\"" << str3 << "\") is " << m3 << endl;
return EXIT_SUCCESS;
}
出力:
std::stod("123.0") is 123
std::stod("0.123") is 0.123
通常、std::stod
とその関数ファミリーは、開始空白文字を破棄します。したがって、次の例に示すように、複数の先頭の空白文字とそれに続く数字を含む文字列を渡すと、変換は正常に実行されます。
さらに、これらの関数は、タイプ size_t*
のオプションの引数を取ることができます。これは、呼び出しが成功した場合に処理された文字数を格納します。文字列が有効な浮動小数点数に変換された場合、先頭の空白文字もカウントされることに注意してください。
#include <iostream>
#include <string>
using std::cout;
using std::endl;
using std::string;
int main() {
string str3 = "123.4 with chars";
string str5 = " 123.4";
size_t ptr1 = -1;
size_t ptr2 = -1;
auto m4 = std::stod(str3, &ptr1);
auto m5 = std::stod(str5, &ptr2);
cout << m4 << " - characters processed: " << ptr1 << endl;
cout << "std::stod(\"" << str5 << "\") is " << m5 << " - " << ptr2
<< " characters processed" << endl;
cout << "length: " << str5.size() << endl;
return EXIT_SUCCESS;
}
出力:
123.4 - characters processed: 5
std::stod(" 123.4") is 123.4 - 16 characters processed
length: 16