C++ で文字列を反復処理する
胡金庫
2023年10月12日
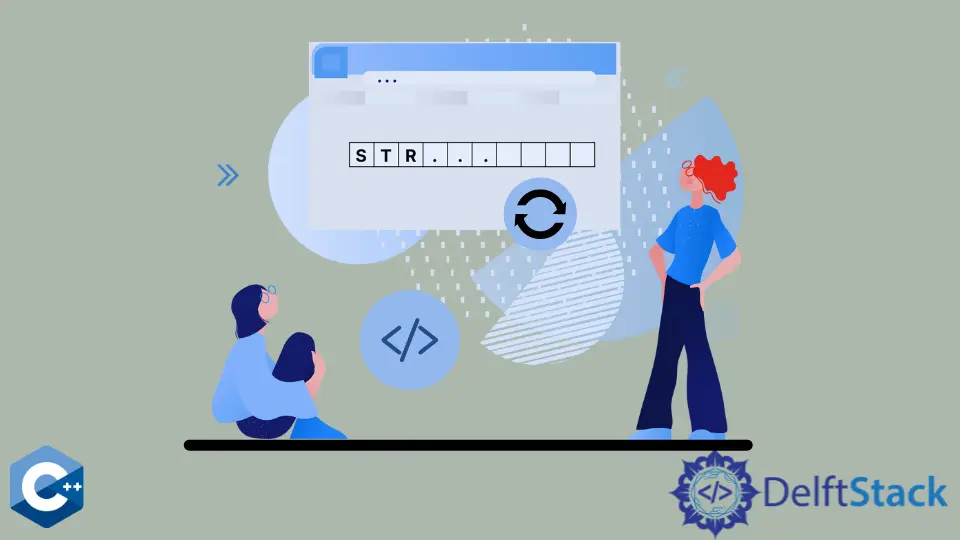
この記事では、C++ でインデックス数を保持しながら文字列を反復処理する方法について、複数の方法を紹介します。
C++ で範囲ベースのループを使用して文字列を反復処理する
現代の C++ 言語スタイルでは、それをサポートする構造体に対して範囲ベースの反復処理を推奨しています。一方、現在のインデックスは size_t
型の別の変数に格納することができ、これは反復のたびにインクリメントされます。インクリメントは変数の最後に ++
演算子で指定されることに注意してください。これをプレフィックスとして置くと、1 から始まるインデックスが得られるからです。以下の例は、プログラムの出力の短い部分のみを示しています。
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
string text = "They talk of days for which they sit and wait";
size_t index = 0;
for (char c : text) {
cout << index++ << " - '" << c << "'" << endl;
}
return EXIT_SUCCESS;
}
出力:
0 - 'T'
1 - 'h'
2 - 'e'
...
43 - 'i'
44 - 't'
C++ で文字列を反復処理するために for
ループを使用する
これは、従来の for
ループの中では優雅さとパワーを持っており、内部スコープに行列や多次元配列操作が含まれている場合に柔軟性を提供します。また、OpenMP 標準のような高度な並列化技術を使用する場合にも好まれる繰り返し構文です。
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
string text = "They talk of days for which they sit and wait";
for (int i = 0; i < text.length(); ++i) {
cout << i << " - '" << text[i] << "'" << endl;
}
return EXIT_SUCCESS;
}
出力:
0 - 'T'
1 - 'h'
2 - 'e'
...
43 - 'i'
44 - 't'
あるいは、メンバ関数 at()
を用いて文字列の個々の文字にアクセスすることもできます。
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl;
using std::string;
int main() {
string text = "They talk of days for which they sit and wait";
for (int i = 0; i < text.length(); ++i) {
cout << i << " - '" << text.at(i) << "'" << endl;
}
return EXIT_SUCCESS;
}
出力:
0 - 'T'
1 - 'h'
2 - 'e'
...
43 - 'i'
44 - 't'
著者: 胡金庫