C++ で文字列の中の部分文字列を検索する方法
胡金庫
2023年10月12日
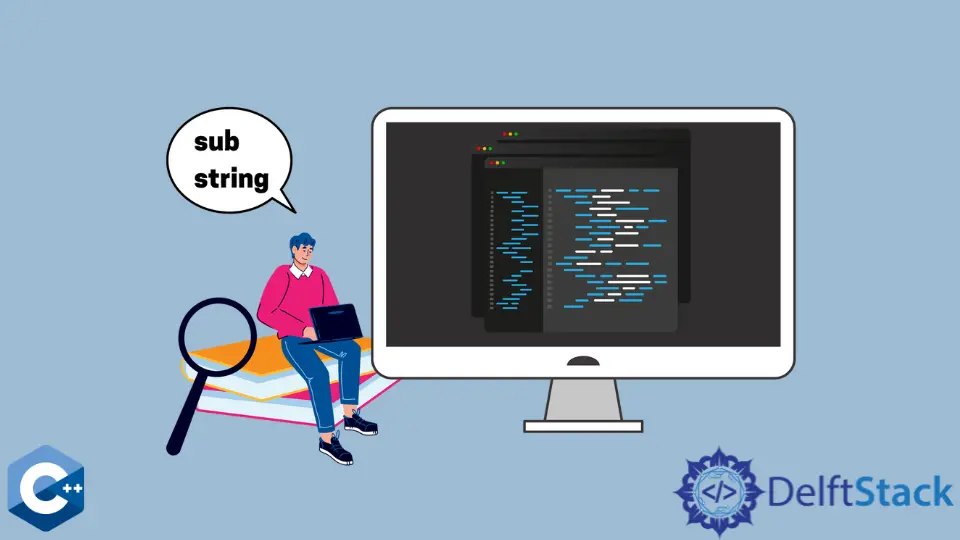
この記事では、C++ で文字列の中から指定された部分文字列を検索するメソッドを示します。
C++ で文字列の中の文字列を求めるには find
メソッドを使用する
問題を解決する最も簡単な方法は find
関数であり、これは組み込みの string
メソッドであり、別の string
オブジェクトを引数にとります。
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
int main() {
string str1 = "this is random string oiwao2j3";
string str2 = "oiwao2j3";
string str3 = "random s tring";
str1.find(str2) != string::npos
? cout << "str1 contains str2" << endl
: cout << "str1 does not contain str3" << endl;
str1.find(str3) != string::npos
? cout << "str1 contains str3" << endl
: cout << "str1 does not contain str3" << endl;
return EXIT_SUCCESS;
}
出力:
str1 contains str2
str1 does not contain str3
別の方法として、find
を使って 2つの文字列の特定の文字範囲を比較することもできます。これを行うには、範囲の開始位置と長さを引数として find
メソッドに渡す必要があります。
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
using std::stoi;
int main() {
string str1 = "this is random string oiwao2j3";
string str3 = "random s tring";
constexpr int length = 6;
constexpr int pos = 0;
str1.find(str3.c_str(), pos, length) != string::npos
? cout << length << " chars match from pos " << pos << endl
: cout << "no match!" << endl;
return EXIT_SUCCESS;
}
出力:
6 chars match from pos 0
C++ で文字列の中から部分文字列を探すには rfind
メソッドを用いる
rfind
メソッドは find
と同様の構造を持ちます。rfind
を利用して最後の部分文字列を見つけたり、与えられた部分文字列にマッチするように特定の範囲を指定したりすることができます。
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
using std::stoi;
int main() {
string str1 = "this is random string oiwao2j3";
string str2 = "oiwao2j3";
str1.rfind(str2) != string::npos
? cout << "last occurrence of str3 starts at pos " << str1.rfind(str2)
<< endl
: cout << "no match!" << endl;
return EXIT_SUCCESS;
}
出力:
last occurrence of str3 starts at pos 22
著者: 胡金庫