C++ でベクトルを配列に変換する方法
胡金庫
2023年10月12日
C++
C++ Vector
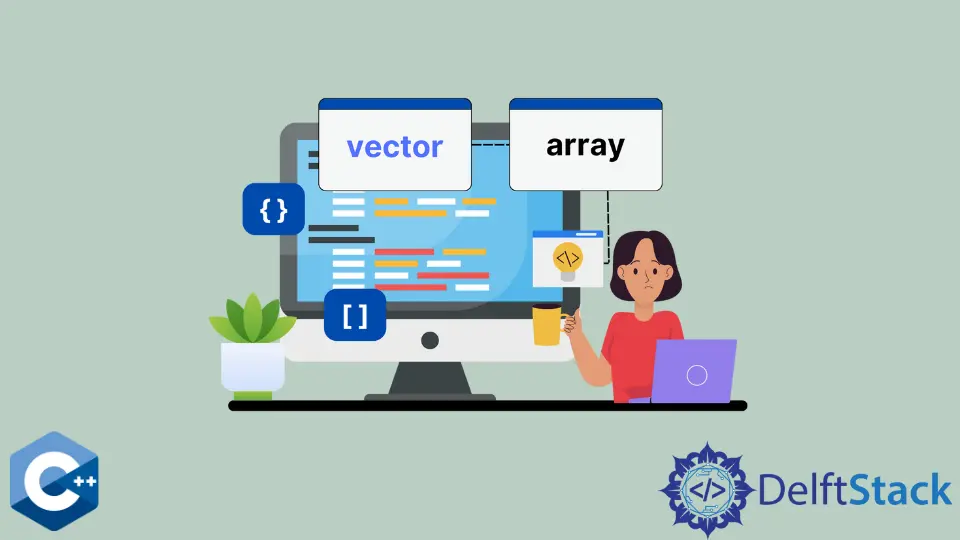
この記事では、C++ でベクトルを配列に変換する方法を紹介します。
data()
メソッドを使用して vector
を配列に変換する
C++ 標準では、vector
のコンテナ要素がメモリ内に連続して格納されることが保証されているので、組み込みのベクトルメソッドである data
を呼び出して、次のコードサンプルに示すように、新たに宣言された double
ポインタに戻り値のアドレスを代入することができます。
d_arr
ポインタを用いて要素を変更すると、元のベクトルのデータ要素が変更されることに注意してください。
#include <iostream>
#include <iterator>
#include <vector>
using std::cin;
using std::copy;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<double> arr{-3.5, -21.1, -1.99, 0.129, 2.5, 3.111};
copy(arr.begin(), arr.end(), std::ostream_iterator<double>(cout, "; "));
cout << endl;
double *d_arr = arr.data();
for (size_t i = 0; i < arr.size(); ++i) {
cout << d_arr[i] << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
出力:
-3.5; -21.1; -1.99; 0.129; 2.5; 3.111;
-3.5; -21.1; -1.99; 0.129; 2.5; 3.111;
演算子の &
アドレスを用いて vector
を配列に変換する
あるいは、メモリ内のオブジェクトのアドレスを取り、新たに宣言された double
へのポインタに代入するアンパサンド演算子を使用することもできます。
この例では、vector
の最初の要素のアドレスを取得していますが、他の要素へのポインタを抽出して必要に応じて演算を行うこともできます。
要素へのアクセスには d_arr[index]
配列記法を用いることができます。
#include <iostream>
#include <iterator>
#include <vector>
using std::cin;
using std::copy;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<double> arr{-3.5, -21.1, -1.99, 0.129, 2.5, 3.111};
copy(arr.begin(), arr.end(), std::ostream_iterator<double>(cout, "; "));
cout << endl;
double *d_arr = &arr[0];
for (size_t i = 0; i < arr.size(); ++i) {
cout << d_arr[i] << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
copy()
関数を使用して vector
を配列に変換する
copy()
メソッドを利用してベクトルを double
配列に変換し、データ要素を別のメモリにコピーすることができます。後で、元の vector
データを変更することを気にせずにデータを変更することができます。
ここでは、スタックメモリとして確保された d_arr
を 6 個の要素で固定して宣言していることに注意してください。ほとんどのシナリオでは、配列のサイズが事前にわからないので、new
や malloc
のようなメソッドを使って動的なメモリ割り当てを行う必要があります。
#include <iostream>
#include <iterator>
#include <vector>
using std::cin;
using std::copy;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<double> arr{-3.5, -21.1, -1.99, 0.129, 2.5, 3.111};
copy(arr.begin(), arr.end(), std::ostream_iterator<double>(cout, "; "));
cout << endl;
double d_arr[6];
copy(arr.begin(), arr.end(), d_arr);
for (size_t i = 0; i < arr.size(); ++i) {
cout << d_arr[i] << "; ";
}
cout << endl;
return EXIT_SUCCESS;
}
出力:
new_vec - | 97 | 98 | 99 | 100 | 101 |
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe
著者: 胡金庫