C++ で文字列を Char 配列に変換する方法
胡金庫
2023年10月12日
C++
C++ String
C++ Char
-
文字列を
Char
配列に変換するにはstd::basic_string::c_str
メソッドを使用する -
文字列を
Char
配列に変換するにはstd::vector
コンテナを使用する -
ポインタ操作を使って文字列を
Char
配列に変換する
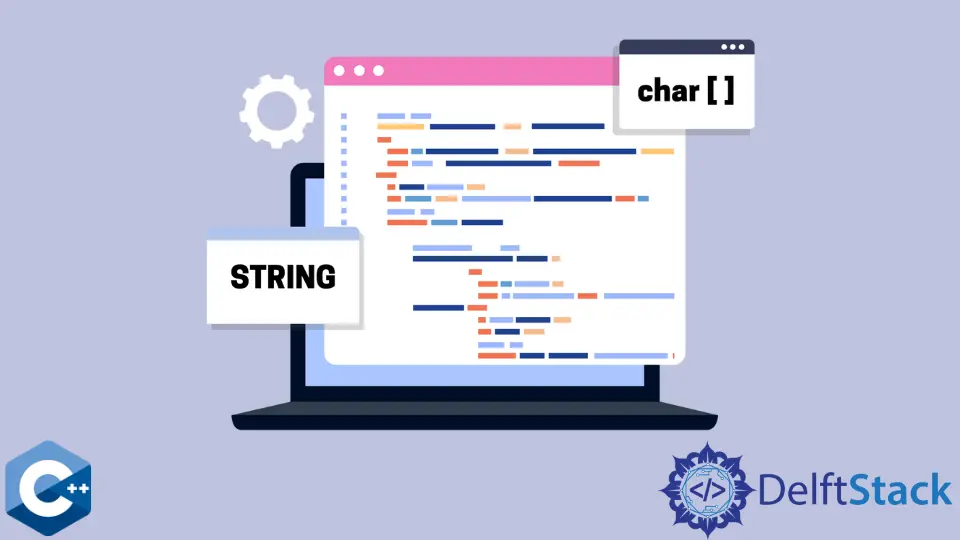
この記事では、文字列を Char
の配列に変換するための複数のメソッドを紹介します。
文字列を Char
配列に変換するには std::basic_string::c_str
メソッドを使用する
このバージョンは上記の問題を解決する C++ の方法です。これは string
クラスの組み込みメソッド c_str
を利用しており、ヌル文字で終端する文字配列へのポインタを返します。
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
int main() {
string tmp_string = "This will be converted to char*";
auto c_string = tmp_string.c_str();
cout << c_string << endl;
return EXIT_SUCCESS;
}
出力:
This will be converted to char*
c_str()
メソッドが返すポインタに格納されているデータを変更したい場合は、まず memove
関数を使ってその範囲を別の場所にコピーしてから、以下のように文字列を操作する必要があります。
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
int main() {
string tmp_string = "This will be converted to char*";
char *c_string_copy = new char[tmp_string.length() + 1];
memmove(c_string_copy, tmp_string.c_str(), tmp_string.length());
/* do operations on c_string_copy here */
cout << c_string_copy;
delete[] c_string_copy;
return EXIT_SUCCESS;
}
なお、c_string
のデータをコピーするには、以下のように様々な関数を利用することができます。ただし、マニュアルページを読み、それらのエッジケースやバグを注意深く考慮することに注意してください。
文字列を Char
配列に変換するには std::vector
コンテナを使用する
もう一つの方法は、string
の文字を vector
コンテナに格納し、その強力なビルトインメソッドを使ってデータを安全に操作することです。
#include <iostream>
#include <iterator>
#include <string>
#include <vector>
using std::cin;
using std::cout;
using std::endl;
using std::string;
using std::vector;
int main() {
string tmp_string = "This will be converted to char*\n";
vector<char> vec_str(tmp_string.begin(), tmp_string.end());
std::copy(vec_str.begin(), vec_str.end(),
std::ostream_iterator<char>(cout, ""));
return EXIT_SUCCESS;
}
ポインタ操作を使って文字列を Char
配列に変換する
このバージョンでは、char
ポインタを tmp_ptr
という名前で定義し、tmp_string
の最初の文字のアドレスをこれに代入しています。
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
int main() {
string tmp_string = "This will be converted to char*\n";
string mod_string = "I've been overwritten";
char *tmp_ptr = &tmp_string[0];
cout << tmp_ptr;
return EXIT_SUCCESS;
}
ただし、tmp_ptr
のデータを変更すると tmp_string
の値も変更されることに注意してください。
#include <cstring>
#include <iostream>
#include <string>
using std::cin;
using std::cout;
using std::endl using std::string;
int main() {
string tmp_string = "This will be converted to char*\n";
string mod_string = "I've been overwritten";
char *tmp_ptr = &tmp_string[0];
memmove(tmp_ptr, mod_string.c_str(), mod_string.length());
cout << tmp_string;
return EXIT_SUCCESS;
}
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe
著者: 胡金庫
関連記事 - C++ String
- C++ で最も長い共通部分文字列を見つける
- C++ で文字列の最初の文字を大文字にする
- C++ で文字列内の最初の繰り返し文字を見つける
- C++ での文字列と文字の比較
- C++ で文字列から最後の文字を削除する
- C++ の文字列から最後の文字を取得する