C++ で削除演算子を使用する
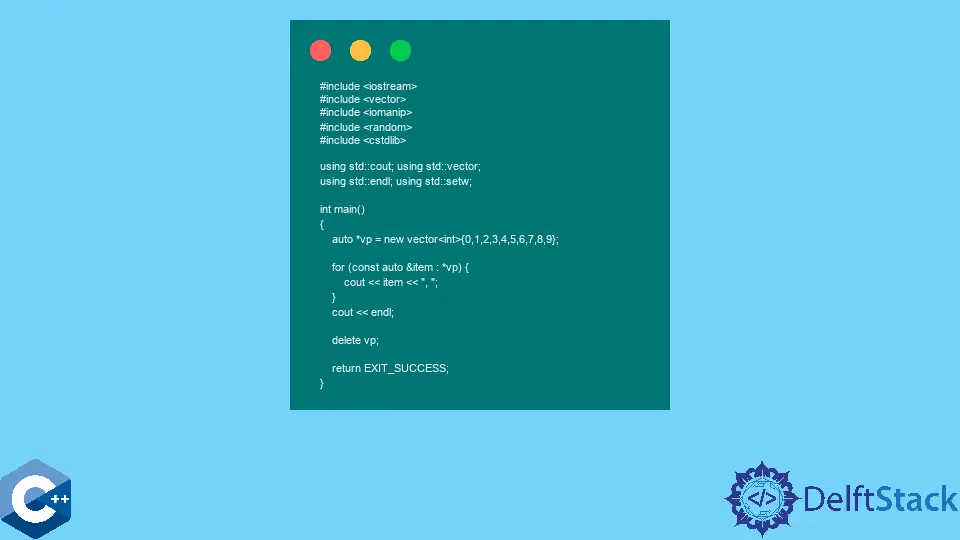
この記事では、C++ で delete
演算子を使用するいくつかの方法について説明します。
delete
演算子を使用して、オブジェクトに割り当てられたリソースを解放する
new
演算子を使用して動的に割り当てられたオブジェクトは、プログラムを終了する前に解放する必要があります。つまり、呼び出し元は、delete
操作を明示的に呼び出す必要があります。コンストラクタ初期化子を使用して、new
演算子でオブジェクトを宣言および初期化できることに注意してください。次のサンプルコードは、そのようなシナリオを示し、main
関数から戻る前に対応するリソースを解放します。ヒープオブジェクトで delete
演算子を呼び出さないと、メモリリークが発生し、長時間実行されるプロセスで大量のメモリが使用されることに注意してください。
#include <cstdlib>
#include <iomanip>
#include <iostream>
#include <random>
#include <vector>
using std::cout;
using std::endl;
using std::setw;
using std::string;
using std::vector;
int main() {
int *num = new int(1024);
auto *sp = new string(10, 'a');
cout << *num << endl;
cout << *sp << endl;
for (const auto &item : *vp) {
cout << item << ", ";
}
cout << endl;
delete num;
delete sp;
return EXIT_SUCCESS;
}
出力:
1024
aaaaaaaaaa
new
および delete
演算子は、標準ライブラリコンテナでも使用できます。つまり、次のサンプルコードでは、std::vector
オブジェクトが単一のステートメントで割り当てられ、初期化されます。new
演算子が vector
へのポインタを返すと、通常の vector
オブジェクトと同じように操作を実行できます。
#include <cstdlib>
#include <iomanip>
#include <iostream>
#include <random>
#include <vector>
using std::cout;
using std::endl;
using std::setw;
using std::vector;
int main() {
auto *vp = new vector<int>{0, 1, 2, 3, 4, 5, 6, 7, 8, 9};
for (const auto &item : *vp) {
cout << item << ", ";
}
cout << endl;
delete vp;
return EXIT_SUCCESS;
}
出力:
0, 1, 2, 3, 4, 5, 6, 7, 8, 9,
削除
演算子を使用して、ベクター内の要素を解放する
動的に割り当てられた配列で delete
演算子を使用する方法は少し異なります。通常、new
と delete
は一度に 1つずつオブジェクトを操作しますが、配列には 1 回の呼び出しで複数の要素を割り当てる必要があります。したがって、次のサンプルコードに示すように、角括弧表記を使用して配列内の要素の数を指定します。このような割り当ては、最初の要素へのポインターを返します。これは、さまざまなメンバーにアクセスするために逆参照できます。最後に、配列が不要な場合は、空の角括弧を含む特別な削除
表記を使用して配列を解放する必要があります。これにより、すべての要素の割り当てが解除されます。
#include <cstdlib>
#include <iomanip>
#include <iostream>
#include <random>
#include <vector>
using std::cout;
using std::endl;
using std::setw;
using std::vector;
constexpr int SIZE = 10;
constexpr int MIN = 1;
constexpr int MAX = 1000;
void initPrintIntVector(int *arr, const int &size) {
std::random_device rd;
std::default_random_engine eng(rd());
std::uniform_int_distribution<int> distr(MIN, MAX);
for (int i = 0; i < size; ++i) {
arr[i] = distr(eng) % MAX;
cout << setw(3) << arr[i] << "; ";
}
cout << endl;
}
int main() {
int *arr1 = new int[SIZE];
initPrintIntVector(arr1, SIZE);
delete[] arr1;
return EXIT_SUCCESS;
}
出力:
311; 468; 678; 688; 468; 214; 487; 619; 464; 734;
266; 320; 571; 231; 195; 873; 645; 981; 261; 243;