C のモジュロ演算子
胡金庫
2023年10月12日
-
C 言語で除算の残りを計算するために
%
モデューロ演算子を使用する -
モデューロ演算子
%
を用いてうるう年チェック関数を C で実装する -
C 言語で与えられた整数範囲内の乱数を生成するために
%
モデューロ演算子を使用する
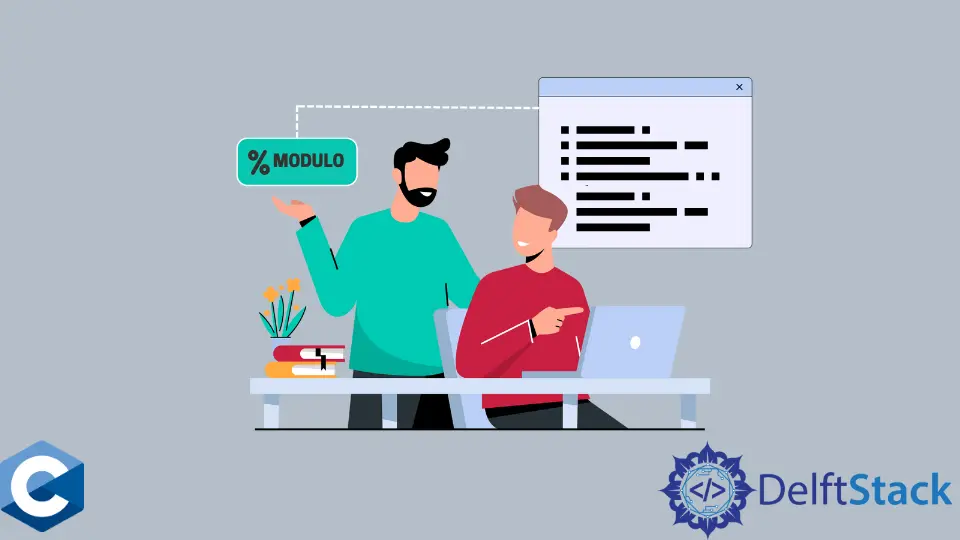
この記事では、C 言語で modulo 演算子を使用する方法の複数の方法を示します。
C 言語で除算の残りを計算するために %
モデューロ演算子を使用する
モデューロ %
は C 言語の二項演算子の一つです。与えられた 2つの数を除算した後の余りを生成します。モデューロ演算子は float
や double
のような浮動小数点数には適用できません。以下のコード例では、%
演算子を用いた最も簡単な例を示し、与えられた int
配列の modulus 9
の結果を表示します。
#include <stdio.h>
#include <stdlib.h>
int main(void) {
int arr[8] = {10, 24, 17, 35, 65, 89, 55, 77};
for (int i = 0; i < 8; ++i) {
printf("%d/%d yields the remainder of - %d\n", arr[i], 9, arr[i] % 9);
}
exit(EXIT_SUCCESS);
}
出力:
10/9 yields the remainder of - 1
24/9 yields the remainder of - 6
17/9 yields the remainder of - 8
35/9 yields the remainder of - 8
65/9 yields the remainder of - 2
89/9 yields the remainder of - 8
55/9 yields the remainder of - 1
77/9 yields the remainder of - 5
モデューロ演算子 %
を用いてうるう年チェック関数を C で実装する
あるいは、より複雑な関数を実装するために %
演算子を使用することもできます。次のコード例では、与えられた年がうるう年かどうかをチェックするブール関数 isLeapYear
を示します。年の値が 4 で割り切れるが 100 で割り切れない場合は閏年とみなされることに注意してください。さらに、年の値が 400 で割り切れる場合、うるう年とみなされます。
#include <stdbool.h>
#include <stdio.h>
#include <stdlib.h>
bool isLeapYear(int year) {
if ((year % 4 == 0 && year % 100 != 0) || year % 400 == 0)
return true;
else
return false;
}
int main(void) {
uint year = 2021;
isLeapYear(year) ? printf("%d is leap\n", year)
: printf("%d is not leap\n", year);
exit(EXIT_SUCCESS);
}
出力:
2021 is not leap
C 言語で与えられた整数範囲内の乱数を生成するために %
モデューロ演算子を使用する
モデューロ演算子のもう一つの有用な機能は、乱数生成処理中に数値の上階を制限することです。すなわち、乱数整数を生成する関数があるとします。その場合、返された数値と必要な値との除算の残りを最大値とすることができます(次の例では MAX
マクロとして定義されています)。乱数生成に srand
と rand
関数を使用するのはロバストな方法ではなく、質の高い乱数を必要とするアプリケーションでは他の機能を使用するべきであることに注意してください。
#include <stdio.h>
#include <stdlib.h>
#include <time.h>
#define COUNT 10
#define MAX 100
int main(void) {
srand(time(NULL));
for (int i = 0; i < COUNT; i++) {
printf("%d, ", rand() % MAX);
}
printf("\b\b \n");
exit(EXIT_SUCCESS);
}
出力:
3, 76, 74, 93, 51, 65, 76, 31, 61, 97
著者: 胡金庫