C の論理 XOR 演算子
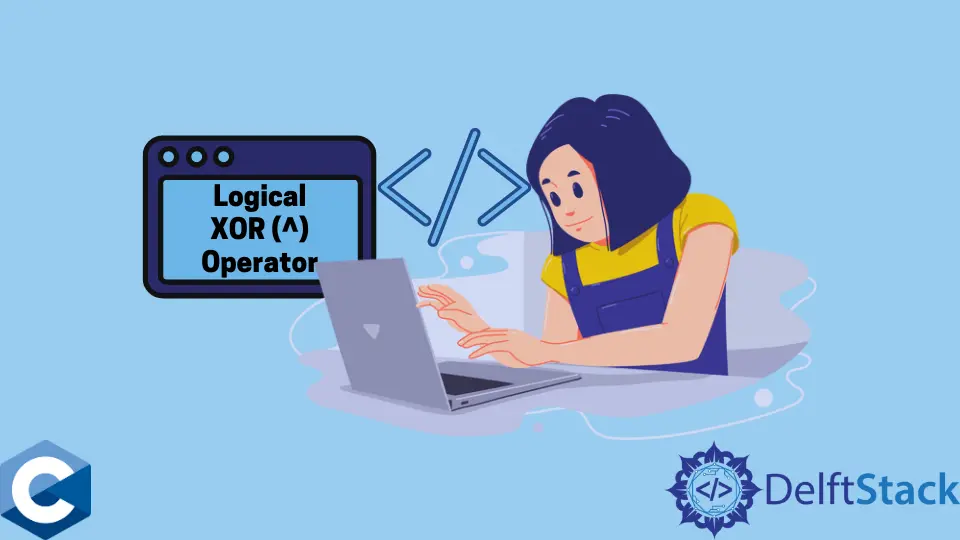
この記事では、C プログラミング言語の論理 XOR (^
) 演算子について理解します。
C の XOR (^
) 演算子の概要
XOR とも呼ばれる排他的 OR
は、オペランドのいずれかが真 (一方が真、もう一方が偽) の場合に真
の結果を返す論理演算子ですが、両方が真でも両方が真でもない場合は返されません。 間違い。 両方のオペランドが true の場合、論理条件の作成で基本的なor
を使用すると、混乱が生じる可能性があります。
そのような状況下では、何が基準を正確に満たしているのかを理解するのは難しいからです。 この問題は、修飾子 exclusive
を単語 or
に追加することで解決され、その意味も明確になりました。
^
を使用して C プログラミング言語で XOR を適用する
^
演算子は、ビットごとの XOR とも呼ばれ、オペランドとして 2つの整数を取り、両方の数値のすべてのビットに対して XOR を実行します。
両方のビットが異なる場合、XOR 演算は 1
の値を提供します。 ビットが同一の場合、値 0.
を返します。
C でビットごとの XOR を適用する例を見てみましょう。まず、ライブラリをインポートして main()
関数を作成します。
#include <stdio.h>
int main() {}
main
関数内で、firstValue
と secondValue
という名前の 2つの変数を int データ型で設定し、それぞれに整数値を設定します。 firstValue
は 0101,
に等しく、secondValue
はバイナリ形式で 1001
に等しくなります。
int firstValue = 5;
int secondValue = 9;
これら 2つの変数ができたので、それらに XOR を適用する必要があるため、applyXOR
という名前の新しい変数を作成し、これらの変数の両方で記号 ^
を使用します。
int applyXOR = firstValue ^ secondValue;
上記の式では、次のように両方のバイナリ値に対して XOR 演算が実行されます。
firstValue |
secondValue |
firstValue ^ secondValue |
---|---|---|
0 | 1 | 1 |
1 | 0 | 1 |
0 | 0 | 0 |
1 | 1 | 0 |
ここで、数値 1100
を 2 進形式で取得します。これを 10 進数に変換すると、値 12
と同じになります。 次に、取得した値を出力します。
printf("The value obtained after applying XOR is: %d", applyXOR);
C プログラミング言語で XOR を適用する
コード例:
#include <stdio.h>
int main() {
int firstValue = 5;
int secondValue = 9;
int applyXOR = firstValue ^ secondValue;
printf("The value obtained after applying XOR is: %d", applyXOR);
return 0;
}
出力:
The value obtained after applying XOR is: 12
XOR (^
) 演算子の動作を示す別の例を見てみましょう。
すべての項目が 1つの数字とは別に偶数回出現する数字のコレクションが与えられた場合、奇数の出現回数を見つけます。 この問題を解決するには、各整数に XOR(^
) 演算子を適用するだけで効果的です。
コード例:
#include <stdio.h>
int getOddOccurringNumber(int arr[], int number) {
int value = 0, i;
for (i = 0; i < number; i++) value ^= arr[i];
return value;
}
int main(void) {
int array[] = {12, 12, 14, 90, 14, 16, 16};
int sizeNumber = sizeof(array) / sizeof(array[0]);
printf("The value that occurred odd times in the list is: %d ",
getOddOccurringNumber(array, sizeNumber));
return 0;
}
出力:
The value that occurred odd times in the list is: 90
I am Waqar having 5+ years of software engineering experience. I have been in the industry as a javascript web and mobile developer for 3 years working with multiple frameworks such as nodejs, react js, react native, Ionic, and angular js. After which I Switched to flutter mobile development. I have 2 years of experience building android and ios apps with flutter. For the backend, I have experience with rest APIs, Aws, and firebase. I have also written articles related to problem-solving and best practices in C, C++, Javascript, C#, and power shell.
LinkedIn