C 言語でサブストリングを取得する
Satishkumar Bharadwaj
2023年10月12日
C
C Char
C String
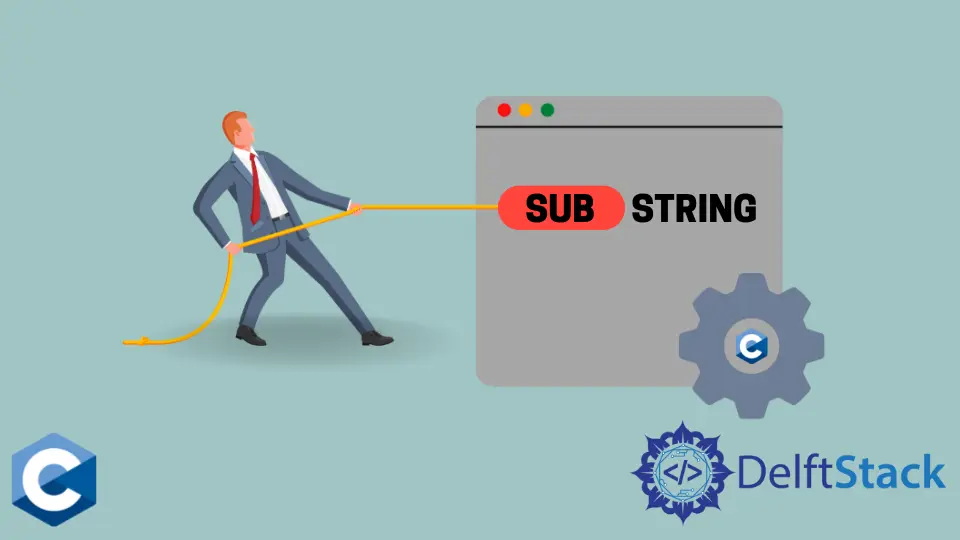
このチュートリアルでは、C 言語で文字の値から部分文字列を取得する方法を紹介します。文字から部分文字列を取得する方法には、memcpy()
や strncpy()
のような異なる方法があります。
C 言語で部分文字列を取得するための memcpy()
関数
関数 memcpy()
は文字数をコピー元からコピー先のメモリ領域にコピーします。この関数は <string.h>
ヘッダファイルで利用できます。
この関数は、コピー元とコピー先のアドレスが重複している場合に問題を生じる。この関数は NULL アドレスがあるかどうかやオーバーフローが発生しているかどうかはチェックしません。
関数 memcpy()
は出力先の文字列へのポインタを返します。エラーを表示するための戻り値はありません。
memcpy()
の構文
void *memcpy(void *destination_string, const void *source_string,
size_t number);
destination_string
は出力先の文字列へのポインタです。source_string
は元の文字型の配列へのポインタです。number
は文字数です。
#include <stdio.h>
#include <string.h>
int main(void) {
char *text = "The test string is here";
char subtext[7];
memcpy(subtext, &text[9], 6);
subtext[6] = '\0';
printf("The original string is: %s\n", text);
printf("Substring is: %s", subtext);
return 0;
}
出力:
The original string is: The test string is here
Substring is: string
C 言語で文字列を取得する strncpy()
関数
関数 strncpy()
は strcpy()
と同じです。唯一の違いは、strncpy()
関数が与えられた文字数を元の文字列から対象の文字列にコピーすることです。関数 strncpy()
は <string.h>
ヘッダファイルで利用可能です。
この関数は元の文字列をコピーした後、コピー先の文字列へのポインタを返します。
strncpy()
の構文
void *strncpy(void *destination_string, const void *source_string,
size_t number);
destination_string
は出力先の文字列へのポインタです。source_string
は元のchar
の値の配列へのポインタです。number
は文字数です。
関数 strncpy()
が source_string
にヌル文字を見つけた場合、この関数は destination_string
にヌル文字を追加します。
#include <stdio.h>
#include <string.h>
int main(void) {
char *text = "The test string is here";
char subtext[7];
strncpy(subtext, &text[9], 6);
subtext[6] = '\0';
printf("The original string is: %s\n", text);
printf("Substring is: %s", subtext);
return 0;
}
出力:
The original string is: The test string is here
Substring is: string
チュートリアルを楽しんでいますか? <a href="https://www.youtube.com/@delftstack/?sub_confirmation=1" style="color: #a94442; font-weight: bold; text-decoration: underline;">DelftStackをチャンネル登録</a> して、高品質な動画ガイドをさらに制作するためのサポートをお願いします。 Subscribe