C における前方宣言と構造体と Typedef 構造体の違い
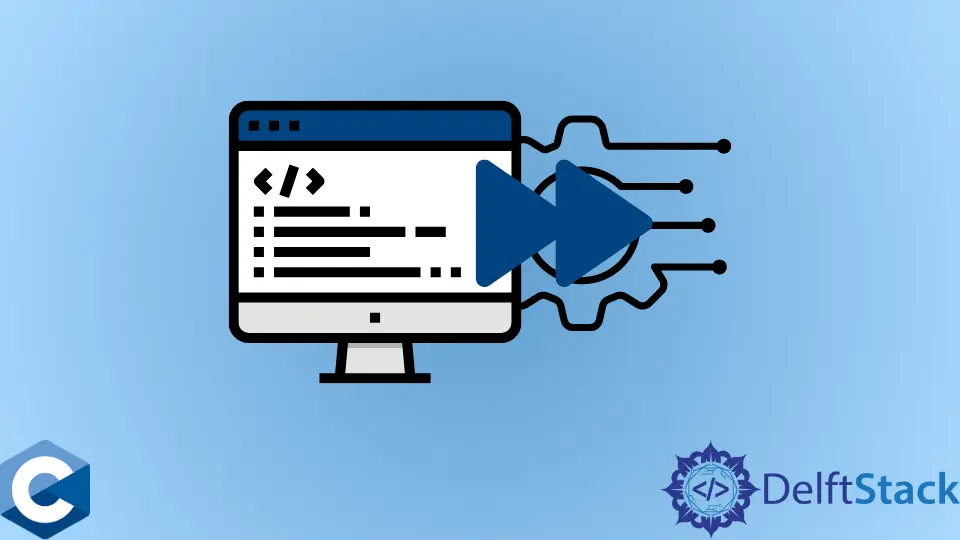
このチュートリアルでは、struct
の概念と typedef
キーワードの使用について説明します。 また、C における 前方宣言
の概念についても説明します。
Cプロジェクトを作成することから始めましょう。
C プロジェクトを作成する
-
最初のステップは、コンパイラをインストールすることです。 Cコンパイラをダウンロードしてインストールする手順.
-
次のステップでは、メニューバーの
ファイル
をクリックして、C 言語で空のプロジェクトを作成します。 -
コンパイルする前にファイルを保存します。
-
コードを実行します。
コンパイルして実行
をクリックします。 -
実行画面が表示されます。
C言語の構造体
Struct は、関連する データ メンバー
グループを作成するために C 言語で使用されます。 構造体のメンバーは同じデータ型を持っておらず、グループには異なる変数が 1 か所に保持されています。
ご存知のように、その配列には同じ型のメンバーがありますが、構造体では、データ メンバーは int
、float
、および char
のように異なる型にすることができます。 次のコード スニペットでは、C 言語で構造体を作成する方法について説明します。
このコードでは、構造体名
とその 変数
を記述して、メイン関数
の ローカル スコープ
で変数を宣言しています。
コード例:
#include <stdio.h>
struct Student { // Structure declaration
int rollno; // Member (int variable)
char gender; // Member (char variable)
}; // End the structure with a semicolon
int main() {
struct Student s1; // Making a variable of a struct
s1.rollno = 3; // assigning value to every member using dot operator
s1.gender = 'M';
printf("The name and gender of a student is %d and %c", s1.rollno, s1.gender);
}
出力:
The name and gender of a student is 3 and M
次のコードでは、変数をグローバル スコープで宣言します。
コード例:
#include <stdio.h>
struct Student { // Structure declaration
int rollno; // Member (int variable)
char gender; // Member (char variable)
} s1; // Declared a variable s1 before ending the struct with a semicolon
int main() {
s1.rollno = 3; // calling a member using a variable
s1.gender = 'M';
printf("The name and gender of a student is %d and %c", s1.rollno, s1.gender);
}
出力:
The name and gender of a student is 3 and M
これでも同じ出力が得られますが、上記のコード スニペットでは、変数 s1
をグローバルに宣言しています。 main()
関数で構造体を定義する必要はありません。
Cのtypedef
typedef
は C 言語で使用され、既存のデータ型の名前を新しいものに変更します。
構文:
typedef<existing_name><alias_name>
最初に、typedef
の 予約語
を記述し、次に C 言語で既存の名前、および割り当てたい名前を記述します。 typedef
を使用した後、プログラム全体で alias_name
を使用します。
コード:
#include <stdio.h>
void main() {
typedef int integer; // assigning int a new name of the integer
integer a = 3; // variable is declared by integer
integer b = 4;
printf("The values of a and b are %d and %d", a,
b); // print value of a and b on the screen
}
出力:
The values of a and b are 3 and 4
C の typedef 構造体
main()
関数に全体の構造体定義を書かなければならないことがわかりました。 毎回 struct student
を書く代わりに、typedef を使用して古い型を新しい型に置き換えることができます。
Typedef は、C 言語で型を作成するのに役立ちます。
コード例:
#include <stdio.h> // including header file of input/output
#include <string.h> // including header file of string
typedef struct Books { // old type
char title[30]; // data members of the struct book
char author[30];
char subject[50];
int id;
} Book; // new type
void main() {
Book b1; // variable of a new type
strcpy(b1.title, "C Programming"); // copy string in title
strcpy(b1.author, "Robert Lafore"); // copy string in author
strcpy(b1.subject, "Typedef Struct in C"); // copy string in subject
b1.id = 564555; // assigning id to variable
printf("Book title is : %s\n", b1.title); // printing book title on screen
printf("Book author is : %s\n", b1.author);
printf("Book subject is : %s\n", b1.subject);
printf("Book id is : %d\n", b1.id);
}
出力:
Book title is : C Programming
Book author is : Robert Lafore
Book subject is : Typedef Struct in C
Book id is : 564555
上記のコードでは、セミコロンの前の構造体の末尾に新しい構造体型を定義しました。 次に、その新しい型を main()
関数で使用しました。
この typedef
により、main()
関数で毎回 struct を定義して変数を作成する手間が軽減されました。
C の前方宣言
前方宣言は、構造体の実際の定義に先行する宣言です。 定義は利用できませんが、事前宣言である前方宣言により、宣言された型を参照できます。
このメソッドは、関数の定義と宣言に使用されます。 main()
関数の上に関数を定義する代わりに、それを上で宣言し、下で定義することができます。これは前方宣言と呼ばれます。
コード例:
#include <stdio.h>
int add(int x, int y); // (prototype)function declaration
void main() {
int n1, n2, sum;
scanf("%d %d", &n1, &n2); // taking numbers from user
sum = add(n1, n2); // call to the add function
printf("The sum of n1 and n2 is %d ", sum); // display sum on the screen
}
int add(int x, int y) // function definition
{
int result;
result = x + y;
return result; // returning the result to the main function
}
上記のコード スニペットは、main()
関数の先頭で関数プロトタイプを宣言したことを示しています。 定義する前に main で add()
関数を呼び出しますが、プロトタイプが既に定義されているため、正常に動作します。
これは前方宣言によるものです。
出力:
4 5
The sum of n1 and n2 is 9