AngularJS でシンプルなテーブルを作成する
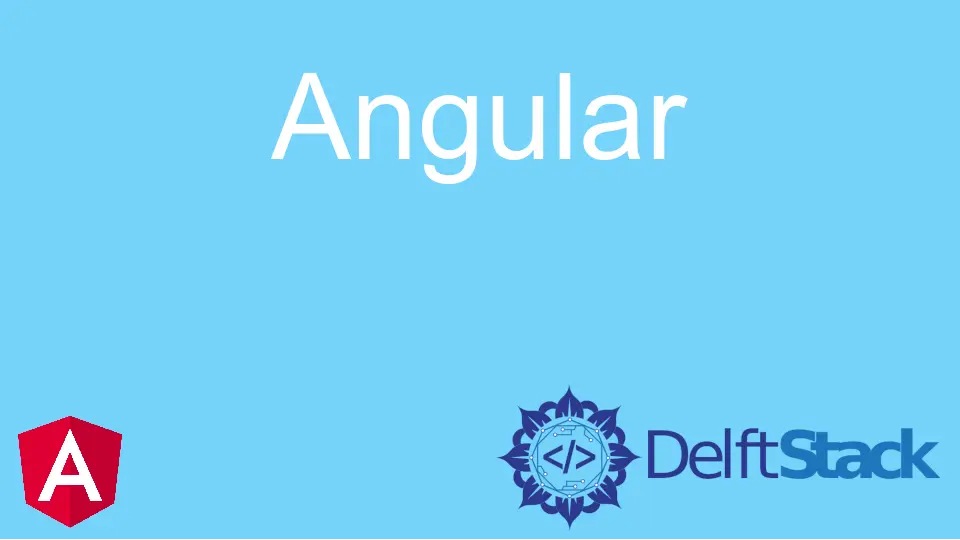
e コマース Web サイトで Web ページを作成する場合、テーブルを使用して、製品のリストとその価格、スタッフのリスト、給与、雇用日などの大規模で複雑な情報を表示する必要があります。
表は、これらのデータを適切に構造化され、消化しやすい方法で提示するのに役立ちます。それはまた私達が私達のウェブサイトのスクリーンの財産をよりよく管理することを可能にします。
したがって、*ngFor
関数を使用して単純なテーブルを作成し、それをデータベースに渡します。JSON Server Rest API
を利用します。
まず、VS Code Editor を使用します。これにより、内部からターミナルを使用して依存関係をインストールできます。次に、ターミナルを開いて npm install -g json-server
と入力し、json server
をシステムにインストールします。
次のステップは、新しいプロジェクトを作成することです。
プロジェクトが作成されたら、プロジェクトフォルダーを開き、db.json
という新しいファイルを追加します。これは、REST API
がアクセスするデータを保存する場所です。
次に、db.json
フォルダーに移動し、テーブルに必要なデータを入力します。
{
"Users": [
{
"id": 1,
"firstName": "Nitin",
"lastName": "Rana",
"email": "nitin.rana@gmail.com",
"mobile": "2345678901",
"salary": "25000"
},
{
"id": 2,
"firstName": "Rajat",
"lastName": "Singh",
"email": "rajat.singh1@gmail.com",
"mobile": "5637189302",
"salary": "30000"
},
{
"id": 3,
"firstName": "Rahul",
"lastName": "Singh",
"email": "rahul.singh1@gmail.com",
"mobile": "5557189302",
"salary": "40000"
},
{
"id": 4,
"firstName": "Akhil",
"lastName": "Verma",
"email": "akhil.verma2@gmail.com",
"mobile": "5690889302",
"salary": "20000"
},
{
"id": 5,
"firstName": "Mohan",
"lastName": "Ram",
"email": "mohan.ram1@gmail.com",
"mobile": "7637189302",
"salary": "60000"
},
{
"id": 6,
"firstName": "Sohan",
"lastName": "Rana",
"email": "sohan.rana@gmail.com",
"mobile": "3425167890",
"salary": "25000"
},
{
"id": 7,
"firstName": "Rajjev",
"lastName": "Singh",
"email": "rajeev.singh1@gmail.com",
"mobile": "5637189302",
"salary": "30000"
},
{
"id": 8,
"firstName": "Mukul",
"lastName": "Singh",
"email": "mukul.singh1@gmail.com",
"mobile": "5557189302",
"salary": "40000"
},
{
"id": 9,
"firstName": "Vivek",
"lastName": "Verma",
"email": "vivek.verma2@gmail.com",
"mobile": "5690889302",
"salary": "20000"
},
{
"id": 10,
"firstName": "Shubham",
"lastName": "Singh",
"email": "shubham.singh@gmail.com",
"mobile": "7637189502",
"salary": "60000"
}
]
}
次に行うことは、app.component.html
をナビゲートして、*ngFor
関数を使用してテーブルを作成することです。ただし、その前に、db.json
からのデータにアクセスする Rest サービス
を作成する必要があります。
次に、ターミナルに向かいます。プロジェクトフォルダに、ng generate service Rest
と入力します。
インストール後、scr/app
フォルダーに rest.service.spec.ts
と rest.service.ts
の 2つのファイルが作成されます。次に、コーディングを行うために rest.service.ts
に移動する必要があります。
import { Injectable } from '@angular/core';
import { HttpClient } from '@angular/common/http';
import { Users } from './Users';
@Injectable({
providedIn: 'root'
})
export class RestService {
constructor(private http : HttpClient) { }
url : string = "http://localhost:3000/Users";
getUsers()
{
return this.http.get<Users[]>(this.url);
}
}
このサービスは、データベースが渡される目的の URL のアクティビティを監視するのに役立ちます。
次に、app.component.html
に移動し、*ngFor
関数を使用してテーブルを作成します。
<h1>Table using JSON Server API</h1>
<hr>
<table id="users">
<tr>
<th *ngFor="let col of columns">
{{col}}
</th>
</tr>
<tr *ngFor="let user of users">
<td *ngFor="let col of index">
{{user[col]}}
</td>
</tr>
</table>
次に行う必要があるのは、app.module.ts
にアクセスして、さらにコーディングを行うことです。ここでは、インポート配列に HttpClientModule
を追加します。
import { BrowserModule } from '@angular/platform-browser';
import { NgModule } from '@angular/core';
import { AppComponent } from './app.component';
import { HttpClientModule } from '@angular/common/http';
@NgModule({
declarations: [
AppComponent
],
imports: [
BrowserModule,
HttpClientModule
],
providers: [],
bootstrap: [AppComponent]
})
export class AppModule { }
db.json
のテーブルの日付を HttpClient
で読み取り可能にするには、文字列プロパティにする必要があります。そこで、Users.ts
ファイルを作成し、次のコードを入力します。
export class Users
{
id : string;
firstName : string;
lastName : string;
email : string;
mobile : string;
salary : string;
constructor(id, firstName, lastName, email, mobile, salary)
{
this.id = id;
this.firstName = firstName;
this.lastName = lastName;
this.email = email;
this.mobile = mobile;
this.salary = salary;
}
}
この時点で残されている唯一のことは、CSS スタイルを使用してテーブルを美化することです。
h1
{
text-align: center;
color: #4CAF50;
}
#users {
font-family: "Trebuchet MS", Arial, Helvetica, sans-serif;
border-collapse: collapse;
width: 100%;
}
#users td, #users th {
border: 1px solid #ddd;
padding: 8px;
}
#users tr:nth-child(even){background-color: #f2f2f2;}
#users tr:hover {background-color: #ddd;}
#users th {
padding-top: 12px;
padding-bottom: 12px;
text-align: left;
background-color: #4CAF50;
color: white;
}
これですべて正常に動作するはずですが、Parameter mobile
が暗黙的に any
タイプであるなどのエラーに遭遇する可能性があります。
ts.config.json
ファイルに移動し、noImplicitAny: false
をリストに追加する必要があります。次に、\\
を noImplicitOverride: true,
に追加して、設定を非アクティブにします。
Fisayo is a tech expert and enthusiast who loves to solve problems, seek new challenges and aim to spread the knowledge of what she has learned across the globe.
LinkedIn