PyQt5 Tutorial - Pulsante a pressione
-
Pulsante di comando in PyQt5 -
QPushButton
-
PyQt5
QLabel
Label Widget Set Style -
PyQt5
QLabel
Label Click Event
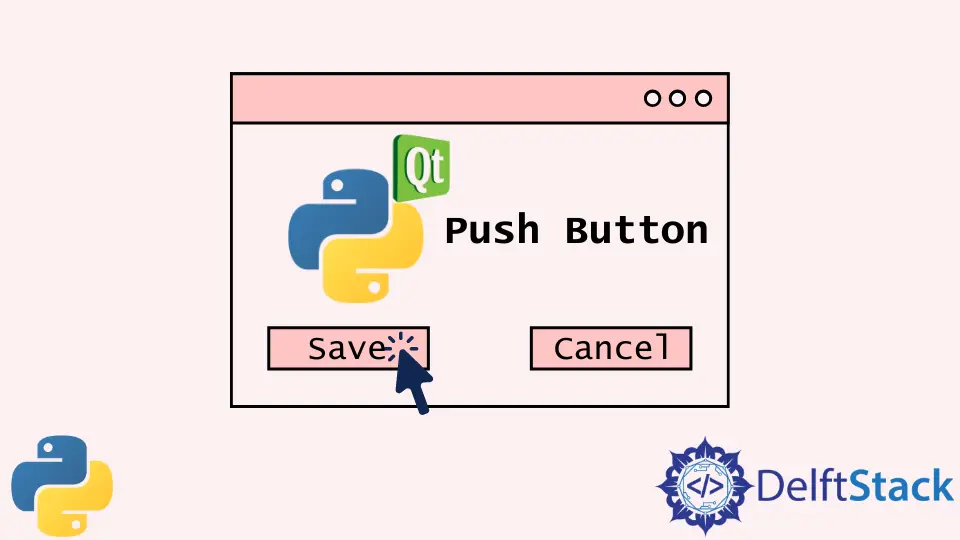
Push button widget QPushButton
è un pulsante di comando in PyQt5. Viene cliccato dall’utente per comandare al PC di eseguire alcune azioni specifiche, come Applica
, Annulla
e Salva
.
Pulsante di comando in PyQt5 - QPushButton
import sys
from PyQt5 import QtWidgets
def basicWindow():
app = QtWidgets.QApplication(sys.argv)
windowExample = QtWidgets.QWidget()
buttonA = QtWidgets.QPushButton(windowExample)
labelA = QtWidgets.QLabel(windowExample)
buttonA.setText("Click!")
labelA.setText("Show Label")
windowExample.setWindowTitle("Push Button Example")
buttonA.move(100, 50)
labelA.move(110, 100)
windowExample.setGeometry(100, 100, 300, 200)
windowExample.show()
sys.exit(app.exec_())
basicWindow()
Dove,
buttonA = QtWidgets.QPushButton(windowExample)
buttonA
è il QPushButton
di QtWidgets
e dovrebbe essere aggiunto alla finestra windowExample
come etichette introdotte negli ultimi capitoli.
buttonA.setText("Click!")
Imposta il testo di buttonA
come Click!
.
In realtà non servirà a niente.
PyQt5 QLabel
Label Widget Set Style
Lo stile del widget QLabel
di PyQt5 come il colore di sfondo, la famiglia di font e la dimensione del font può essere impostato con il metodo setStyleSheet
. Funziona come il foglio di stile in CSS.
buttonA.setStyleSheet(
"background-color: red;font-size:18px;font-family:Times New Roman;"
)
Imposta buttonA
con i seguenti stili,
Stile | Valore |
---|---|
Background-color |
red |
Font-size |
18px |
Font-family |
Times New Roman |
È conveniente impostare gli stili in PyQt5 perché è simile ai CSS.
import sys
from PyQt5 import QtWidgets
def basicWindow():
app = QtWidgets.QApplication(sys.argv)
windowExample = QtWidgets.QWidget()
buttonA = QtWidgets.QPushButton(windowExample)
labelA = QtWidgets.QLabel(windowExample)
buttonA.setStyleSheet(
"background-color: red;font-size:18px;font-family:Times New Roman;"
)
buttonA.setText("Click!")
labelA.setText("Show Label")
windowExample.setWindowTitle("Push Button Example")
buttonA.move(100, 50)
labelA.move(110, 100)
windowExample.setGeometry(100, 100, 300, 200)
windowExample.show()
sys.exit(app.exec_())
basicWindow()
PyQt5 QLabel
Label Click Event
L’evento click del pulsante è collegato ad una funzione specifica con il metodo QLabel.click.connect(func)
.
import sys
from PyQt5 import QtWidgets
class Test(QtWidgets.QMainWindow):
def __init__(self):
QtWidgets.QMainWindow.__init__(self)
self.buttonA = QtWidgets.QPushButton("Click!", self)
self.buttonA.clicked.connect(self.clickCallback)
self.buttonA.move(100, 50)
self.labelA = QtWidgets.QLabel(self)
self.labelA.move(110, 100)
self.setGeometry(100, 100, 300, 200)
def clickCallback(self):
self.labelA.setText("Button is clicked")
if __name__ == "__main__":
app = QtWidgets.QApplication(sys.argv)
test = Test()
test.show()
sys.exit(app.exec_())
Quando il QPushButton
buttonA
viene cliccato, esso attiva la funzione clickCallback
per impostare il testo dell’etichetta come Button is clicked
.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook