Scrivi byte su un file in Python
- Scrivi byte su un file in Python
- Scrivi un array di byte su un file in Python
-
Scrivi oggetti
BytesIO
in un file binario
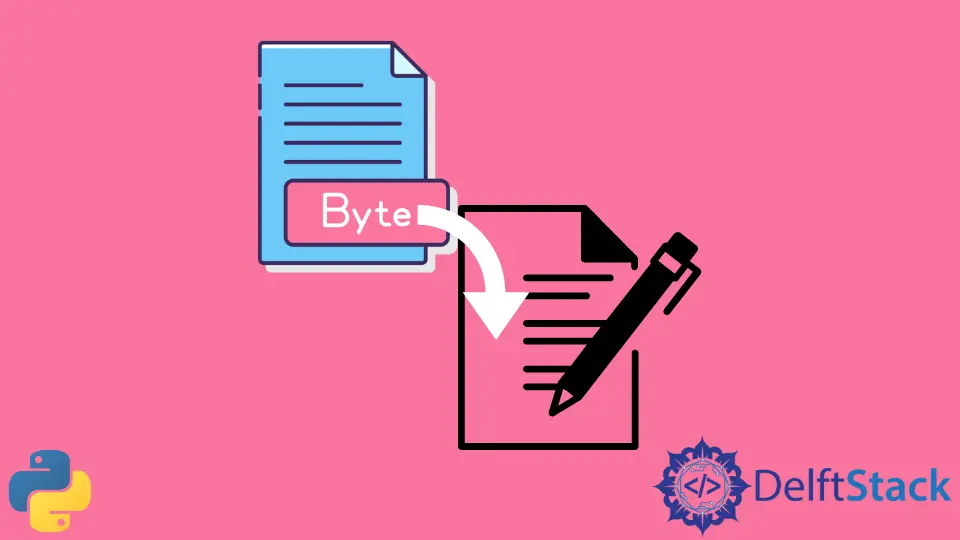
In questo tutorial, introdurremo come scrivere byte in un file binario in Python.
I file binari contengono stringhe di tipo byte. Quando leggiamo un file binario, viene restituito un oggetto di tipo byte. In Python, i byte sono rappresentati utilizzando cifre esadecimali. Sono preceduti dal carattere b
, che indica che sono byte.
Scrivi byte su un file in Python
Per scrivere byte in un file, creeremo prima un oggetto file utilizzando la funzione open()
e forniremo il percorso del file. Il file dovrebbe essere aperto in modalità wb
, che specifica la modalità di scrittura nei file binari. Il codice seguente mostra come scrivere byte in un file.
data = b"\xC3\xA9"
with open("test.bin", "wb") as f:
f.write(data)
Possiamo anche usare la modalità di aggiunta - a
quando abbiamo bisogno di aggiungere più dati alla fine del file esistente. Per esempio:
data = b"\xC3\xA9"
with open("test.bin", "ab") as f:
f.write(data)
Per scrivere byte in una posizione specifica, possiamo usare la funzione seek()
che specifica la posizione del puntatore del file per leggere e scrivere dati. Per esempio:
data = b"\xC3\xA9"
with open("test.bin", "wb") as f:
f.seek(2)
f.write(data)
Scrivi un array di byte su un file in Python
Possiamo creare un array di byte usando la funzione bytearray()
. Restituisce un oggetto mutabile bytearray
. Possiamo anche convertirlo in byte per renderlo immutabile. Nell’esempio seguente, scriviamo un array di byte in un file.
arr = bytearray([1, 2, 5])
b_arr = bytes(arr)
with open("test.bin", "wb") as f:
f.write(b_arr)
Scrivi oggetti BytesIO
in un file binario
Il modulo io
ci permette di estendere le funzioni e le classi di input-output relative alla gestione dei file. Viene utilizzato per memorizzare byte e dati in blocchi del buffer di memoria e ci consente anche di lavorare con i dati Unicode. Il metodo getbuffer()
della classe BytesIO
viene utilizzato qui per recuperare una vista di lettura e scrittura dell’oggetto. Vedere il codice seguente.
from io import BytesIO
bytesio_o = BytesIO(b"\xC3\xA9")
with open("test.bin", "wb") as f:
f.write(bytesio_o.getbuffer())
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedIn