Aggiungi una nuova riga a un file CSV in Python
-
Aggiungi i dati nella lista al file CSV in Python usando
writer.writerow()
-
Aggiungi i dati nel dizionario al file CSV in Python usando
DictWriter.writerow()
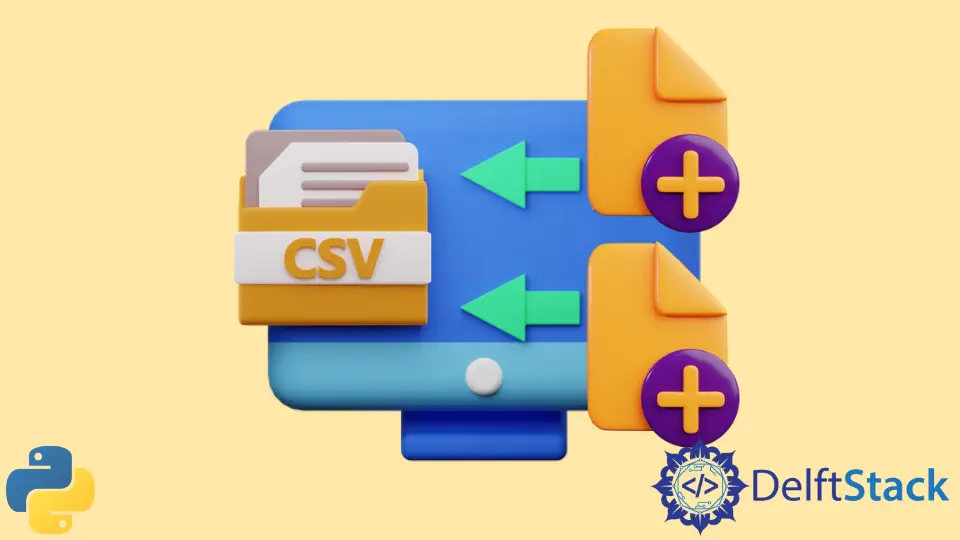
Se desideri aggiungere una nuova riga in un file CSV in Python, puoi utilizzare uno dei seguenti metodi.
- Assegnare i dati della riga desiderata in un elenco. Quindi, aggiungi i dati di questa lista al file CSV usando
writer.writerow()
. - Assegnare i dati della riga desiderata in un dizionario. Quindi, aggiungi i dati di questo dizionario al file CSV usando
DictWriter.writerow()
.
Aggiungi i dati nella lista al file CSV in Python usando writer.writerow()
In questo caso, prima di aggiungere la nuova riga al vecchio file CSV, dobbiamo assegnare i valori della riga a un elenco.
Per esempio,
list = ["4", "Alex Smith", "Science"]
Successivamente, passa questi dati dall’elenco come argomento alla funzione writerow()
dell’oggetto CSV writer()
.
Per esempio,
csvwriter_object.writerow(list)
Prerequisiti:
-
La classe CSV
writer
deve essere importata dal moduloCSV
.from csv import writer
-
Prima di eseguire il codice, il file CSV deve essere chiuso manualmente.
Esempio: aggiungi i dati nella lista al file CSV utilizzando writer.writerow()
Ecco un esempio del codice che mostra come si possono aggiungere i dati presenti in una Lista in un file CSV -
# Pre-requisite - Import the writer class from the csv module
from csv import writer
# The data assigned to the list
list_data = ["03", "Smith", "Science"]
# Pre-requisite - The CSV file should be manually closed before running this code.
# First, open the old CSV file in append mode, hence mentioned as 'a'
# Then, for the CSV file, create a file object
with open("CSVFILE.csv", "a", newline="") as f_object:
# Pass the CSV file object to the writer() function
writer_object = writer(f_object)
# Result - a writer object
# Pass the data in the list as an argument into the writerow() function
writer_object.writerow(list_data)
# Close the file object
f_object.close()
Supponiamo prima di eseguire il codice; il vecchio file CSV contiene il contenuto seguente.
ID,NAME,SUBJECT
01,Henry,Python
02,Alice,C++
Una volta eseguito il codice, il file CSV verrà modificato.
ID,NAME,SUBJECT
01,Henry,Python
02,Alice,C++
03,Smith,Science
Aggiungi i dati nel dizionario al file CSV in Python usando DictWriter.writerow()
In questo caso, prima di aggiungere la nuova riga al vecchio file CSV, assegna i valori della riga a un dizionario.
Per esempio,
dict = {"ID": 5, "NAME": "William", "SUBJECT": "Python"}
Successivamente, passa questi dati dal dizionario come argomento alla funzione writerow()
dell’oggetto DictWriter()
del dizionario.
Per esempio,
dictwriter_object.writerow(dict)
Prerequisiti:
-
La classe
DictWriter
deve essere importata dal moduloCSV
.from csv import DictWriter
-
Prima di eseguire il codice, il file CSV deve essere chiuso manualmente.
Esempio: aggiungi i dati nel dizionario al file CSV utilizzando DictWriter.writerow()
Ecco un esempio del codice che mostra come si possono aggiungere i dati presenti in un Dizionario in un file CSV.
# Pre-requisite - Import the DictWriter class from csv module
from csv import DictWriter
# The list of column names as mentioned in the CSV file
headersCSV = ["ID", "NAME", "SUBJECT"]
# The data assigned to the dictionary
dict = {"ID": "04", "NAME": "John", "SUBJECT": "Mathematics"}
# Pre-requisite - The CSV file should be manually closed before running this code.
# First, open the old CSV file in append mode, hence mentioned as 'a'
# Then, for the CSV file, create a file object
with open("CSVFILE.csv", "a", newline="") as f_object:
# Pass the CSV file object to the Dictwriter() function
# Result - a DictWriter object
dictwriter_object = DictWriter(f_object, fieldnames=headersCSV)
# Pass the data in the dictionary as an argument into the writerow() function
dictwriter_object.writerow(dict)
# Close the file object
f_object.close()
Supponiamo, prima di eseguire il codice, che il vecchio file CSV contenga il contenuto seguente.
ID,NAME,SUBJECT
01,Henry,Python
02,Alice,C++
03,Smith,Science
Una volta eseguito il codice, il file CSV verrà modificato.
ID,NAME,SUBJECT
01,Henry,Python
02,Alice,C++
03,Smith,Science
04,John,Mathematics