Come controllare se un file esiste in Python
-
try...except
per verificare l’esistenza del file (> Python 2.x) -
os.path.isfile()
per controllare se il file esiste (>=Python 2.x) -
pathlib.Path.is_file()
per verificare se il file esiste (>=Python 3.4)
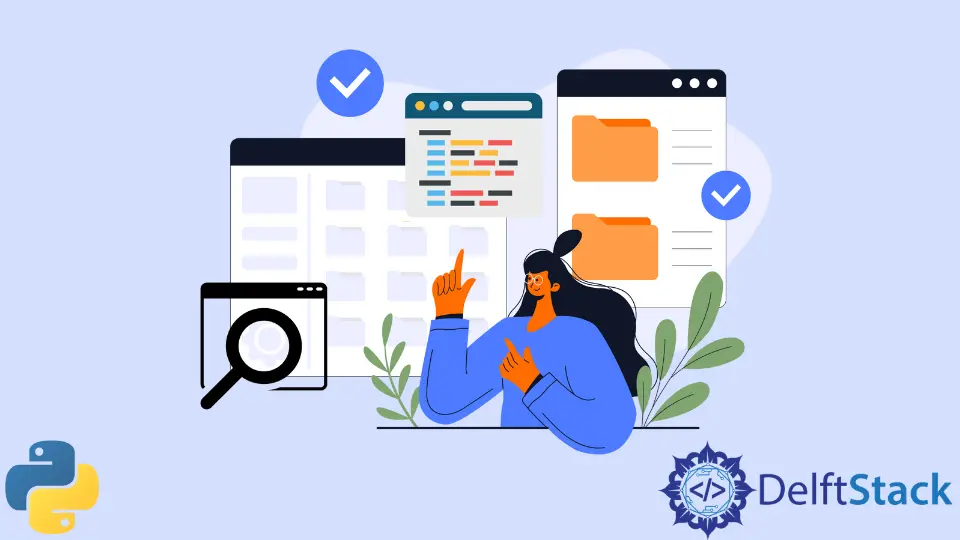
Questo tutorial introdurrà tre diverse soluzioni per verificare se un file esiste in Python.
try...except
blocco per verificare l’esistenza del file (>=Python 2.x)- funzione
os.path.isfile
per controllare l’esistenza del file (>=Python 2.x) pathlib.Path.is_file()
controlla l’esistenza del file (>=Python 3.4)
try...except
per verificare l’esistenza del file (> Python 2.x)
Potremmo provare ad aprire il file e controllare se il file esiste o meno a seconda che venga lanciato o meno l’IOError
(in Python 2.x) o FileNotFoundError
(in Python 3.x).
def checkFileExistance(filePath):
try:
with open(filePath, "r") as f:
return True
except FileNotFoundError as e:
return False
except IOError as e:
return False
Nei codici di esempio di cui sopra, lo rendiamo compatibile con Python 2/3 elencando sia FileNotFoundError
che IOError
nella parte di cattura delle eccezioni.
os.path.isfile()
per controllare se il file esiste (>=Python 2.x)
import os
fileName = "temp.txt"
os.path.isfile(fileName)
Controlla se il file fileName
esiste.
Alcuni sviluppatori preferiscono usare os.path.exists()
per verificare se un file esiste. Ma non è in grado di distinguere se l’oggetto è un file o una directory.
import os
fileName = "temp.txt"
print(os.path.exists(fileName))
fileName = r"C:\Test"
print(os.path.exists(fileName))
Quindi, usare os.path.isfile
solo se si vuole controllare se il file esiste.
pathlib.Path.is_file()
per verificare se il file esiste (>=Python 3.4)
A partire da Python 3.4, introduce un metodo orientato agli oggetti nel modulo pathlib
per controllare se un file esiste.
from pathlib import Path
fileName = "temp.txt"
fileObj = Path(fileName)
print(fileObj.is_file())
Allo stesso modo, ha anche metodi is_dir()
e exists()
per verificare se esiste una directory o un file/cartella.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook