Da JSON a CSV in Python
-
Usa il metodo Pandas DataFrames
to_csv()
per convertire JSON in CSV in Python -
Utilizza il modulo
csv
per convertire JSON in un file CSV
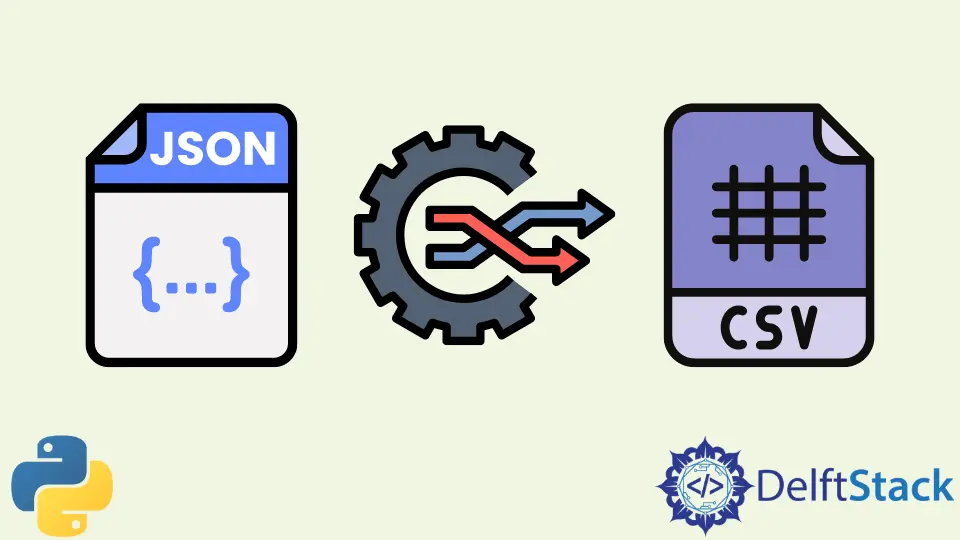
JSON sta per JavaScript Object Notation
. Si basa sul formato degli oggetti in JavaScript ed è una tecnica di codifica per rappresentare i dati strutturati. È ampiamente utilizzato in questi giorni, soprattutto per la condivisione di dati tra server e applicazioni web.
Un file CSV viene utilizzato per archiviare i dati in un formato tabulare come i fogli di calcolo Excel.
In questo tutorial impareremo come convertire i dati JSON in un file CSV.
Usa il metodo Pandas DataFrames to_csv()
per convertire JSON in CSV in Python
In questo metodo, convertiremo prima il JSON in un Pandas DataFrame e da lì lo convertiremo in un file CSV utilizzando il metodo to_csv()
. Possiamo leggere la stringa JSON utilizzando la funzione json.loads()
fornita nella libreria json
in Python per convertire JSON in un DataFrame. Quindi passiamo questo oggetto JSON alla funzione json_normalize()
che restituirà un Pandas DataFrame contenente i dati richiesti.
Il seguente frammento di codice spiegherà come lo facciamo.
import pandas as pd
import json
data = """
{
"Results":
[
{ "id": "1", "Name": "Jay" },
{ "id": "2", "Name": "Mark" },
{ "id": "3", "Name": "Jack" }
],
"status": ["ok"]
}
"""
info = json.loads(data)
df = pd.json_normalize(info["Results"])
df.to_csv("samplecsv.csv")
Il contenuto del file CSV creato è di seguito.
,id,Name
0,1,Jay
1,2,Mark
2,3,Jack
Utilizza il modulo csv
per convertire JSON in un file CSV
In questo metodo, useremo la libreria csv
in Python che è usata per leggere e scrivere file CSV. Innanzitutto, leggeremo i dati JSON come abbiamo fatto nel metodo precedente. Apriamo un file in modalità di scrittura e usiamo il DictWriter()
dal modulo csv
per creare un oggetto che ci permette di mappare e scrivere dati JSON nel file. I fieldnames
sono chiavi che vengono identificate e abbinate ai dati quando scriviamo righe usando la funzione writerows()
.
Il seguente frammento di codice mostrerà come implementare il metodo precedente:
import csv
import json
data = """
{
"Results":
[
{ "id": "1", "Name": "Jay" },
{ "id": "2", "Name": "Mark" },
{ "id": "3", "Name": "Jack" }
],
"status": ["ok"]
}
"""
info = json.loads(data)["Results"]
print(info[0].keys())
with open("samplecsv.csv", "w") as f:
wr = csv.DictWriter(f, fieldnames=info[0].keys())
wr.writeheader()
wr.writerows(info)
Manav is a IT Professional who has a lot of experience as a core developer in many live projects. He is an avid learner who enjoys learning new things and sharing his findings whenever possible.
LinkedInArticolo correlato - Python JSON
- Ottieni JSON dall'URL in Python
- Come stampare un file JSON in Python
- Scrivi JSON su un file in Python
- Converti file CSV in file JSON in Python
- Converti XML in JSON in Python