Converti colonna DataFrame in stringa in Pandas
-
Pandas DataFrame Series Metodo
astype(str)
-
Metodo DataFrame
apply
per operare sugli elementi nella colonna
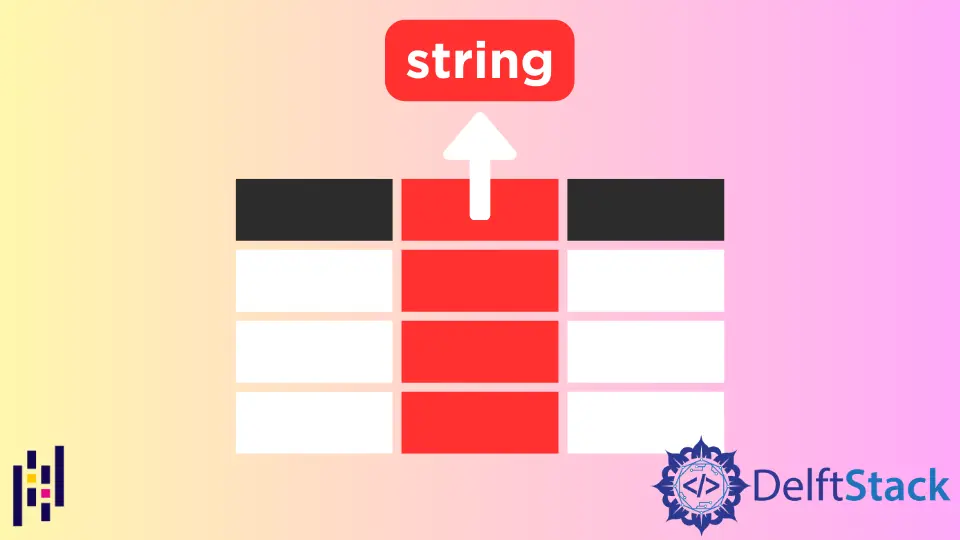
Introdurremo metodi per convertire la colonna DataFrame di Pandas in string
.
- Metodo Pandas DataFrame Series
astype(str)
- Metodo DataFrame
apply
per operare sugli elementi nella colonna
Useremo lo stesso DataFrame di seguito in questo articolo.
import pandas as pd
df = pd.DataFrame({"A": [1, 2, 3], "B": [4.1, 5.2, 6.3], "C": ["7", "8", "9"]})
print(df)
print(df.dtypes)
A B C
0 1 4.1 7
1 2 5.2 8
2 3 6.3 9
A int64
B float64
C object
dtype: object
Pandas DataFrame Series Metodo astype(str)
Metodo Pandas Series astype(dtype)
converte la serie Pandas nel tipo dtype
specificato.
pandas.Series.astype(str)
Converte la colonna Serie, DataFrame come in questo articolo, in stringa
.
>>> df
A B C
0 1 4.1 7
1 2 5.2 8
2 3 6.3 9
>>> df['A'] = df['A'].astype(str)
>>> df
A B C
0 1 4.1 7
1 2 5.2 8
2 3 6.3 9
>>> df.dtypes
A object
B float64
C object
dtype: object
Il metodo astype()
non modifica i dati DataFrame
sul posto, quindi dobbiamo assegnare la Pandas Series
restituita alla specifica colonna DataFrame
.
Potremmo anche convertire più colonne in stringhe contemporaneamente inserendo i nomi delle colonne tra parentesi quadre per formare una lista.
>>> df[['A','B']] = df[['A','B']].astype(str)
>>> df
A B C
0 1 4.1 7
1 2 5.2 8
2 3 6.3 9
>>> df.dtypes
A object
B object
C object
dtype: object
Metodo DataFrame apply
per operare sugli elementi nella colonna
apply(func, *args, **kwds)
Il metodo apply
di DataFrame
applica la funzione func
a ciascuna colonna o riga.
Potremmo usare la funzione lambda
al posto di func
per semplicità.
>>> df['A'] = df['A'].apply(lambda _: str(_))
>>> df
A B C
0 1 4.1 7
1 2 5.2 8
2 3 6.3 9
>>> df.dtypes
A object
B float64
C object
dtype: object
Non è possibile utilizzare il metodo apply
per applicare la funzione a più colonne.
>>> df[['A','B']] = df[['A','B']].apply(lambda _: str(_))
Traceback (most recent call last):
File "<pyshell#31>", line 1, in <module>
df[['A','B']] = df[['A','B']].apply(lambda _: str(_))
File "D:\WinPython\WPy-3661\python-3.6.6.amd64\lib\site-packages\pandas\core\frame.py", line 3116, in __setitem__
self._setitem_array(key, value)
File "D:\WinPython\WPy-3661\python-3.6.6.amd64\lib\site-packages\pandas\core\frame.py", line 3144, in _setitem_array
self.loc._setitem_with_indexer((slice(None), indexer), value)
File "D:\WinPython\WPy-3661\python-3.6.6.amd64\lib\site-packages\pandas\core\indexing.py", line 606, in _setitem_with_indexer
raise ValueError('Must have equal len keys and value '
ValueError: Must have equal len keys and value when setting with an iterable
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn FacebookArticolo correlato - Pandas DataFrame
- Come ottenere le intestazioni delle colonne DataFrame Pandas come lista
- Come cancellare la colonna DataFrame Pandas DataFrame
- Come convertire la colonna DataFrame in data e ora in pandas
- Converti un Float in un Integer in Pandas DataFrame
- Ordina Pandas DataFrame in base ai valori di una colonna
- Ottieni l'aggregato di Pandas Group-By e Sum