Ottieni la prima riga di pandas dataframe
-
Ottieni la prima riga di un DataFrame Pandas utilizzando la proprietà
pandas.DataFrame.iloc
- Ottieni la prima riga da un DataFrame Pandas in base alla condizione specificata
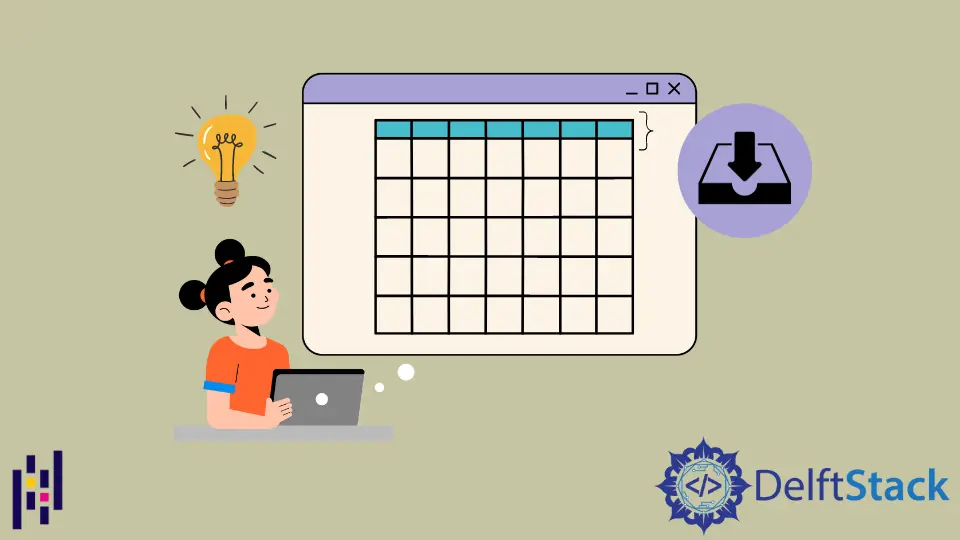
Questo tutorial spiega come possiamo ottenere la prima riga da un Pandas DataFrame usando la proprietà pandas.DataFrame.iloc
e il metodo pandas.DataFrame.head()
.
Useremo il DataFrame nell’esempio seguente per spiegare come possiamo ottenere la prima riga da un Pandas DataFrame.
import pandas as pd
df = pd.DataFrame(
{
"C_1": ["A", "B", "C", "D"],
"C_2": [40, 34, 38, 45],
"C_3": [430, 980, 200, 350],
}
)
print(df)
Produzione:
C_1 C_2 C_3
0 A 40 430
1 B 34 980
2 C 38 200
3 D 45 350
Ottieni la prima riga di un DataFrame Pandas utilizzando la proprietà pandas.DataFrame.iloc
import pandas as pd
df = pd.DataFrame(
{
"C_1": ["A", "B", "C", "D"],
"C_2": [40, 34, 38, 45],
"C_3": [430, 980, 200, 350],
}
)
row_1 = df.iloc[0]
print("The DataFrame is:")
print(df, "\n")
print("The First Row of the DataFrame is:")
print(row_1)
Produzione:
The DataFrame is:
C_1 C_2 C_3
0 A 40 430
1 B 34 980
2 C 38 200
3 D 45 350
The First Row of the DataFrame is:
C_1 A
C_2 40
C_3 430
Name: 0, dtype: object
Visualizza la prima riga del DataFrame df
. Per selezionare la prima riga, usiamo l’indice predefinito della prima riga, cioè 0
con la proprietà iloc
del DataFrame.
Ottieni la prima riga da un DataFrame Pandas usando il metodo pandas.DataFrame.head()
Il metodo pandas.DataFrame.head()
restituisce un DataFrame con le prime 5 righe del DataFrame. Possiamo anche passare un numero come argomento al metodo pandas.DataFrame.head()
che rappresenta il numero di righe più in alto da selezionare. Possiamo passare 1 come argomento al metodo pandas.DataFrame.head()
per selezionare solo la prima riga del DataFrame.
import pandas as pd
df = pd.DataFrame(
{
"C_1": ["A", "B", "C", "D"],
"C_2": [40, 34, 38, 45],
"C_3": [430, 980, 200, 350],
}
)
row_1 = df.head(1)
print("The DataFrame is:")
print(df, "\n")
print("The First Row of the DataFrame is:")
print(row_1)
Produzione:
The DataFrame is:
C_1 C_2 C_3
0 A 40 430
1 B 34 980
2 C 38 200
3 D 45 350
The First Row of the DataFrame is:
C_1 C_2 C_3
0 A 40 430
Ottieni la prima riga da un DataFrame Pandas in base alla condizione specificata
Per estrarre la prima riga che soddisfa le condizioni specificate da un DataFrame, inizialmente filtriamo le righe che soddisfano le condizioni specificate e quindi selezioniamo la prima riga dal DataFrame filtrato utilizzando i metodi discussi sopra.
import pandas as pd
df = pd.DataFrame(
{
"C_1": ["A", "B", "C", "D"],
"C_2": [40, 34, 38, 45],
"C_3": [430, 980, 500, 350],
}
)
filtered_df = df[(df.C_2 < 40) & (df.C_3 > 450)]
row_1_filtered = filtered_df.head(1)
print("The DataFrame is:")
print(df, "\n")
print("The Filtered DataFrame is:")
print(filtered_df, "\n")
print("The First Row with C_2 less than 45 and C_3 greater than 450 is:")
print(row_1_filtered)
Produzione:
The DataFrame is:
C_1 C_2 C_3
0 A 40 430
1 B 34 980
2 C 38 500
3 D 45 350
The Filtered DataFrame is:
C_1 C_2 C_3
1 B 34 980
2 C 38 500
The First Row with C_2 less than 45 and C_3 greater than 450 is:
C_1 C_2 C_3
1 B 34 980
Verrà visualizzata la prima riga con il valore della colonna C_2
inferiore a 45 e il valore della colonna C_3
maggiore di 450.
Possiamo anche usare il metodo query()
per filtrare le righe dal DataFrame.
import pandas as pd
df = pd.DataFrame(
{
"C_1": ["A", "B", "C", "D"],
"C_2": [40, 34, 38, 45],
"C_3": [430, 980, 500, 350],
}
)
filtered_df = df.query("(C_2 < 40) & (C_3 > 450)")
row_1_filtered = filtered_df.head(1)
print("The DataFrame is:")
print(df, "\n")
print("The Filtered DataFrame is:")
print(filtered_df, "\n")
print("The First Row with C_2 less than 45 and C_3 greater than 450 is:")
print(row_1_filtered)
Produzione:
The DataFrame is:
C_1 C_2 C_3
0 A 40 430
1 B 34 980
2 C 38 500
3 D 45 350
The Filtered DataFrame is:
C_1 C_2 C_3
1 B 34 980
2 C 38 500
The First Row with C_2 less than 45 and C_3 greater than 450 is:
C_1 C_2 C_3
1 B 34 980
Filtrerà tutte le righe con il valore della colonna C_2
inferiore a 45 e il valore della colonna C_3
maggiore di 450 utilizzando il metodo query()
e quindi selezionare la prima riga da filtered_df
usando il metodo head()
.
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedInArticolo correlato - Pandas DataFrame Row
- Ottieni il conteggio delle righe di un DataFrame Pandas
- Mescola casualmente le righe DataFrame in Pandas
- Filtra le righe del dataframe in base ai valori delle colonne in Pandas
- Scorri le righe di un DataFrame in Pandas
- Ottieni l'indice di tutte le righe la cui colonna specifica soddisfa una determinata condizione in Pandas
- Ottieni l'indice delle righe la cui colonna corrisponde a un valore specifico in Pandas