Converti XML in JSON in PHP
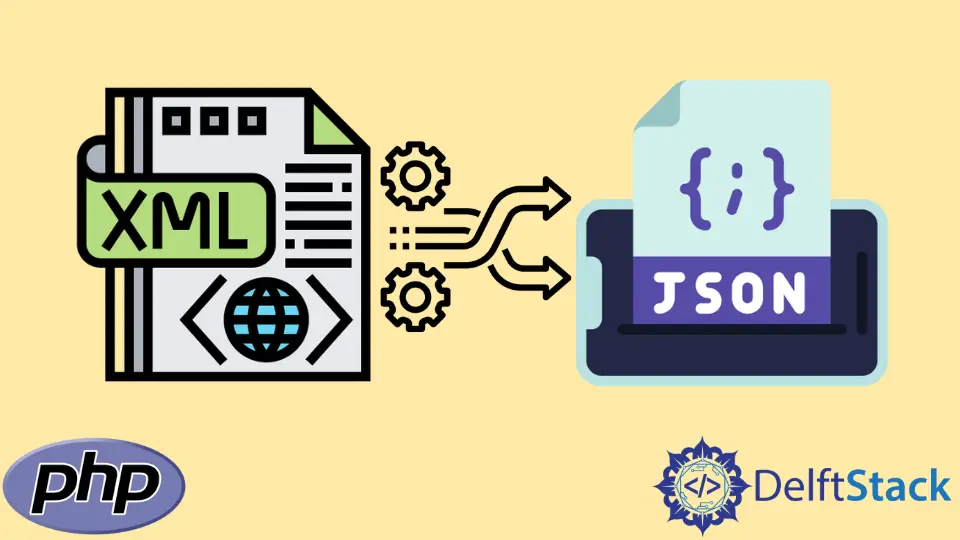
Questo articolo introdurrà un metodo per convertire una stringa XML in JSON in PHP.
Usa la funzione simplexml_load_string()
e json_encode()
per convertire una stringa XML in JSON in PHP
Useremo due funzioni per convertire una stringa XML in JSON in PHP perché non esiste una funzione specializzata per la conversione diretta. Queste due funzioni sono simplexml_load_string()
e json_encode()
. La sintassi corretta per utilizzare queste funzioni per la conversione di una stringa XML in JSON è la seguente.
simplexml_load_string($data, $class_name, $options, $ns, $is_prefix);
La funzione simplexml_load_string()
accetta cinque parametri. I dettagli dei suoi parametri sono i seguenti.
Variabili | Descrizione | |
---|---|---|
$data |
obbligatorio | Una stringa XML ben formata. |
$class_name |
opzionale | Usiamo questo parametro opzionale in modo che simplexml_load_string() restituisca un oggetto della classe specificata. Quella classe dovrebbe estendere la classe SimpleXMLElement. |
options |
opzionale | Possiamo anche usare il parametro options per specificare parametri Libxml aggiuntivi. |
ns |
opzionale | Il prefisso dello spazio dei nomi o l’URI. |
$is_prefix |
opzionale | Impostato su true se $ns è un prefisso, false se è un URI. Il suo valore predefinito false . |
Questa funzione restituisce l’oggetto della classe SimpleXMLElement
contenente i dati contenuti nella stringa XML, o False
in caso di errore.
json_encode($value, $flags, $depth);
La funzione json_encode()
ha tre parametri. I dettagli dei suoi parametri sono i seguenti.
Variabili | Descrizione | |
---|---|---|
$value |
obbligatorio | Il valore da codificare. |
$flags |
opzionale | Maschera di bit consistono di JSON_FORCE_OBJECT , JSON_HEX_QUOT , JSON_HEX_TAG , JSON_HEX_AMP , JSON_HEX_APOS , JSON_INVALID_UTF8_IGNORE , JSON_INVALID_UTF8_SUBSTITUTE , JSON_NUMERIC_CHECK , JSON_PARTIAL_OUTPUT_ON_ERROR , JSON_PRESERVE_ZERO_FRACTION , JSON_PRETTY_PRINT , JSON_UNESCAPED_LINE_TERMINATORS , JSON_UNESCAPED_SLASHES , JSON_UNESCAPED_UNICODE , JSON_THROW_ON_ERROR . |
$depth |
opzionale | La profondità massima. Dovrebbe essere maggiore di zero. |
Questa funzione restituisce il valore JSON. Il programma seguente mostra i modi in cui possiamo usare le funzioni simplexml_load_string()
e json_encode()
per convertire una stringa XML in JSON in PHP.
<?php
$xml_string = <<<XML
<?xml version='1.0' standalone='yes'?>
<movies>
<movie>
<title>PHP: Behind the Parser</title>
<characters>
<character>
<name>Ms. Coder</name>
<actor>Onlivia Actora</actor>
</character>
<character>
<name>Mr. Coder</name>
<actor>El ActÓr</actor>
</character>
</characters>
<plot>
So, this language. It is like, a programming language. Or is it a
scripting language? All is revealed in this thrilling horror spoof
of a documentary.
</plot>
<great-lines>
<line>PHP solves all my web problems</line>
</great-lines>
<rating type="thumbs">7</rating>
<rating type="stars">5</rating>
</movie>
</movies>
XML;
$xml = simplexml_load_string($xml_string);
$json = json_encode($xml); // convert the XML string to JSON
var_dump($json);
?>
Produzione:
string(415) "{"movie":{"title":"PHP: Behind the Parser","characters":{"character":[{"name":"Ms. Coder","actor":"Onlivia Actora"},{"name":"Mr. Coder","actor":"El Act\u00d3r"}]},"plot":"\n So, this language. It is like, a programming language. Or is it a\n scripting language? All is revealed in this thrilling horror spoof\n of a documentary.\n ","great-lines":{"line":"PHP solves all my web problems"},"rating":["7","5"]}}"