Estrai dati da JSON in PHP
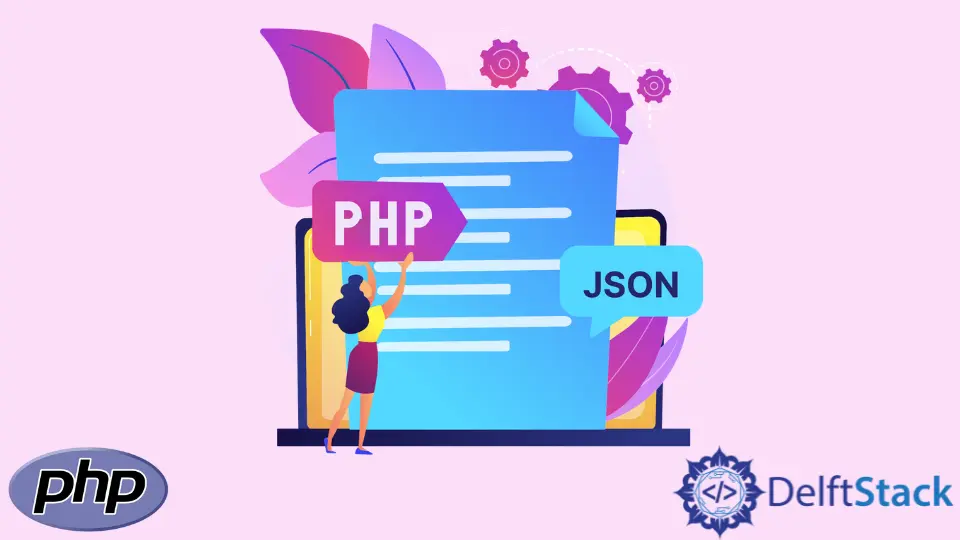
In questo articolo, introdurremo metodi per estrarre dati da JSON in PHP.
- Utilizzo della funzione
json_decode()
Usa la funzione json_decode()
per estrarre dati da JSON in PHP
Useremo la funzione incorporata json_decode()
per estrarre i dati da JSON. Convertiremo la stringa JSON in un oggetto o in un array per estrarre i dati. La sintassi corretta per utilizzare questa funzione è la seguente.
json_decode($jsonString, $assoc, $depth, $options);
La funzione incorporata json_decode()
ha quattro parametri. I dettagli dei suoi parametri sono i seguenti
Parametri | Descrizione | |
---|---|---|
$jsonString |
obbligatorio | È la stringa codificata JSON da cui vogliamo estrarre i dati. |
$assoc |
opzionale | È una variabile booleana . Se è TRUE, la funzione restituirà un array associativo. Se FALSE, la funzione restituirà l’oggetto. |
$depth |
opzionale | È un numero intero. Specifica la profondità specificata. |
$options |
opzionale | Specifica la maschera di bit di JSON_BIGINT_AS_STRING , JSON_INVALID_UTF8_IGNORE , JSON_INVALID_UTF8_SUBSTITUTE , JSON_OBJECT_AS_ARRAY , JSON_THROW_ON_ERROR . Puoi controllare i loro dettagli qui. |
Questa funzione restituisce NULL se la stringa JSON non è in un formato corretto. Restituisce un array o un oggetto associativo a seconda del parametro $assoc
.
Il programma seguente mostra come possiamo usare la funzione json_decode()
per estrarre dati da una stringa JSON.
<?php
$jsonString ='{
"firstName":"Olivia",
"lastName":"Mason",
"dateOfBirth":
{
"year":"1999",
"month":"06",
"day":"19"
}
}';
$data = json_decode($jsonString);
echo("The data is: \n");
var_dump($data);
?>
La funzione restituirà un oggetto perché non abbiamo passato il parametro $assoc
.
Produzione:
The data is:
object(stdClass)#1 (3) {
["firstName"]=>
string(6) "Olivia"
["lastName"]=>
string(5) "Mason"
["dateOfBirth"]=>
object(stdClass)#2 (3) {
["year"]=>
string(4) "1999"
["month"]=>
string(2) "06"
["day"]=>
string(2) "19"
}
}
Se passiamo il parametro $assoc
, la funzione restituirà un array associativo.
<?php
$jsonString ='{
"firstName":"Olivia",
"lastName":"Mason",
"dateOfBirth":
{
"year":"1999",
"month":"06",
"day":"19"
}
}';
$data = json_decode($jsonString, true);
echo("The data is: \n");
var_dump($data);
?>
Produzione:
The data is:
array(3) {
["firstName"]=>
string(6) "Olivia"
["lastName"]=>
string(5) "Mason"
["dateOfBirth"]=>
array(3) {
["year"]=>
string(4) "1999"
["month"]=>
string(2) "06"
["day"]=>
string(2) "19"
}
}
Se la funzione ha restituito un oggetto, possiamo accedere ai dati nel modo seguente:
<?php
$jsonString ='{
"firstName":"Olivia",
"lastName":"Mason",
"dateOfBirth":
{
"year":"1999",
"month":"06",
"day":"19"
}
}';
$data = json_decode($jsonString);
echo("The first name is: \n");
echo $data->firstName;
?>
La funzione restituirà il primo nome dai dati estratti.
Produzione:
The first name is:
Olivia
Se la funzione ha restituito un array, possiamo accedere direttamente ai dati nel modo seguente:
<?php
$jsonString ='{
"firstName":"Olivia",
"lastName":"Mason",
"dateOfBirth":
{
"year":"1999",
"month":"06",
"day":"19"
}
}';
$data = json_decode($jsonString, true);
echo("The first name is: \n");
echo $data['firstName'];
?>
La funzione restituirà il primo nome dai dati estratti.
Produzione:
The first name is:
Olivia
Possiamo anche iterare attraverso il nostro array.
<?php
$jsonString ='{
"firstName":"Olivia",
"lastName":"Mason",
"dateOfBirth": "19-09-1999"
}';
$data = json_decode($jsonString, true);
foreach ($data as $key=> $data1) {
echo $key, " : ";
echo $data1, "\n";
}
?>
La funzione restituirà i dati estratti.
Produzione:
firstName : Olivia
lastName : Mason
dateOfBirth : 19-09-1999