Converti stringa in InputStream in Java
-
Usa
ByteArrayInputStream()
per convertire una stringa inInputStream
in Java -
Usa
StringReader
eReaderInputStream
per convertire una stringa in unInputStream
in Java -
Usa
org.apache.commons.io.IOUtils
per convertire una stringa in unInputStream
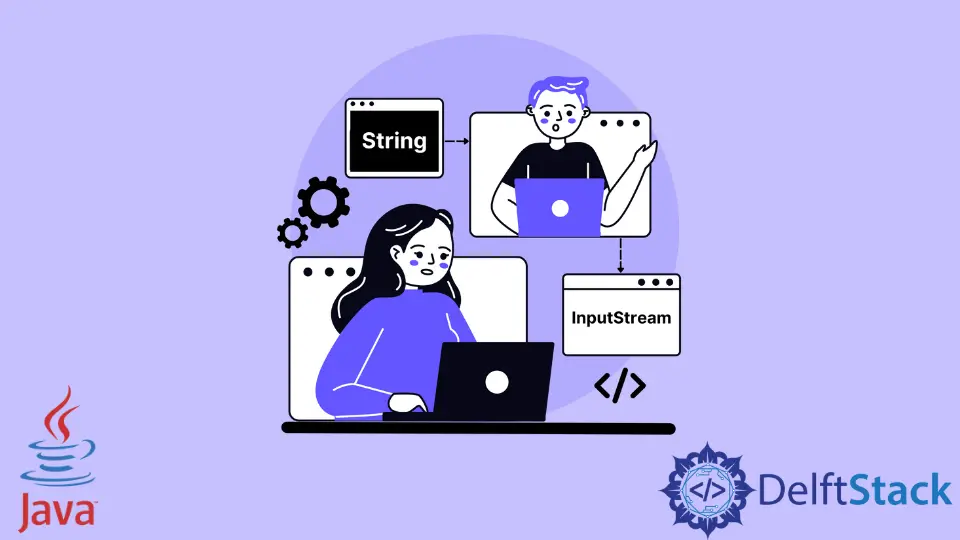
Parleremo di come convertire una stringa in un InputStream
in Java utilizzando diversi metodi. Una stringa è un insieme di caratteri, mentre un InputStream
è un insieme di byte. Vediamo come possiamo convertire una stringa in InputStream
in Java.
Usa ByteArrayInputStream()
per convertire una stringa in InputStream
in Java
Il pacchetto Input/Output di Java ha la classe ByteArrayInputStream
che legge gli array di byte come InputStream
. Per prima cosa, usiamo getBytes()
per ottenere i byte da exampleString
con il set di caratteri UTF_8, e poi li passiamo a ByteArrayInputStream
.
Per verificare se riusciamo nel nostro obiettivo, possiamo leggere inputStream
usando read()
e convertire ogni byte
in un char
. Questo restituirà la nostra stringa originale.
import java.io.ByteArrayInputStream;
import java.io.IOException;
import java.io.InputStream;
import java.nio.charset.StandardCharsets;
public class Main {
public static void main(String[] args) throws IOException {
String exampleString = "This is a sample string";
InputStream inputStream =
new ByteArrayInputStream(exampleString.getBytes(StandardCharsets.UTF_8));
// To check if we can read the string back from the inputstream
int i;
while ((i = inputStream.read()) != -1) {
char getSingleChar = (char) i;
System.out.print(getSingleChar);
}
}
}
Produzione:
This is a sample string
Usa StringReader
e ReaderInputStream
per convertire una stringa in un InputStream
in Java
La seconda tecnica per convertire la stringa in InputStream
utilizza due metodi, StringReader
e ReaderInputStream
. Il primo serve per leggere la stringa e avvolgerlo in un reader
mentre il secondo prende due argomenti, un reader
e i charset
. Alla fine, otteniamo l’InputStream
.
import java.io.IOException;
import java.io.InputStream;
import java.io.StringReader;
import java.nio.charset.StandardCharsets;
import org.apache.commons.io.input.ReaderInputStream;
public class Main {
public static void main(String[] args) throws IOException {
String exampleString = "This is a sample string";
StringReader stringReader = new StringReader(exampleString);
InputStream inputStream = new ReaderInputStream(stringReader, StandardCharsets.UTF_8);
// To check if we can read the string back from the inputstream
int i;
while ((i = inputStream.read()) != -1) {
char getSingleChar = (char) i;
System.out.print(getSingleChar);
}
}
}
Produzione:
This is a sample string
Usa org.apache.commons.io.IOUtils
per convertire una stringa in un InputStream
Possiamo anche utilizzare la libreria Apache Commons per semplificare il nostro compito. La classe IOUtls
di questa libreria Apache Commons ha un metodo toInputStream()
che accetta una stringa e il set di caratteri da utilizzare. Questo metodo è il più semplice di tutti in quanto dobbiamo chiamare un solo metodo per convertire la stringa Java in InputStream
.
import java.io.IOException;
import java.io.InputStream;
import java.nio.charset.StandardCharsets;
import org.apache.commons.io.IOUtils;
public class Main {
public static void main(String[] args) throws IOException {
String exampleString = "This is a sample string";
InputStream is = IOUtils.toInputStream(exampleString, StandardCharsets.UTF_8);
// To check if we can read the string back from the inputstream
int i;
while ((i = is.read()) != -1) {
char getSingleChar = (char) i;
System.out.print(getSingleChar);
}
}
}
Produzione:
This is a sample string
Rupam Saini is an android developer, who also works sometimes as a web developer., He likes to read books and write about various things.
LinkedIn