Ripeti su ogni elemento della mappa in Java
-
Come iterare elementi
Map
in Java -
Iterare gli elementi
Map
utilizzando il ciclifor
in Java -
Iterazione degli elementi
Map
utilizzandoforeach
in Java -
Iterare gli elementi
Map
utilizzandoEntry
eIteratore
in Java -
Itera gli elementi
Map
usandofor-each
ekeySet()
in Java -
Iterare gli elementi
Map
usandowhile-loop
in Java -
Itera gli elementi
Map
usandoStream
eforEach
in Java -
Iterazione degli elementi della mappa utilizzando
forEach
elambda
in Java
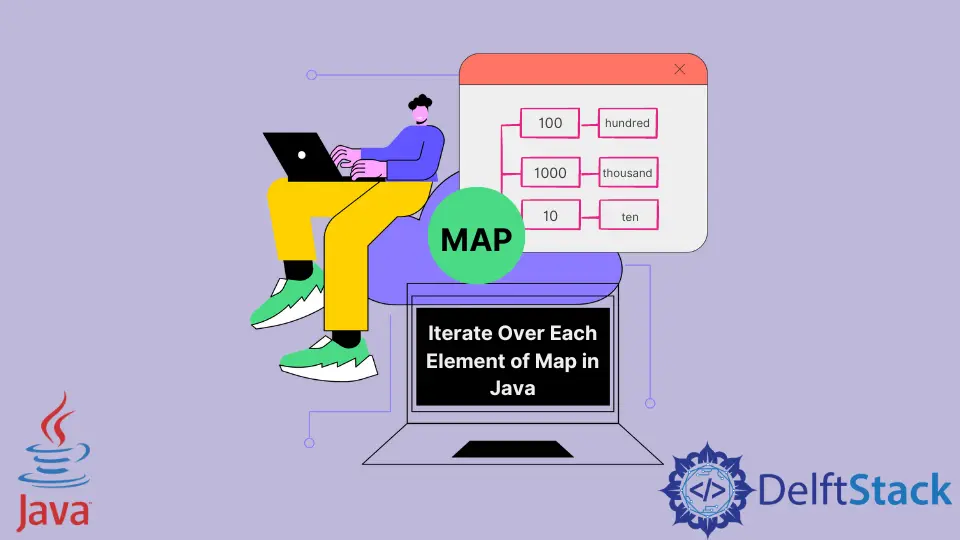
Questo tutorial introduce come iterare su ogni elemento della mappa ed elenca alcuni codici di esempio per comprenderlo.
Come iterare elementi Map
in Java
Map è un’interfaccia che viene utilizzata per raccogliere dati sotto forma di coppia chiave-valore. Java fornisce diversi modi per iterare gli elementi della mappa come il cicli for
, il bucle for-each
, il cicli while
, il metodo forEach()
, ecc. Vediamo gli esempi.
Iterare gli elementi Map
utilizzando il cicli for
in Java
Usiamo un semplice cicli for
per iterare gli elementi Map
. Qui, nel bucle iterator()
viene utilizzato il metodo per ottenere le voci.
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
public class SimpleTesting {
public static void main(String[] args) {
Map<Integer, String> map = new HashMap<>();
map.put(10, "Ten");
map.put(100, "Hundred");
map.put(1000, "Thousand");
for (Iterator<Map.Entry<Integer, String>> entries = map.entrySet().iterator();
entries.hasNext();) {
Map.Entry<Integer, String> entry = entries.next();
System.out.println(entry.getKey() + " : " + entry.getValue());
}
}
}
Produzione:
100 : Hundred
1000 : Thousand
10 : Ten
Iterazione degli elementi Map
utilizzando foreach
in Java
Usiamo il bucle for-each
e il metodo entrySet()
per iterare ogni voce della mappa. Il entrySet()
restituisce un insieme di voci della mappa.
import java.util.HashMap;
import java.util.Map;
public class SimpleTesting {
public static void main(String[] args) {
Map<Integer, String> map = new HashMap<>();
map.put(10, "Ten");
map.put(100, "Hundred");
map.put(1000, "Thousand");
for (Map.Entry<Integer, String> entry : map.entrySet()) {
System.out.println(entry.getKey() + ":" + entry.getValue());
}
}
}
Produzione:
100 : Hundred
1000 : Thousand
10 : Ten
Iterare gli elementi Map
utilizzando Entry
e Iteratore
in Java
Il metodo iterator()
restituisce un Iterator
per attraversare gli elementi mentre Entry
è usato per raccogliere l’entrata di Map
.
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
public class SimpleTesting {
public static void main(String[] args) {
Map<Integer, String> map = new HashMap<>();
map.put(10, "Ten");
map.put(100, "Hundred");
map.put(1000, "Thousand");
Iterator<Map.Entry<Integer, String>> entries = map.entrySet().iterator();
while (entries.hasNext()) {
Map.Entry<Integer, String> entry = entries.next();
System.out.println(entry.getKey() + ":" + entry.getValue());
}
}
}
Produzione:
100 : Hundred
1000 : Thousand
10 : Ten
Itera gli elementi Map
usando for-each
e keySet()
in Java
Il metodo keySet()
è usato per raccogliere il set di chiavi di Map
che è ulteriormente usato per iterare usando il bucle for-each
.
import java.util.HashMap;
import java.util.Map;
public class SimpleTesting {
public static void main(String[] args) {
Map<Integer, String> map = new HashMap<>();
map.put(10, "Ten");
map.put(100, "Hundred");
map.put(1000, "Thousand");
for (Integer key : map.keySet()) {
System.out.println(key + " : " + map.get(key));
}
}
}
Produzione:
100 : Hundred
1000 : Thousand
10 : Ten
Iterare gli elementi Map
usando while-loop
in Java
Qui, abbiamo usato il metodo iterator()
per ottenere l’iteratore delle chiavi e quindi iterare queste chiavi usando il bucle while. Per ottenere valore da una chiave, abbiamo utilizzato il metodo get()
.
import java.util.HashMap;
import java.util.Iterator;
import java.util.Map;
public class SimpleTesting {
public static void main(String[] args) {
Map<Integer, String> map = new HashMap<>();
map.put(10, "Ten");
map.put(100, "Hundred");
map.put(1000, "Thousand");
Iterator<Integer> itr = map.keySet().iterator();
while (itr.hasNext()) {
Integer key = itr.next();
System.out.println(key + " : " + map.get(key));
}
}
}
Produzione:
100 : Hundred
1000 : Thousand
10 : Ten
Itera gli elementi Map
usando Stream
e forEach
in Java
Possiamo usare stream per iterare gli elementi. Qui, abbiamo usato entrySet()
per raccogliere voci di mappa che hanno attraversato ulteriormente il metodo di flusso forEach()
.
import java.util.HashMap;
import java.util.Map;
public class SimpleTesting {
public static void main(String[] args) {
Map<Integer, String> map = new HashMap<>();
map.put(10, "Ten");
map.put(100, "Hundred");
map.put(1000, "Thousand");
map.entrySet().stream().forEach(System.out::println);
}
}
Produzione:
100=Hundred
1000=Thousand
10=Ten
Iterazione degli elementi della mappa utilizzando forEach
e lambda
in Java
Possiamo anche usare l’espressione lambda per iterare gli elementi della mappa. Qui, abbiamo usato l’espressione lambda all’interno del metodo forEach()
.
import java.util.HashMap;
import java.util.Map;
public class SimpleTesting {
public static void main(String[] args) {
Map<Integer, String> map = new HashMap<>();
map.put(10, "Ten");
map.put(100, "Hundred");
map.put(1000, "Thousand");
map.forEach((key, value) -> System.out.println(key + " : " + value));
}
}
Produzione:
100 : Hundred
1000 : Thousand
10 : Ten
Articolo correlato - Java Map
- Differenza tra hashmap e map in Java
- Converti JSON in Map in Java
- Ordina una mappa per valore in Java
- Ottieni chiave da valore in Java Hashmap