Controlla se una stringa è vuota o nulla in Java
Hassan Saeed
12 ottobre 2023
-
Usa
str == null
per verificare se una stringa ènull
in Java -
Usa
str.isEmpty()
per controllare se una stringa è vuota in Java
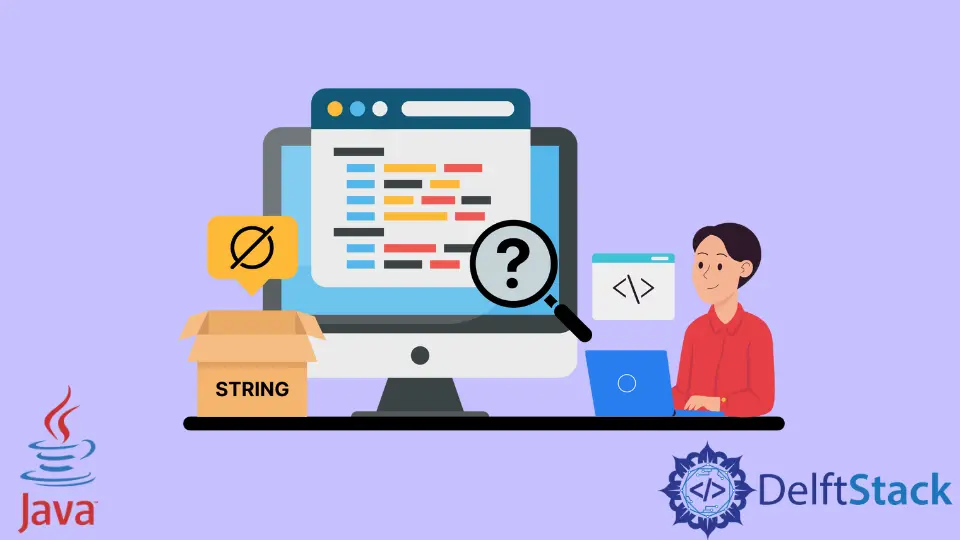
Questo tutorial discute i metodi per verificare se una stringa è vuota o nulla in Java.
Usa str == null
per verificare se una stringa è null
in Java
Il modo più semplice per verificare se una data stringa è null
in Java è confrontarla con null
usando str == null
. L’esempio seguente lo illustra:
public class MyClass {
public static void main(String args[]) {
String str1 = null;
String str2 = "Some text";
if (str1 == null)
System.out.println("str1 is a null string");
else
System.out.println("str1 is not a null string");
if (str2 == null)
System.out.println("str2 is a null string");
else
System.out.println("str2 is not a null string");
}
}
Produzione:
str1 is a null string
str2 is not a null string
Usa str.isEmpty()
per controllare se una stringa è vuota in Java
Il modo più semplice per verificare se una data stringa è vuota in Java è usare il metodo integrato della classe String
- isEmpty()
. L’esempio seguente lo illustra:
public class MyClass {
public static void main(String args[]) {
String str1 = "";
String str2 = "Some text";
if (str1.isEmpty())
System.out.println("str1 is an empty string");
else
System.out.println("str1 is not an empty string");
if (str2.isEmpty())
System.out.println("str2 is an empty string");
else
System.out.println("str2 is not an empty string");
}
}
Produzione:
str1 is an empty string
str2 is not an empty string
Se siamo interessati a verificare entrambe le condizioni contemporaneamente, possiamo farlo utilizzando l’operatore logico OR
- ||
. L’esempio seguente lo illustra:
public class MyClass {
public static void main(String args[]) {
String str1 = "";
if (str1.isEmpty() || str1 == null)
System.out.println("This is an empty or null string");
else
System.out.println("This is neither empty nor null string");
}
}
Produzione:
This is an empty or null string