Converti un valore Int in una stringa in Go
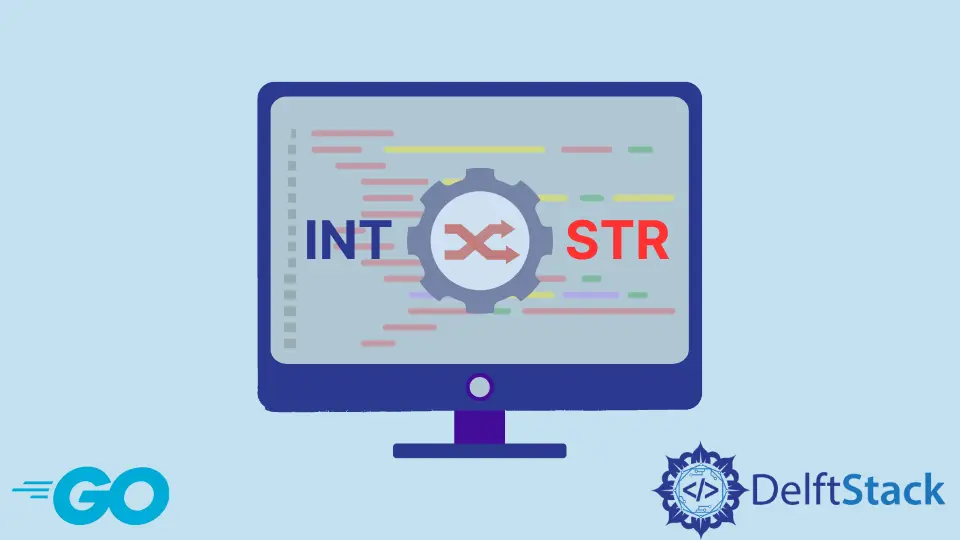
I tipi di dati determinano i valori che è possibile assegnare al tipo e le operazioni che possono essere eseguite su di esso. La conversione dei tipi di dati è un’operazione ampiamente utilizzata nella programmazione e tra le conversioni dei tipi di dati la conversione di int nel valore di stringa è ampiamente diffusa.
Viene utilizzato durante la stampa di un numero sullo schermo o quando si lavora con il numero come se fosse una stringa. Go fornisce la conversione di stringhe e numeri interi direttamente da un pacchetto proveniente dalla libreria standard strconv
.
Se usiamo la conversione semplice da int
a string
, il valore intero viene interpretato come un punto di codice Unicode. E la stringa risultante conterrà il carattere rappresentato dal punto di codice, codificato in UTF-8
.
package main
import "fmt"
func main() {
s := string(97)
fmt.Printf("Value of 97 after conversion : %v\n", s)
}
Produzione:
Value of 97 after conversion : a
Ma questo non è desiderabile, e quindi usiamo alcune funzioni standard per convertire interi nel tipo di dati stringa, che sono discussi di seguito:
Funzione Itoa
dal pacchetto strconv
Pacchetto strconv
implementa conversioni da e verso rappresentazioni di stringhe di tipi di dati di base. Per convertire un numero intero in una stringa, usiamo la funzione Itoa
dal pacchetto strconv
.
package main
import (
"fmt"
"strconv"
)
func main() {
x := 138
fmt.Printf("Datatype of 138 before conversion : %T\n", x)
a := strconv.Itoa(x)
fmt.Printf("Datatype of 138 after conversion : %T\n", a)
fmt.Println("Number: " + a)
}
Produzione:
Datatype of 138 before conversion : int
Datatype of 138 after conversion : string
Number: 138
Funzione FormatInt
dal pacchetto strconv
Usiamo strconv.FormatInt
per formattare un int64
in una data base. FormatInt
fornisce la rappresentazione di stringa dell’intero nella base menzionata, per 2
<= base
<= 36
e il risultato utilizza le lettere minuscole da a
a z
per i valori numerici >= 10
.
package main
import (
"fmt"
"strconv"
)
func main() {
var integer_1 int64 = 31
fmt.Printf("Value of integer_1 before conversion : %v\n", integer_1)
fmt.Printf("Datatype of integer_1 before conversion : %T\n", integer_1)
var string_1 string = strconv.FormatInt(integer_1, 10)
fmt.Printf("Value of integer_1 after conversion in base 10: %v\n", string_1)
fmt.Printf("Datatype of integer_1 after conversion in base 10 : %T\n", string_1)
var string_2 string = strconv.FormatInt(integer_1, 16)
fmt.Printf("Value of integer_1 after conversion in base 16 : %v\n", string_2)
fmt.Printf("Datatype of integer_1 after conversion in base 16 : %T\n", string_2)
}
Produzione:
Value of integer_1 before conversion : 31
Datatype of integer_1 before conversion : int64
Value of integer_1 after conversion in base 10: 31
Datatype of integer_1 after conversion in base 10 : string
Value of integer_1 after conversion in base 16 : 1f
Datatype of integer_1 after conversion in base 16 : string
Metodo fmt.Sprint
Quando passiamo un numero intero nel metodo fmt.Sprint
, otteniamo un valore stringa dell’intero.
package main
import (
"fmt"
)
func main() {
x := 138
fmt.Printf("Datatype of 138 before conversion : %T\n", x)
a := fmt.Sprint(x)
fmt.Printf("Datatype of 138 after conversion : %T\n", a)
fmt.Println("Number: " + a)
}
Produzione:
Datatype of 138 before conversion : int
Datatype of 138 after conversion : string
Number: 138
Suraj Joshi is a backend software engineer at Matrice.ai.
LinkedIn