Ottieni il valore ASCII di Char in C++
-
Usa
std::copy
estd::ostream_iterator
per ottenere il valore ASCII dichar
-
Usa gli identificatori di formato
printf
per ottenere il valore ASCII dichar
-
Usa
int()
per ottenere il valore ASCII dichar
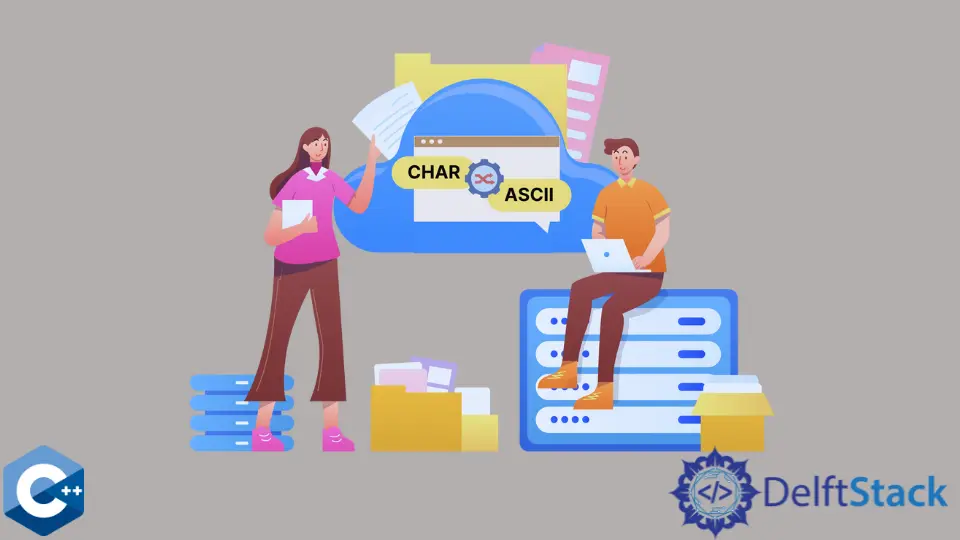
Questo articolo spiegherà diversi metodi su come ottenere il valore ASCII di char
in C++.
Usa std::copy
e std::ostream_iterator
per ottenere il valore ASCII di char
La codifica dei caratteri ASCII è quasi onnipresente nei computer anche se da allora sono emersi schemi standard più recenti, come UTF-8 e altri. Originariamente, ASCII codificava lettere inglesi, cifre decimali, simboli di punteggiatura e alcuni codici di controllo aggiuntivi. Tutti questi simboli sono rappresentati utilizzando un valore intero dalla gamma [0 - 127]
. Dato che i tipi char
sono implementati come numeri interi, possiamo trattarne i valori e inviarli allo stream cout
usando gli algoritmi ostream_iterator<int>
e std::copy
. Si noti che le lettere dell’alfabeto conseguenti hanno valori numerici adiacenti.
#include <iostream>
#include <iterator>
#include <vector>
using std::copy;
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<char> chars{'g', 'h', 'T', 'U', 'q', '%', '+', '!', '1', '2', '3'};
cout << "chars: ";
std::copy(chars.begin(), chars.end(), std::ostream_iterator<int>(cout, "; "));
return EXIT_SUCCESS;
}
Produzione:
chars: 103; 104; 84; 85; 113; 37; 43; 33; 49; 50; 51;
Usa gli identificatori di formato printf
per ottenere il valore ASCII di char
La funzione printf
è un’altra alternativa per visualizzare i valori char
con i codici ASCII corrispondenti. Nota che printf
accetta entrambi gli argomenti come tipi char
e differenzia solo gli specificatori di formato %c
/%d
. Anche se questi ultimi specificatori sono normalmente documentati come formattatori char
e int
, rispettivamente, sono compatibili con i tipi dell’altro.
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<char> chars{'g', 'h', 'T', 'U', 'q', '%', '+', '!', '1', '2', '3'};
cout << endl;
for (const auto &number : chars) {
printf("The ASCII value of '%c' is: %d\n", number, number);
}
return EXIT_SUCCESS;
}
Produzione:
The ASCII value of 'g' is: 103
The ASCII value of 'h' is: 104
The ASCII value of 'T' is: 84
The ASCII value of 'U' is: 85
The ASCII value of 'q' is: 113
The ASCII value of '%' is: 37
The ASCII value of '+' is: 43
The ASCII value of '!' is: 33
The ASCII value of '1' is: 49
The ASCII value of '2' is: 50
The ASCII value of '3' is: 51
Usa int()
per ottenere il valore ASCII di char
Infine, si possono convertire i valori char
usando la notazione int(c)
e visualizzare i valori direttamente nel flusso cout
come qualsiasi altra variabile. Nota che questo esempio è più in stile C++ rispetto alla versione printf
con i suoi specificatori di formato. Al contrario, i valori dei caratteri ASCII possono essere stampati dai valori int
utilizzando la notazione char(i)
.
#include <iostream>
#include <vector>
using std::cout;
using std::endl;
using std::vector;
int main() {
vector<char> chars{'g', 'h', 'T', 'U', 'q', '%', '+', '!', '1', '2', '3'};
cout << endl;
for (auto &number : chars) {
cout << "The ASCII value of '" << number << "' is: " << int(number) << endl;
}
return EXIT_SUCCESS;
}
Produzione:
The ASCII value of 'g' is: 103
The ASCII value of 'h' is: 104
The ASCII value of 'T' is: 84
The ASCII value of 'U' is: 85
The ASCII value of 'q' is: 113
The ASCII value of '%' is: 37
The ASCII value of '+' is: 43
The ASCII value of '!' is: 33
The ASCII value of '1' is: 49
The ASCII value of '2' is: 50
The ASCII value of '3' is: 51
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook