Array di strutture in C
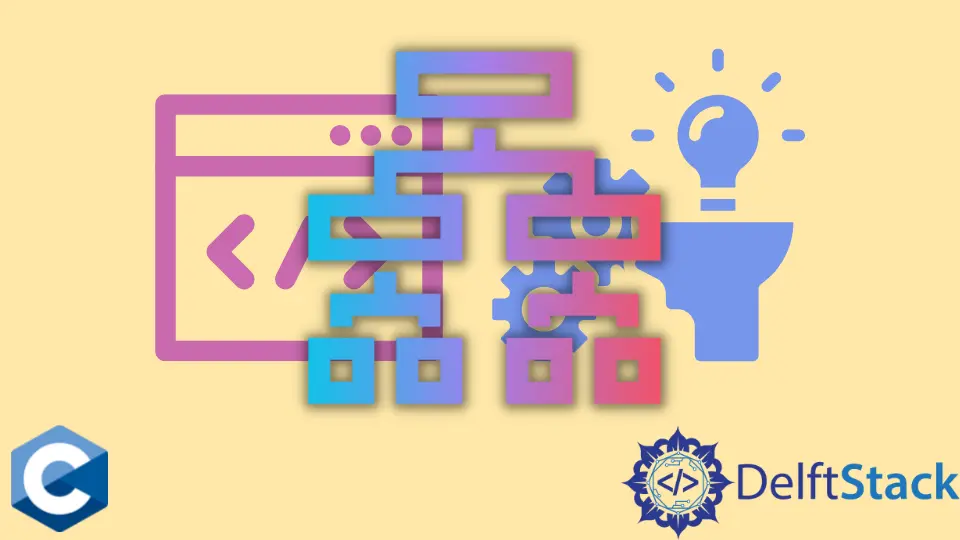
Questo tutorial introduce come creare un array di strutture in C. È la raccolta di più variabili di struttura in cui ogni variabile contiene informazioni su entità diverse.
Array di struct
in C
Un array è una raccolta sequenziale dello stesso tipo di dati e una struttura è un tipo di dati definito dall’utente. La dichiarazione di un array di strutture è la stessa di un array dei tipi di dati primitivi, ma utilizza la struttura ha il tipo di dati dei suoi elementi.
Considera un esempio di una struttura denominata Student
come di seguito:
struct Student {
int rollNumber;
char studentName[10];
float percentage;
};
Possiamo dichiarare un array di strutture come di seguito.
struct Student studentRecord[5];
Qui, lo studentRecord
è un array di 5 elementi in cui ogni elemento è di tipo struct
Student
. Si accede ai singoli elementi utilizzando la notazione indice ([]
) e si accede ai membri utilizzando il punto .
operatore.
Lo studentRecord[0]
punta all’elemento 0th
dell’array, e lo studentRecord[1]
punta al primo elemento dell’array.
Allo stesso modo,
- Lo
studentRecord[0].rollNumber
si riferisce al membrorollNumber
dello 0° elemento dell’array. - Lo
studentRecord[0].studentName
si riferisce al membrostudentName
del 0° elemento dell’array. - Lo
studentRecord[0].percentage
si riferisce al membropercentuale
del 0° elemento dell’array.
Il programma completo per dichiarare un array della struct
in C è il seguente.
#include <stdio.h>
#include <string.h>
struct Student {
int rollNumber;
char studentName[10];
float percentage;
};
int main(void) {
int counter;
struct Student studentRecord[5];
printf("Enter Records of 5 students");
for (counter = 0; counter < 5; counter++) {
printf("\nEnter Roll Number:");
scanf("%d", &studentRecord[counter].rollNumber);
printf("\nEnter Name:");
scanf("%s", &studentRecord[counter].studentName);
printf("\nEnter percentage:");
scanf("%f", &studentRecord[counter].percentage);
}
printf("\nStudent Information List:");
for (counter = 0; counter < 5; counter++) {
printf("\nRoll Number:%d\t Name:%s\t Percentage :%f\n",
studentRecord[counter].rollNumber,
studentRecord[counter].studentName,
studentRecord[counter].percentage);
}
return 0;
}
Produzione:
Enter Record of 5 students
Enter Roll number:1
Enter Name: John
Enter percentage: 78
Enter Roll number:2
Enter Name: Nick
Enter percentage: 84
Enter Roll number:3
Enter Name: Jenny
Enter percentage: 56
Enter Roll number:4
Enter Name: Jack
Enter percentage: 77
Enter Roll number:5
Enter Name: Peter
Enter percentage: 76
Student Information List
Roll Number: 1 Name: John percentage:78.000000
Roll Number: 2 Name: Nick percentage:84.000000
Roll Number: 3 Name: Jenny percentage:56.000000
Roll Number: 4 Name: Jack percentage:77.000000
Roll Number: 5 Name: Peter percentage:76.000000
Crea un array di struct
usando la funzione malloc()
in C
C’è un altro modo per creare un array di struct
in C. La memoria può essere allocata usando la funzione malloc()
per un array di struct
. Questa è chiamata allocazione dinamica della memoria.
La funzione malloc()
(allocazione della memoria) viene utilizzata per allocare dinamicamente un singolo blocco di memoria con la dimensione specificata. Questa funzione restituisce un puntatore di tipo void
.
Il puntatore restituito può essere lanciato in un puntatore di qualsiasi forma. Inizializza ogni blocco con il valore di spazzatura predefinito.
La sintassi della funzione malloc()
è la seguente:
ptrVariable = (cast - type*)malloc(byte - size)
Il programma completo per creare dinamicamente un array di struct è il seguente.
#include <stdio.h>
int main(int argc, char** argv) {
typedef struct {
char* firstName;
char* lastName;
int rollNumber;
} STUDENT;
int numStudents = 2;
int x;
STUDENT* students = malloc(numStudents * sizeof *students);
for (x = 0; x < numStudents; x++) {
students[x].firstName = (char*)malloc(sizeof(char*));
printf("Enter first name :");
scanf("%s", students[x].firstName);
students[x].lastName = (char*)malloc(sizeof(char*));
printf("Enter last name :");
scanf("%s", students[x].lastName);
printf("Enter roll number :");
scanf("%d", &students[x].rollNumber);
}
for (x = 0; x < numStudents; x++)
printf("First Name: %s, Last Name: %s, Roll number: %d\n",
students[x].firstName, students[x].lastName, students[x].rollNumber);
return (0);
}
Produzione:
Enter first name:John
Enter last name: Williams
Enter roll number:1
Enter first name:Jenny
Enter last name: Thomas
Enter roll number:2
First Name: John Last Name: Williams Roll Number:1
First Name: Jenny Last Name: Thomas Roll Number:2
Articolo correlato - C Array
- Allocare dinamicamente un array in C
- Array di stringhe in C
- Cancella array di caratteri in C
- Copia array di caratteri in C
- Inizializza l'array di caratteri in C