Funzione Pandas DataFrame DataFrame.to_excel()
-
Sintassi di
pandas.DataFrame.to_excel()
-
Codici di esempio: Pandas
DataFrame.to_excel()
-
Codici di esempio: Pandas
DataFrame.to_excel()
conExcelWriter
-
Codici di esempio: Pandas
DataFrame.to_excel
da aggiungere a un file Excel esistente -
Codici di esempio: Pandas
DataFrame.to_excel
per scrivere più fogli -
Codici di esempio: Pandas
DataFrame.to_excel
con parametroheader
-
Codici di esempio: Pandas
DataFrame.to_excel
conindex=False
-
Codici di esempio: Pandas
DataFrame.to_excel
con parametroindex_label
-
Codici di esempio: Pandas
DataFrame.to_excel
con parametrofloat_format
-
Codici di esempio: Pandas
DataFrame.to_excel
con parametrofreeze_panes
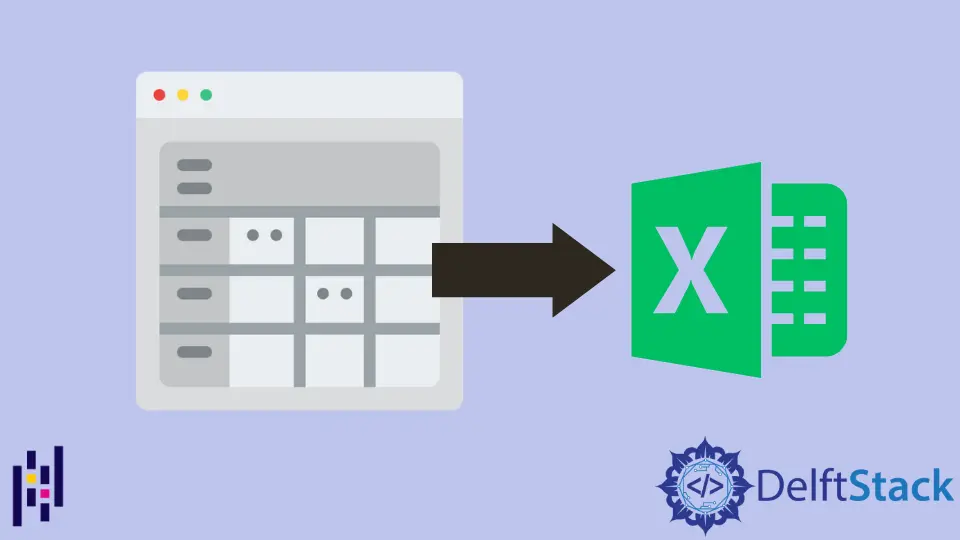
La funzione Python Pandas DataFrame.to_excel(value)
scarica i dati del dataframe in un file Excel, in uno o più fogli.
Sintassi di pandas.DataFrame.to_excel()
DataFrame.to_excel(
excel_writer,
sheet_name="Sheet1",
na_rep="",
float_format=None,
columns=None,
header=True,
index=True,
index_label=None,
startrow=0,
startcol=0,
engine=None,
merge_cells=True,
encoding=None,
inf_rep="inf",
verbose=True,
freeze_panes=None,
)
Parametri
excel_writer |
Percorso del file Excel o l’esistente pandas.ExcelWriter |
sheet_name |
Nome del foglio in cui viene eseguito il dump del dataframe |
na_rep |
Rappresentazione di valori nulli. |
float_format |
Formato dei numeri mobili |
header |
Specifica l’intestazione del file Excel generato. |
index |
Se True , scrivi il dataframe index in Excel. |
index_label |
Etichetta della colonna per la colonna dell’indice. |
startrow |
La riga della cella in alto a sinistra per scrivere i dati in Excel. L’impostazione predefinita è 0 |
startcol |
La colonna della cella in alto a sinistra per scrivere i dati in Excel. L’impostazione predefinita è 0 |
engine |
Parametro facoltativo per specificare il motore da utilizzare. openyxl o xlswriter |
merge_cells |
Unisci MultiIndex alle celle unite |
encoding |
Codifica del file Excel di output. Necessario solo se viene utilizzato il writer xlwt , altri writer supportano Unicode in modo nativo. |
inf_rep |
Rappresentazione dell’infinito. L’impostazione predefinita è inf |
verbose |
Se True , i log degli errori contengono più informazioni |
freeze_panes |
Specificare il più in basso e il più a destra del riquadro bloccato. È a base uno, ma non a base zero. |
Ritorno
None
.
Codici di esempio: Pandas DataFrame.to_excel()
import pandas as pd
dataframe= pd.DataFrame({'Attendance': [60, 100, 80, 78, 95],
'Name': ['Olivia', 'John', 'Laura', 'Ben', 'Kevin'],
'Marks': [90, 75, 82, 64, 45]})
dataframe.to_excel('test.xlsx')
Il chiamante DataFrame
è
Attendance Name Marks
0 60 Olivia 90
1 100 John 75
2 80 Laura 82
3 78 Ben 64
4 95 Kevin 45
Viene creato test.xlsx
.
Codici di esempio: Pandas DataFrame.to_excel()
con ExcelWriter
L’esempio sopra usa il percorso del file come excel_writer
, e potremmo anche usare pandas.Excelwriter
per specificare il file excel che il dataframe scarica.
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": [60, 100, 80, 78, 95],
"Name": ["Olivia", "John", "Laura", "Ben", "Kevin"],
"Marks": [90, 75, 82, 64, 45],
}
)
with pd.ExcelWriter("test.xlsx") as writer:
dataframe.to_excel(writer)
Codici di esempio: Pandas DataFrame.to_excel
da aggiungere a un file Excel esistente
import pandas as pd
import openpyxl
dataframe = pd.DataFrame(
{
"Attendance": [60, 100, 80, 78, 95],
"Name": ["Olivia", "John", "Laura", "Ben", "Kevin"],
"Marks": [90, 75, 82, 64, 45],
}
)
with pd.ExcelWriter("test.xlsx", mode="a", engine="openpyxl") as writer:
dataframe.to_excel(writer, sheet_name="new")
Dovremmo specificare il motore come openpyxl
ma non predefinito xlsxwriter
; altrimenti, avremo l’errore che xlswriter
non supporta la modalità append
.
ValueError: Append mode is not supported with xlsxwriter!
openpyxl
deve essere installato e importato perché non fa parte di pandas
.
pip install openpyxl
Codici di esempio: Pandas DataFrame.to_excel
per scrivere più fogli
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": [60, 100, 80, 78, 95],
"Name": ["Olivia", "John", "Laura", "Ben", "Kevin"],
"Marks": [90, 75, 82, 64, 45],
}
)
with pd.ExcelWriter("test.xlsx") as writer:
dataframe.to_excel(writer, sheet_name="Sheet1")
dataframe.to_excel(writer, sheet_name="Sheet2")
Esegue il dump dell’oggetto dataframe sia su Sheet1
che su Sheet2
.
Puoi anche scrivere dati diversi su più fogli se specifichi il parametro columns
.
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": [60, 100, 80, 78, 95],
"Name": ["Olivia", "John", "Laura", "Ben", "Kevin"],
"Marks": [90, 75, 82, 64, 45],
}
)
with pd.ExcelWriter("test.xlsx") as writer:
dataframe.to_excel(writer, columns=["Name", "Attendance"], sheet_name="Sheet1")
dataframe.to_excel(writer, columns=["Name", "Marks"], sheet_name="Sheet2")
Codici di esempio: Pandas DataFrame.to_excel
con parametro header
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": [60, 100, 80, 78, 95],
"Name": ["Olivia", "John", "Laura", "Ben", "Kevin"],
"Marks": [90, 75, 82, 64, 45],
}
)
with pd.ExcelWriter("test.xlsx") as writer:
dataframe.to_excel(writer, header=["Student", "First Name", "Score"])
L’intestazione predefinita nel file Excel creato è la stessa dei nomi delle colonne del dataframe. Il parametro header
specifica la nuova intestazione per sostituire quella predefinita.
Codici di esempio: Pandas DataFrame.to_excel
con index=False
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": [60, 100, 80, 78, 95],
"Name": ["Olivia", "John", "Laura", "Ben", "Kevin"],
"Marks": [90, 75, 82, 64, 45],
}
)
with pd.ExcelWriter("test.xlsx") as writer:
dataframe.to_excel(writer, index=False)
index = False
specifica che DataFrame.to_excel()
genera un file Excel senza riga di intestazione.
Codici di esempio: Pandas DataFrame.to_excel
con parametro index_label
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": [60, 100, 80, 78, 95],
"Name": ["Olivia", "John", "Laura", "Ben", "Kevin"],
"Marks": [90, 75, 82, 64, 45],
}
)
with pd.ExcelWriter("test.xlsx") as writer:
dataframe.to_excel(writer, index_label="id")
index_label='id'
imposta il nome della colonna della colonna indice come id
.
Codici di esempio: Pandas DataFrame.to_excel
con parametro float_format
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": [60, 100, 80, 78, 95],
"Name": ["Olivia", "John", "Laura", "Ben", "Kevin"],
"Marks": [90, 75, 82, 64, 45],
}
)
with pd.ExcelWriter("test.xlsx") as writer:
dataframe.to_excel(writer, float_format="%.1f")
float_format="%.1f"
specifica che il numero in virgola mobile deve avere due cifre in virgola mobile.
Codici di esempio: Pandas DataFrame.to_excel
con parametro freeze_panes
import pandas as pd
dataframe = pd.DataFrame(
{
"Attendance": [60, 100, 80, 78, 95],
"Name": ["Olivia", "John", "Laura", "Ben", "Kevin"],
"Marks": [90, 75, 82, 64, 45],
}
)
with pd.ExcelWriter("test.xlsx") as writer:
dataframe.to_excel(writer, freeze_panes=(1, 1))
freeze_panes=(1,1)
specifica che il file excel ha la prima riga bloccata e la prima colonna bloccata.
Founder of DelftStack.com. Jinku has worked in the robotics and automotive industries for over 8 years. He sharpened his coding skills when he needed to do the automatic testing, data collection from remote servers and report creation from the endurance test. He is from an electrical/electronics engineering background but has expanded his interest to embedded electronics, embedded programming and front-/back-end programming.
LinkedIn Facebook